result_dart 1.1.1
result_dart: ^1.1.1 copied to clipboard
Result for dart. It is an implementation based on Kotlin Result and Swift Result.
RESULT_DART
This package aims to create an implemetation of Kotlin's and Swift's Result class and own operators.
Inspired by Higor Lapa's multiple_result package, the `dartz` package and the `fpdart` package.
Explore the docs Β»
Report Bug
Β·
Request Feature
Table of Contents
About The Project #
Overruns are common in design, and modern architectures always designate a place to handle failures.
This means dramatically decreasing try/catch usage and keeping treatments in one place.
But the other layers of code need to know about the two main values [Success, Failure]
. The solution lies in the
Result
class pattern implemented in Kotlin
and Swift
and now also in Dart
via this package(result_dart
).
This project is distributed under the MIT License. See LICENSE
for more information.
Sponsors #
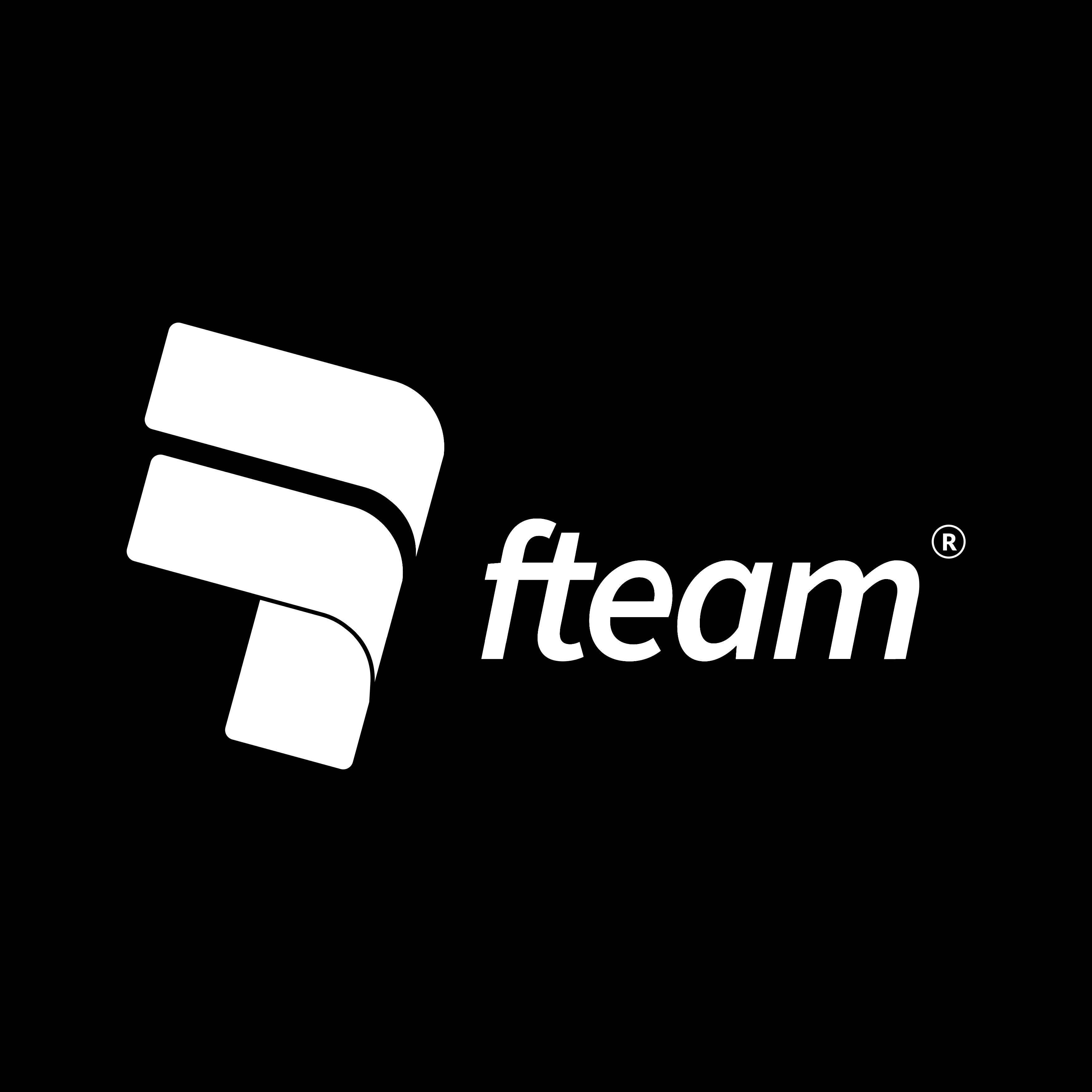
Getting Started #
To get result_dart working in your project follow either of the instructions below:
a) Add result_dart as a dependency in your Pubspec.yaml:
dependencies:
result_dart: x.x.x
b) Use Dart Pub:
dart pub add result_dart
How to Use #
In the return of a function that you want to receive an answer as Sucess or Failure, set it to return a Result type;
Result getSomethingPretty();
then add the Success and the Failure types.
