infinite_scroll_pagination 5.0.0
infinite_scroll_pagination: ^5.0.0 copied to clipboard
Lazily load and display pages of items as the user scrolls down your screen.
Chosen as a Flutter Favorite by the Flutter Ecosystem Committee
Infinite Scroll Pagination #
Unopinionated, extensible and highly customizable package to help you lazily load and display small chunks of items as the user scrolls down the screen – known as infinite scrolling pagination, endless scrolling pagination, auto-pagination, lazy loading pagination, progressive loading pagination, etc.
Designed to feel like part of the Flutter framework.
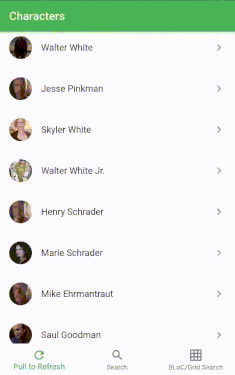
Tutorial #
By raywenderlich.com (step-by-step, hands-on, in-depth, and illustrated).
Usage #
class ListViewScreen extends StatefulWidget {
const ListViewScreen({super.key});
@override
State<ListViewScreen> createState() => _ListViewScreenState();
}
class _ListViewScreenState extends State<ListViewScreen> {
late final _pagingController = PagingController<int, Photo>(
getNextPageKey: (state) => (state.keys?.last ?? 0) + 1,
fetchPage: (pageKey) => RemoteApi.getPhotos(pageKey),
);
@override
void dispose() {
_pagingController.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) => PagingListener(
controller: _pagingController,
builder: (context, state, fetchNextPage) => PagedListView<int, Photo>(
state: state,
fetchNextPage: fetchNextPage,
builderDelegate: PagedChildBuilderDelegate(
itemBuilder: (context, item, index) => ImageListTile(item: item),
),
),
);
}
For more usage examples, please take a look at our cookbook or check out the example project.
Features #
-
Architecture-agnostic: Works with any state management approach, from setState to BLoC. Not even Future usage is assumed.
-
Layout-agnostic: Out-of-the-box widgets corresponding to GridView, SliverGrid, ListView and SliverList – including
.separated
constructors. Not enough? You can easily create a custom layout. -
API-agnostic: By letting you in complete charge of your API calls, Infinite Scroll Pagination works with any pagination strategy.
-
Highly customizable: You can change everything. Provide your own progress, error and empty list indicators. Too lazy to change? The defaults will cover you.
-
Extensible: Seamless integration with pull-to-refresh, searching, filtering and sorting.
-
Listen to state changes: In addition to displaying widgets to inform the current status, such as progress and error indicators, you can also use a listener to display dialogs/snackbars/toasts or execute any other action.
Migration #
if you are upgrading the package, please check the migration guide for instructions on how to update your code.
API Overview #