notification_builder 0.0.1
notification_builder: ^0.0.1 copied to clipboard
A widget that builds using notifications dispatched via the build context.
notification_builder
#
đĻģ A widget that builds using notifications dispatched via the build context.
Table of contents #
About #
Notifications â is a tool Flutter uses to pass the data higher in the widget tree hierarchy. Somewhere in depth of your widget tree you can fire a notification and it will go up, like a bubble. And on top, you can catch it using a NotificationBuilder
to build your UI.
The problem #
Imagine the following widget tree:
MyWidget(
color: // I want this to be changed once the button below is clicked!
child: const SomeChild(
child: AnotherChild(
// More and more widgets...
child: ChildWithTheButton(),
),
),
);
class ChildWithTheButton extends StatelessWidget {
@override
Widget build(BuildContext context) {
return TextButton(
title: 'Change colour',
onPressed: // Here! I want the colour to be changed on this button pressed!
);
}
}
You could either pass something like a ChangeNotifier<Color>
, or pass a callback function to set the state, or even use an InheritedWidget
. Another option is to use a NotificationListener
.
The solution #
đĄ Click here to check out the full example.
Define your notification:
class ColorNotification extends Notification {
const ColorNotification(this.color);
final Color color;
}
Use a NotificationBuilder to catch notifications:
// MyWidget
NotificationBuilder<ColorNotification>(
builder: (context, notification, child) {
// Note: the notification parameter will be null at the very first build.
// Use a fallback value like this.
final color = notification?.color ?? Colors.white;
return AnimatedContainer(
duration: const Duration(milliseconds: 200),
curve: Curves.fastOutSlowIn,
decoration: BoxDecoration(color: color),
child: child,
);
},
child: const SomeChild(...),
),
Fire notifications from the widget tree below the builder:
onPressed: () {
ColorNotification(color).dispatch(context);
},
Result #
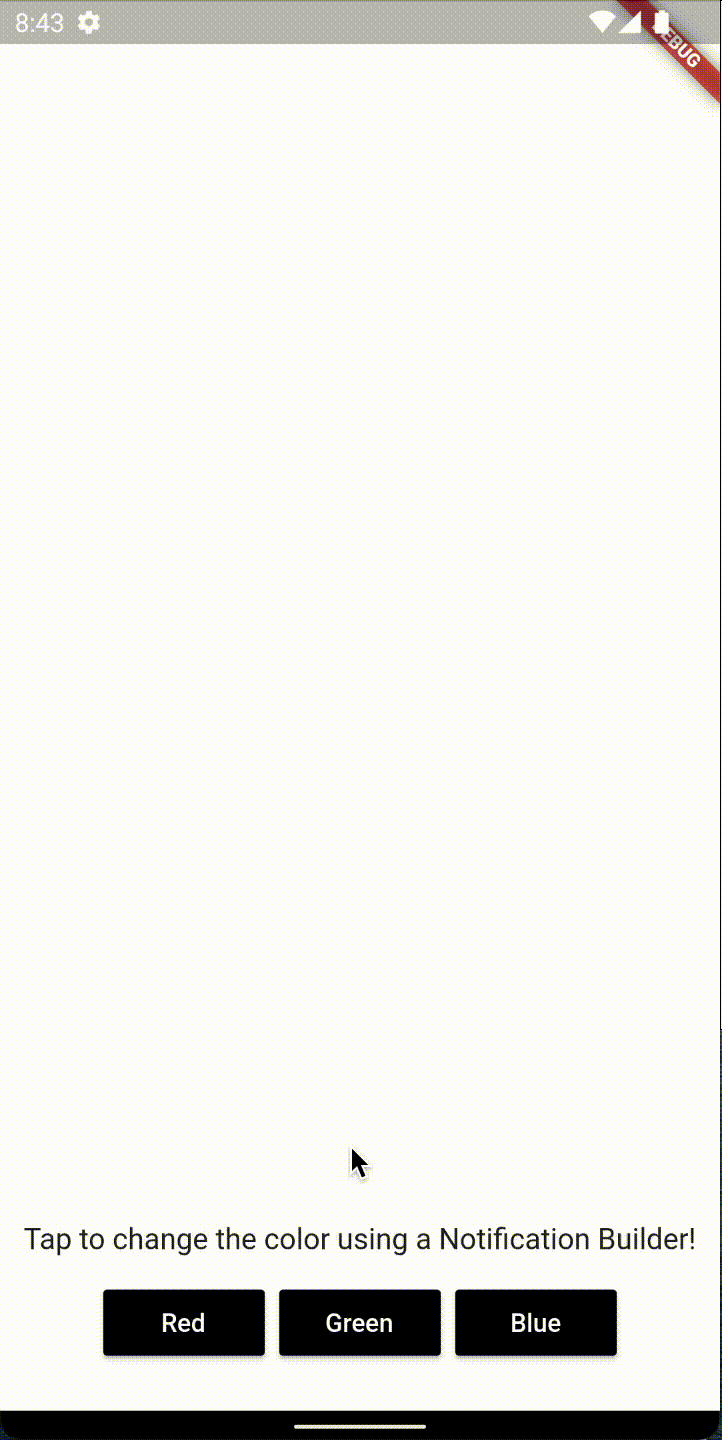
If you don't want certain notification to trigger rebuilds... #
Then you can use the buildWhen
parameter!
buildWhen: (notification) {
// Now if a passed color would be red, the notification will be ignored!
return notification.color != Colors.red,
}
Getting started #
pub #
Add the package to pubspec.yaml
:
dependencies:
notification_builder:
Import #
Add the dependency to your file:
import 'package:notification_builder/notification_builder.dart';