Result<String, Exception> getSomethingPretty() {
}
In the return of the above function, you just need to use:
// Using Normal instance
return Success('Something Pretty');
// Using Result factory
return Result.success('Something Pretty');
// import 'package:result_dart/functions.dart'
return successOf('Something Pretty');
// Using extensions
return 'Something Pretty'.toSuccess();
or
// Using Normal instance
return Failure(Exception('something ugly happened...'));
// Using Result factory
return Result.failure('something ugly happened...');
// import 'package:result_dart/functions.dart'
return failureOf('Something Pretty');
// Using extensions
return 'something ugly happened...'.toFailure();
The function should look something like this:
Result<String, Exception> getSomethingPretty() {
if(isOk) {
return Success('OK!');
} else {
return Failure(Exception('Not Ok!'));
}
}
or when using extensions, like this:
Result<String, Exception> getSomethingPretty() {
if(isOk) {
return 'OK!'.toSuccess();
} else {
return Exception('Not Ok!').toFailure();
}
}
IMPORTANT NOTE: The
toSuccess()
andtoFailure()
methods cannot be used on aResult
object or aFuture
. If you try, will be throw a Assertion exception.
Handling the Result with fold
:
Returns the result of onSuccess for the encapsulated value
if this instance represents Success
or the result of onError function
for the encapsulated value if it is Failure
.
void main() {
final result = getSomethingPretty();
final String message = result.fold(
(success) {
// handle the success here
return "success";
},
(failure) {
// handle the failure here
return "failure";
},
);
}
Handling the Result with getOrThrow
Returns the success value as a throwing expression.
void main() {
final result = getSomethingPretty();
try {
final value = result.getOrThrow();
} on Exception catch(e){
// e
}
}
Handling the Result with getOrNull
Returns the value of [Success] or null.
void main() {
final result = getSomethingPretty();
result.getOrNull();
}
Handling the Result with getOrElse
Returns the encapsulated value if this instance represents Success
or the result of onFailure
function for
the encapsulated a Failure
value.
void main() {
final result = getSomethingPretty();
result.getOrElse((failure) => 'OK');
}
Handling the Result with getOrDefault
Returns the encapsulated value if this instance represents
Success
or the defaultValue
if it is Failure
.
void main() {
final result = getSomethingPretty();
result.getOrDefault('OK');
}
Handling the Result with exceptionOrNull
Returns the value of [Failure] or null.
void main() {
final result = getSomethingPretty();
result.exceptionOrNull();
}
Transforming a Result #
Mapping success value with map
Returns a new Result
, mapping any Success
value
using the given transformation.
void main() {
final result = getResult()
.map((e) => MyObject.fromMap(e));
result.getOrNull(); //Instance of 'MyObject'
}
Mapping failure value with mapError
Returns a new Result
, mapping any Error
value
using the given transformation.
void main() {
final result = getResult()
.mapError((e) => MyException(e));
result.exceptionOrNull(); //Instance of 'MyException'
}
Chain others [Result] by any Success
value with flatMap
Returns a new Result
, mapping any Success
value
using the given transformation and unwrapping the produced Result
.
