dart_cmder 0.0.6-prerelease
dart_cmder: ^0.0.6-prerelease copied to clipboard
This Dart package offers a streamlined and intuitive interface for building powerful Command Line Interface (CLI) applications.
dart_cmder #
This Dart package offers a streamlined and intuitive interface for building powerful Command Line Interface (CLI) applications.
It comes bundled with an integrated logger (Trace), providing comprehensive visibility into the execution flow of your CLI apps. Additionally, the package provides a set of convenient out-of-the-box arguments, including the project root path, log level, and an optional log files directory to save your log files. These pre-defined arguments simplify the process of configuring and customizing your CLI applications.
With this package, developers can effortlessly handle input, output, and essential configuration options, allowing them to focus on the core logic of their applications. Whether you're crafting a simple script or a complex command-line tool, this package empowers you to deliver seamless user experiences.
Explore the documentation, check the examples and unleash the full potential of your CLI apps with dart_cmder.
Table of Contents #
- Usage
- Interface
- Tools
- Usage
- Contribution
- Changelog
Usage #
- Create a command with custom arguments
class DemoCommand extends BaseCommand {
DemoCommand({
super.arguments = const <BaseArgument<void>>[],
super.subCommands = const <BaseCommand>[],
});
@override
String get name => 'cmd';
@override
String get description => 'This is a demo command';
@override
List<BaseArgument<void>> get arguments => <BaseArgument<void>>[enabledArg, inputArg, modeArg];
static const FlagArgument enabledArg = FlagArgument(
name: 'enabled',
abbr: 'e',
help: 'This is a demo flag argument',
);
static const OptionArgument<String> inputArg = OptionArgument<String>(
name: 'input',
abbr: 'i',
help: 'This is a demo option argument',
defaultsTo: 'default-input-value',
);
static const EnumArgument<Mode> modeArg = EnumArgument<Mode>(
name: 'mode',
abbr: 'm',
help: 'This is a demo enum argument',
defaultsTo: Mode.debug,
);
static const MultiEnumArgument<Feature> featureArg = MultiEnumArgument<Feature>(
name: 'feature',
abbr: 'f',
help: 'This is a demo multi-option argument',
defaultsTo: <Feature>[Feature.feat1],
);
Mode get mode => modeArg.parse(argResults)!;
bool get enabled => enabledArg.parse(argResults);
@override
Future<void> execute() async {
printArguments();
Trace.info('Lorem ipsum dolor sit amet, consectetur adipiscing elit, \n'
'sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.');
}
}
- Create a runner
class DemoRunner extends BaseRunner {
DemoRunner({
final List<BaseCommand> commands = const <BaseCommand>[],
}) : super(
executableName: 'demo',
description: 'This is a demo CLI app written in Dart using dart_cmder.',
$commands: <BaseCommand>[
DemoCommand(),
...commands,
],
);
}
- Run your CLI app
import 'dart:io';
import 'package:trace/trace.dart';
import 'demo_runner.dart';
void main() {
final List<String> args = <String>[
'cmd',
'--no-enabled',
'-m',
'option3',
'-i',
'Hello dart_cmder',
'-l',
LogLevel.verbose.name,
'-d',
'${Directory.current.path}/logs',
];
DemoRunner().run(args);
}
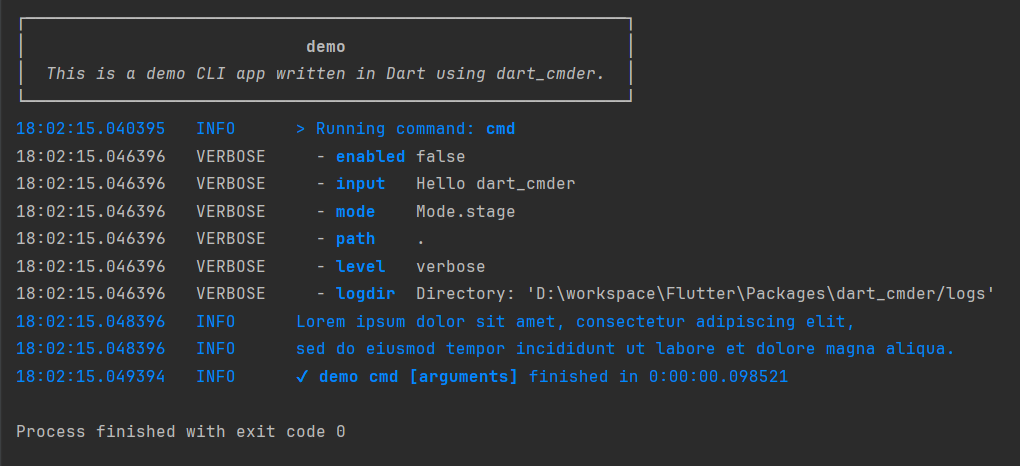
Check the example folder for the complete CLI app samples.
Contribution #
Check the contribution guide if you want to help with dart_cmder.
Changelog #
Check the changelog to learn what's new in dart_cmder.