flutter_quill 4.1.9
flutter_quill: ^4.1.9 copied to clipboard
A rich text editor supporting mobile and web (Demo App @ bulletjournal.us)
A rich text editor for Flutter
FlutterQuill is a rich text editor and a Quill component for Flutter.
This library is a WYSIWYG editor built for the modern mobile platform, with web compatibility under development. Check out our Youtube Playlist to take a detailed walkthrough of the code base. You can join our Slack Group for discussion.
Demo App: https://bulletjournal.us/home/index.html
Pub: https://pub.dev/packages/flutter_quill
Usage #
See the example
directory for a minimal example of how to use FlutterQuill. You typically just need to instantiate a controller:
QuillController _controller = QuillController.basic();
and then embed the toolbar and the editor, within your app. For example:
Column(
children: [
QuillToolbar.basic(controller: _controller),
Expanded(
child: Container(
child: QuillEditor.basic(
controller: _controller,
readOnly: false, // true for view only mode
),
),
)
],
)
Check out Sample Page for advanced usage.
Input / Output #
This library uses Quill as an internal data format.
- Use
_controller.document.toDelta()
to extract the deltas. - Use
_controller.document.toPlainText()
to extract plain text.
FlutterQuill provides some JSON serialisation support, so that you can save and open documents. To save a document as JSON, do something like the following:
var json = jsonEncode(_controller.document.toDelta().toJson());
You can then write this to storage.
To open a FlutterQuill editor with an existing JSON representation that you've previously stored, you can do something like this:
var myJSON = jsonDecode(incomingJSONText);
_controller = QuillController(
document: Document.fromJson(myJSON),
selection: TextSelection.collapsed(offset: 0));
Configuration #
The QuillToolbar
class lets you customise which formatting options are available.
Sample Page provides sample code for advanced usage and configuration.
Font Size
Within the editor toolbar, a drop-down with font-sizing capabilties is available. This can be enabled or disabled with showFontSize
.
When enabled, the default font-size values can be modified via optional fontSizeValues
. fontSizeValues
accepts a Map<String, int>
consisting of a String
title for the font size and an int
value for the font size. Example:
fontSizeValues: const {'Small':8, 'Medium':24, 'Large':46}
If a font size of 0
is used, then font sizing is removed from the given block of text.
fontSizeValues: const {'Default':0, 'Small':8, 'Medium':24, 'Large':46}
The initial value for font-size in the editor can also be defined via optional initialFontSizeValue
which corresponds to the index value within your map. For example, if you would like the Medium font size to be the default start size in the above example, you would set initialFontSizeValue: 2
.
This initial font size is not applied to the delta when first building the widget, it is only used to change the initial value for the drop-down widget.
Web #
For web development, use flutter config --enable-web
for flutter or use ReactQuill for React.
It is required to provide EmbedBuilder
, e.g. defaultEmbedBuilderWeb.
Also it is required to provide webImagePickImpl
, e.g. Sample Page.
Desktop #
It is required to provide filePickImpl
for toolbar image button, e.g. Sample Page.
Custom Size Image for Mobile #
Define mobileWidth
, mobileHeight
, mobileMargin
, mobileAlignment
as follows:
{
"insert": {
"image": "https://user-images.githubusercontent.com/122956/72955931-ccc07900-3d52-11ea-89b1-d468a6e2aa2b.png"
},
"attributes":{
"style":"mobileWidth: 50; mobileHeight: 50; mobileMargin: 10; mobileAlignment: topLeft"
}
}
Translation #
The package offers translations for the quill toolbar and editor, it will follow the system locale unless you set your own locale with:
QuillToolbar(locale: Locale('fr'), ...)
QuillEditor(locale: Locale('fr'), ...)
Currently, translations are available for these 19 locales:
Locale('en')
Locale('ar')
Locale('de')
Locale('da')
Locale('fr')
Locale('zh', 'CN')
Locale('ko')
Locale('ru')
Locale('es')
Locale('tr')
Locale('uk')
Locale('ur')
Locale('pt')
Locale('pl')
Locale('vi')
Locale('id')
Locale('no')
Locale('fa')
Locale('hi')
Contributing to translations #
The translation file is located at lib/src/translations/toolbar.i18n.dart. Feel free to contribute your own translations, just copy the English translations map and replace the values with your translations. Then open a pull request so everyone can benefit from your translations!
Sponsors #
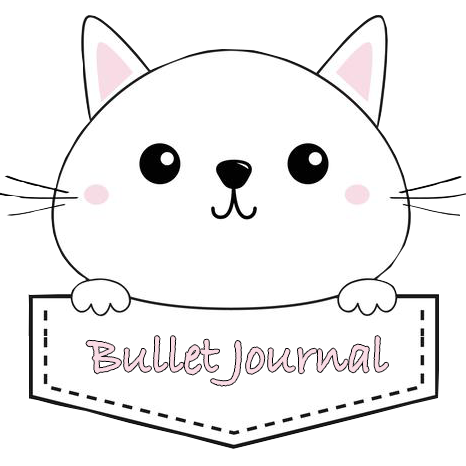