versionarte 1.2.0
versionarte: ^1.2.0 copied to clipboard
Force update, show update indicator and disable the app for maintenance with total freedom over the UI.
versionarte #
Force update, show update indicator and disable the app for maintenance with total freedom over the UI.
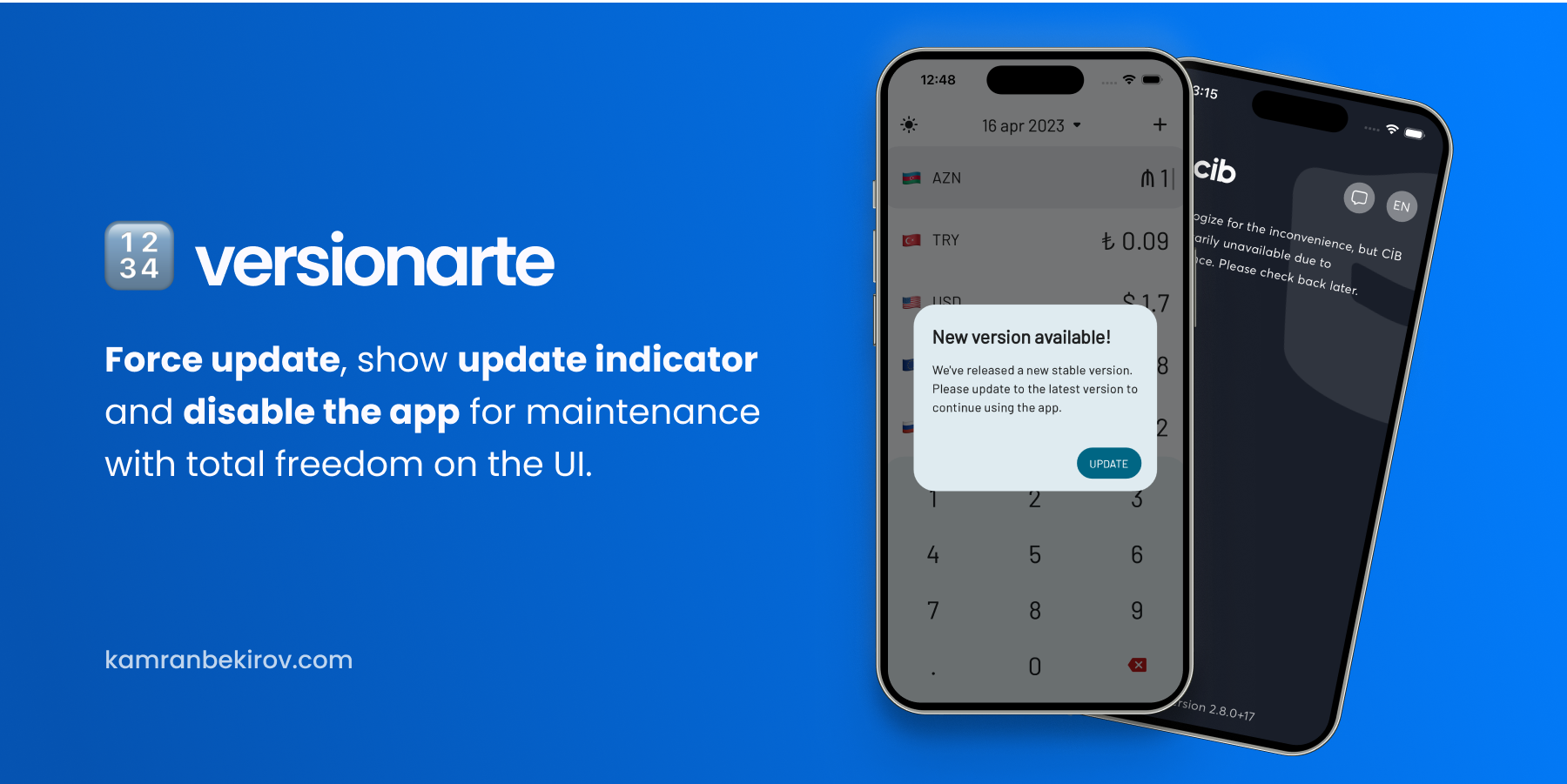
Features can be implemented with versionarte:
- β Force users to update to the latest version
- ππ»ββοΈ Have separate values for each platform
- π§ Disable app for maintenance with custom informative text
- π Inform users about an optional update availability
- π Launch the App Store on iOS and Play Store on Android
π Getting Started #
Add the package to your pubspec.yaml
file:
dependencies:
versionarte: <latest_version>
Import the package in your Dart code:
import 'package:versionarte/versionarte.dart';
π‘ Obtain the status #
Call Versionarte.check
method by providing it a VersionarteProvider
(an object responsible for fetching the versioning information from a remote service) to get a VersionarteResult
(an object containing app's versioning and availability information).
There are 2 built-in providers, RemoteConfigVersionarteProvider and RestfulVersionarteProvider, which fetches the versioning information from Firebase Remote Config and RESTful API respectively. You can also create your own custom provider by extending the VersionarteProvider class.
