ansix 0.3.4
ansix: ^0.3.4 copied to clipboard
AnsiX is a powerful and easy-to-use library that provides tools and extensions for adding ANSI color and styling support to your Dart & Flutter applications.
AnsiX #
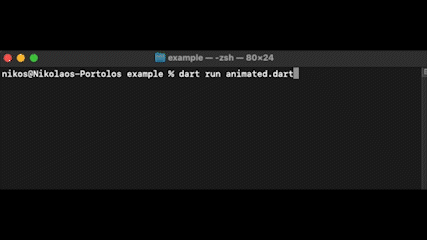
AnsiX is a powerful and easy-to-use library that provides tools and extensions for adding ANSI color and styling support to your Dart & Flutter applications.
You can easily create colorful and visually appealing output for your command-line interfaces, including bold, italic, underline, and strikethrough text, as well as foreground and background colors in a wide range of ANSI-compatible terminals.
Whether you're building a CLI tool, a dart server, or a Flutter application, AnsiX makes it easy to add ANSI styling to your output with minimal effort and maximum flexibility.
Table of contents #
- Introduction
- AnsiX Features
- Examples
- FAQ
- Contribution
- Changelog
Introduction #
ANSI escape codes, also known as ANSI color codes or ANSI escape sequences, are a set of standardized sequences of characters used in computing to control text formatting, color, and other visual aspects of terminal output.
The ANSI escape codes consist of a special sequence of characters that begins with the escape character (ESC, ASCII code 27) followed by one or more control characters. These control characters can include commands to change the color or background of text, move the cursor to a specific location on the screen, erase part of the screen, and more.
For example, the ANSI escape code "\e[31m"
changes the text color to red, while "\e[42m"
changes the background color to green.
The code "\e[2J"
clears the entire screen, and "\e[;H"
moves the cursor to the top-left corner of the screen.
ANSI escape codes are widely used in command-line interfaces, terminal emulators, and other text-based applications to provide a richer and more interactive user experience.
Examples #
import 'package:ansix/ansix.dart';
void main() {
// Ensure that the attached terminal supports ANSI formatting
AnsiX.ensureSupportsAnsi();
// String extensions
print('This is a bold text'.bold());
print('This is a text with red foreground color'.red());
