pub_api_client 2.0.4
pub_api_client: ^2.0.4 copied to clipboard
An API Client for Pub to interact with public package information.
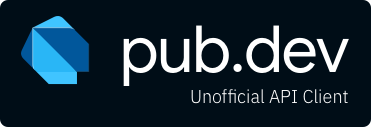
An unofficial API client for Pub.dev #
Aims to be the most complete and stable pub.dev API client. If any particular endpoint is missing please open an issue.
Table of contents #
Usage #
A simple usage example:
import 'package:pub_api_client/pub_api_client.dart';
main() {
final client = PubClient();
}
API #
Packages #
Get Package Info
Retrieves all available information about an specific package.
final package = await client.packageInfo('pkg_name');
Get Package Score
Returns the following score information about a package.
- Pub Points
- Popularity
- Likes
final score = await client.packageScore('pkg_name');
Get Package Metrics
The method 'packageMetrics' returns the package 'score' together with a 'scorecard'
final metrics = await client.packageMetrics('pkg_name');
Get Package Versions
The method 'packageVersions' also returns the versions. However if all you need is versions use this method since it's lighter.
final versions = await client.packageVersions('pkg_name');
Get Package Version Info
The method packageVersionInfo
returns information about a version of a specific package.
final version = await client.packageVersionInfo('pkg_name', 'version');
Get Package Publisher
The method packagePublisher
returns the publisherId of a specific package.
final publisher = await client.packagePublisher('pkg_name');
// publisher.publisherId
Get Package Options
The method packageOptions
returns options of a package.
final options = await client.packageOptions('pkg_name');
Get Documentation
The method documentation
returns all versions and their respective documentation status.
final documentation = await client.documentation('pkg_name');
Like Packages #
All requests for likes require pub.dev authentication.
List liked packages
Displays list of of packages you have liked. Returns list of packageLikes
final likes = await client.listPackageLikes();
Package Like Status
Returns like status of a package.
final like = await client.likePackageStatus();
Like a Package
Likes a package. The method returns packageLike
payload
final like = await client.likePackage('pkg_name');
Unlike a Package
Unlikes a package. The method returns packageLike
payload
final like = await client.unlikePackage('pkg_name');
Search Packages #
Search for packages on pub.dev. Will return the packages that match the query. You can filter the search to a specific publisher
, or packages with a certain dependency
.
final results = await client.search('query', publisher:'publisher_id', dependency:'dependency_name');
// Returns the packages that match the query
print(results.packages)
Paging Search Results
You are able to page search results.
final results = await client.search('query');
final nextResults = await results.nextPage();
print(nextResults.packages)
If you want to retrieve a specific result page you can call the page
parameter directly.
final results = await client.search('query',page:2);
print(results.packages)
Utilities #
Update Notification #
Custom Update Notification
final latest = await client.checkLatest('pkg_name', currentVersion:'current_version');
// Returns the packages that match the query
print(latest.needUpdate) // bool if package needs update
print(latest.packageInfo) // Returns information about the package
print(latest.latestVersion) // Returns the latest version of the package.
This package has a helper method if you want to notify users of your CLI of a newer version. In order to check for update you have to provide currentVersion
.
Check Update Printer #
Snippet for implementation of check update functionality
/// Checks and prints if [currentVersion] of a [package]
/// is not the latest version.
Future<bool> checkUpdatePrinter(
String package, {
required String currentVersion,
}) async {
final latest = await PubClient().checkLatest(
package,
currentVersion: currentVersion,
);
final latestVersion = latest.latestVersion;
if (latest.needUpdate) {
print(
'Update Available for $package: $currentVersion → $latestVersion',
);
print('Changelog: ${latest.packageInfo.changelogUrl}');
}
return latest.needUpdate;
}