1. Using Firebase Remote Config #
The RemoteConfigVersionarteProvider
fetches information stored in Firebase Remote Config with the key name of "versionarte". You need to set up the Firebase Remote Config service before using this provider. See Firebase Remote Config setup guide to learn more about configuration.
Example:
final result = await Versionarte.check(
versionarteProvider: RemoteConfigVersionarteProvider(),
);
Optional parameters:
keyName
: key name for the Firebase Remote Config to fetch. By default, it's set to "versionarte". Specify if you upload the Configuration JSON using a different key name.initializeInternally
: if your project already initializes and configures Firebase Remote Config, set this tofalse
. By default, it's set totrue
.remoteConfigSettings
: settings for Firebase Remote Config ifinitializeInternally
set to true. By default,fetchTimeout
andminimumFetchInterval
are set to10 seconds
.
2. Using RESTful API #
The RestfulVersionarteProvider
fetches versioning and availability information by sending HTTP GET request to the specified URL with optional headers. The response body should be a JSON string that follows the Configuration JSON format.
Example:
final result = await Versionarte.check(
versionarteProvider: RestfulVersionarteProvider(
url: 'https://myapi.com/getVersioning',
),
);
Optional parameters:
headers
: headers to send with the HTTP GET request. By default, it's set to an empty map.
3. Using custom VersionarteProvider #
To use remote services to provide versioning and availability information of your app, extend the VersionarteProvider
class and override the getStoreVersioning
method which is responsible for fetching the information and returning it as a StoreVersioning
object.
class MyCustomVersionarteProvider extends VersionarteProvider {
@override
Future<StoreVersioning> getStoreVersioning() async {
final result = MyCustomService.fetchVersioning();
final decodedResult = jsonDecode(result);
return StoreVersioning.fromJson(decodedResult);
}
Example:
final result = await Versionarte.check(
versionarteProvider: MyCustomVersionarteProvider(),
);
π― Handle the status #
Obtained VersionarteResult
has 3 parameters:
status
: (VersionarteResult) the status of the app. It can be one of the following values:VersionarteStatus.inactive
: the app is inactive for usage.VersionarteStatus.forcedUpdate
: user must update before continuing.VersionarteStatus.outdated
: user can continue with and without updating.VersionarteStatus.upToDate
: the user's version is up to date.VersionarteStatus.unknown
: error occured while checking status.
details
: (StorePlatformDetails) Details for the current platform, including messages for when the app is inactive.
Then, based on status
do the if-else checks:
if (result.status == VersionarteResult.inactive) {
final message = result.details.status.getMessageForLanguage('en');
// TODO: Handle the case where the app is inactive
} else if (result == VersionarteResult.forcedUpdate) {
// TODO: Handle the case where an update is required
} else if (result == VersionarteResult.upToDate) {
// TODO: Handle the case where an update is optional
}
π Launching the download stores #
To launch download page of the app use Versionarte.launchDownloadUrl
:
final Map<TargetPlatform, String?> downloadUrls = result.storeVersioning!.downloadUrls;
await Versionarte.launchDownloadUrl(downloadUrls);
π‘ Don't forget to add "download_url" property to each platform that you support on Configuration JSON π‘ Launching store won't work on iOS simulator due to its limitations.
See the example directory for a complete sample app.
ποΈ Configuration JSON #
For providing app's status and availability information, versionarte requires a specific JSON format. Whether you're using RemoteConfigVersionarteProvider
, RestfulVersionarteProvider
, or a custom VersionarteProvider
, make sure to use this JSON format.
π‘ Information for all platforms in the JSON is not necessary: you can provide information for only one platform, or for two platforms, or for all three platforms.
π‘ While the app status is active, the message
can be left empty or set to null
.
{
"android": {
"version": {
"minimum": "2.7.0",
"latest": "2.8.0"
},
"download_url": "https://play.google.com/store/apps/details?id=app.librokit",
"status": {
"active": true,
"message": {
"en": "App is in maintanence mode, please come back later.",
"es": "La aplicaciΓ³n estΓ‘ en modo de mantenimiento, vuelva mΓ‘s tarde."
}
}
},
"iOS": {
// Same stucture as above
}
"macOS": {
// Same stucture as above
},
"windows": {
// Same stucture as above
},
"linux": {
// Same stucture as above
}
}
This JSON represents information stored separately for three platforms, containing the minimum and latest versions, and the availability status.
Each platform contains two objects:
version
:minimum
: The minimum version of the app users can use.latest
: The latest version of the app available.download_url
: The URL to download the app from the store.
status
:active
: A boolean that indicates whether the app is currently active or not.message
: A Map that contains the messages for different languages to be displayed to the user when app is inactive. The keys of the map represent the language codes (e.g., "en" for English, "es" for Spanish), and the values represent the message in that language.
π Faced issues? #
If you encounter any problems or you feel the library is missing a feature, please raise a ticket on GitHub and I'll look into it.