final StringBuffer buffer = StringBuffer()
..writeWithForegroundColor('Hello ', AnsiColor.blue)
..writeStyled(
'AnsiX ',
textStyle: const AnsiTextStyle(bold: true),
foregroundColor: AnsiColor.aquamarine2,
)
..writeWithForegroundColor('!', AnsiColor.red)
..writeWithForegroundColor('!', AnsiColor.green)
..writeWithForegroundColor('!', AnsiColor.blue);
// StringBuffer extensions
print(buffer);
}
- Print data grid
import 'package:ansix/ansix.dart';
void main() {
// Ensure that the attached terminal supports ANSI formatting
AnsiX.ensureSupportsAnsi();
final List<List<Object?>> rows = <List<Object?>>[
<Object?>['#', 'Title', 'Release Year', 'IMDb Rate'],
...movies.mapIndexed((int i, Movie m) {
return <Object>[i, m.title, m.releaseYear, m.rate];
}).toList(growable: false),
<Object?>['Average', '', '', movies.map((Movie m) => m.rate).toList(growable: false).average],
];
final AnsiGrid verticalGrid = AnsiGrid.fromRows(rows, theme: verticalTheme);
print(verticalGrid);
}
- Vertical grid
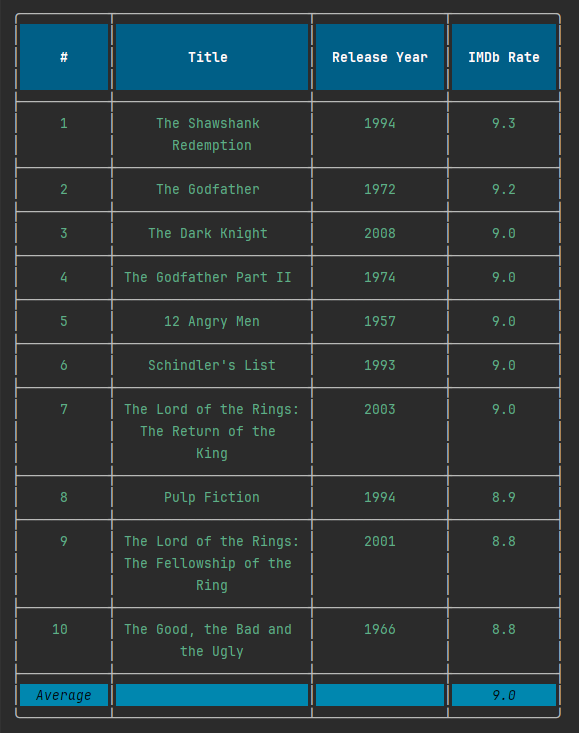
- Horizontal grid
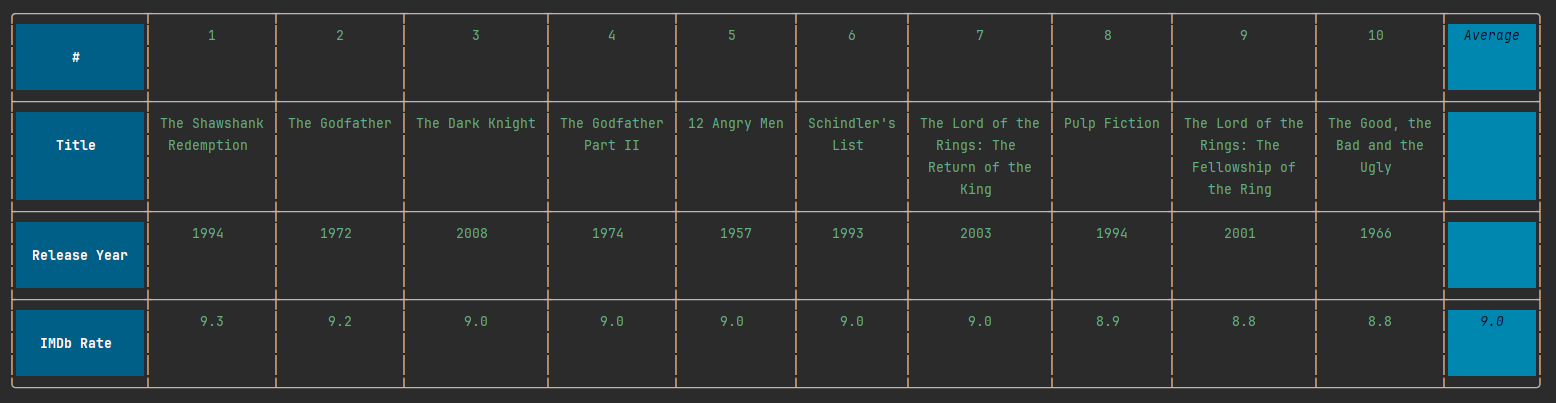
You can also check the example folder for more samples.
- Print tree view
import 'package:ansix/ansix.dart';
void main() {
// Ensure that the attached terminal supports ANSI formatting
AnsiX.ensureSupportsAnsi();
final User user = User(
id: '123456789',
name: 'John Doe',
phone: '555-1234',
email: 'john.doe@email.com',
);
AnsiX.printTreeView(
user,
theme: AnsiTreeViewTheme.$default(),
);
}
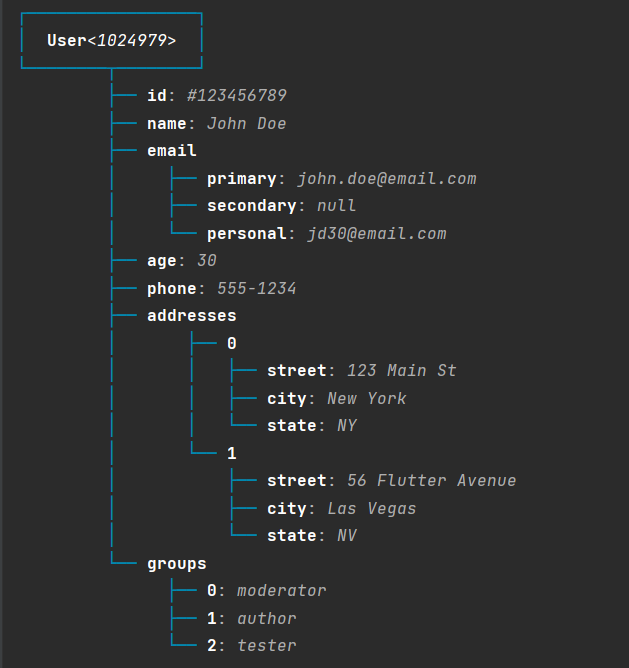
- Print json/map
import 'package:ansix/ansix.dart';
void main() {
// Ensure that the attached terminal supports ANSI formatting
AnsiX.ensureSupportsAnsi();
final Map<String, dynamic> json = <String, dynamic>{
'field1': 'value',
'field2': 3.0,
'field3': true,
};
print('Json'.underline().colored(background: AnsiColor.darkSeaGreen, foreground: AnsiColor.black));
AnsiX.printJson(json, foreground: AnsiColor.cadetBlue);
}
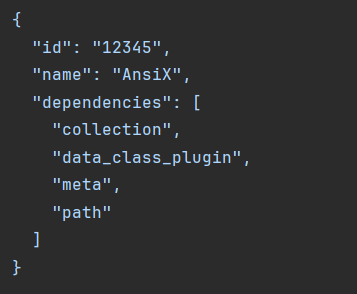
FAQ #
If you have questions about AnsiX, make sure to check the FAQ document.
Contribution #
Check the contribution guide if you want to help with AnsiX.
Changelog #
Check the changelog to learn what's new in AnsiX.