Result<String, MyException> checkIsEven(String input){
if(input % 2 == 0){
return Success(input);
} else {
return Failure(MyException('isn`t even!'));
}
}
void main() {
final result = getNumberResult()
.flatMap((s) => checkIsEven(s));
}
Chain others [Result] by Failure
value with flatMapError
Returns a new Result
, mapping any Error
value
using the given transformation and unwrapping the produced Result
.
void main() {
final result = getNumberResult()
.flatMapError((e) => checkError(e));
}
Resolve [Result] by Failure
value with recover
Returns the encapsulated Result
of the given transform function
applied to the encapsulated a Failure
or the original
encapsulated value if it is success.
void main() {
final result = getNumberResult()
.recover((f) => Success('Resolved!'));
}
Add a pure Success
value with pure
Change the [Success] value.
void main() {
final result = getSomethingPretty().pure(10);
String? mySuccessResult;
if (result.isSuccess()) {
mySuccessResult = result.getOrNull(); // 10
}
}
Add a pure Failure
value with pureError
Change the [Failure] value.
void main() {
final result = getSomethingPretty().pureError(10);
if (result.isFailure()) {
result.exceptionOrNull(); // 10
}
}
Swap a Result
with swap
Swap the values contained inside the [Success] and [Failure] of this [Result].
void main() {
Result<String, int> result =...;
Result<int, String> newResult = result.swap();
}
Unit Type #
Some results do not need a specific return. Use the Unit type to signal an empty return.
Result<Unit, Exception>
Help with functions that return their parameter: #
NOTE: use import 'package:result_dart/functions.dart'
Sometimes it is necessary to return the parameter of the function as in this example:
final result = Success<int, String>(0);
String value = result.when((s) => '$s', (e) => e);
print(string) // "0";
We can use the identity
function or its acronym id
to facilitate the declaration of this type of function that returns its own parameter and does nothing else:
final result = Success<int, String>(0);
// changed `(e) => e` by `id`
String value = result.when((s) => '$s', id);
print(string) // "0";
Use AsyncResult type: #
AsyncResult<S, E>
represents an asynchronous computation.
Use this component when working with asynchronous Result.
AsyncResult has some of the operators of the Result object to perform data transformations (Success or Failure) before executing the Future.
All Result operators is available in AsyncResult
AsyncResult<S, E>
is a typedef of Future<Result<S, E>>
.
AsyncResult<String, Exception> fetchProducts() async {
try {
final response = await dio.get('/products');
final products = ProductModel.fromList(response.data);
return Success(products);
} on DioError catch (e) {
return Failure(ProductException(e.message));
}
}
...
final state = await fetch()
.map((products) => LoadedState(products))
.mapLeft((failure) => ErrorState(failure))
Features #
- β Result implementation.
- β Result`s operators(map, flatMap, mapError, flatMapError, swap, when, fold, getOrNull, exceptionOrNull, isSuccess, isError).
- β AsyncResult implementation.
- β AsyncResult`s operators(map, flatMap, mapError, flatMapError, swap, when, fold, getOrNull, exceptionOrNull, isSuccess, isError).
- β Auxiliar functions (id, identity, success, failure).
- β Unit type.
Contributing #
Contributions are what make the open source community such an amazing place to learn, inspire, and create. Any contributions you make are greatly appreciated.
If you have a suggestion that would make this better, please fork the repo and create a pull request. You can also simply open an issue with the appropriate tag. Don't forget to give the project a star! Thanks again!
- Fork the Project
- Create your Feature Branch (
git checkout -b feature/AmazingFeature
) - Commit your Changes (
git commit -m 'Add some AmazingFeature'
) - Push to the Branch (
git push origin feature/AmazingFeature
) - Open a Pull Request
Remember to include a tag, and to follow Conventional Commits and Semantic Versioning when uploading your commit and/or creating the issue.
Contact #
Flutterando Community
Acknowledgements #
Thank you to all the people who contributed to this project, whithout you this project would not be here today.
Maintaned by #
Built and maintained by Flutterando.