live_stream_core 3.0.0
live_stream_core: ^3.0.0 copied to clipboard
Live Stream Core is a core control designed specifically for live streaming scenarios, providing a series of powerful API functionalities to help developers quickly implement voice chat room features
Live Stream Core #
English | 简体中文
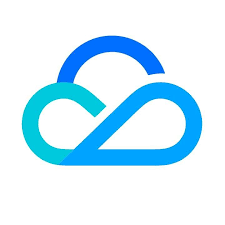
Product Features #
- Supports seat management
- Supports microphone management
- Supports custom seat layout
- Supports custom seat styles
Environment Preparation #
Flutter #
- Flutter 3.27.4 or higher.
- Dart 3.6.2 or higher.
Android #
- Android Studio 3.5 or higher.
- Android devices with Android 5.0 or higher.
iOS #
- Xcode 15.0 or higher.
- Ensure your project has a valid developer signature configured.
Getting Started #
Adding Dependencies #
Follow the documentation to add the live_stream_core
package as a pubspec dependency.
Activating Services #
To use the interactive live streaming barrage feature, ensure that you have activated the service.
-
Activate the Service
You can activate the service and obtain theSDKAppID
andSDKSecretKey
in the Console. -
Configure SDKAppID and SDKSecretKey
Open theexample/lib/debug/generate_test_user_sig.dart
file and fill in the obtainedSDKAppID
andSDKSecretKey
:static int sdkAppId = 0; // Replace with your activated SDKAppID static String secretKey = ''; // Replace with your activated SDKSecretKey
copied to clipboard
Example Experience #
If you want to quickly integrate or experience the interactive barrage effect, you can refer to the example code to integrate it into your application or directly run the example program for a hands-on experience.
APIs #
Creating a Voice Chat Room Core Component Instance #
You need to first create a SeatGridController
and then assign it to the voice chat room core component SeatGridWidget
.
SeatGridController
provides APIs, whileSeatGridWidget
is used to display the seat UI.
You can addSeatGridWidget
anywhere you need to display the seat UI.
final controller = SeatGridController();
SeatGridWidget(controller: controller);
Logging In #
To ensure you can use the API functionalities of the live streaming core component, you need to perform the login operation first.
const String userId = 'replace with your userId';
final result = await TUIRoomEngine.login(
'Replace with your activated SDKAppID',
userId,
'Replace with your userSig');
Starting a Voice Chat Room #
You can use the startVoiceRoom
API to start a voice chat room.
import 'package:live_stream_core/live_stream_core.dart';
import 'package:rtc_room_engine/rtc_room_engine.dart';
final roomInfo = TUIRoomInfo(roomId: 'replace with your roomId');
roomInfo.name = 'replace with your roomName';
roomInfo.seatMode = TUISeatMode.applyToTake;
roomInfo.isSeatEnabled = true;
roomInfo.roomType = TUIRoomType.livingRoom;
final result = await controller.startVoiceRoom(roomInfo);
Ending a Voice Chat Room #
You can use the stopVoiceRoom
API to end a voice chat room.
import 'package:live_stream_core/live_stream_core.dart';
final result = await controller.stopVoiceRoom();
Joining a Voice Chat Room #
You can use the joinVoiceRoom
API to join a voice chat room.
import 'package:live_stream_core/live_stream_core.dart';
final result = await controller.joinVoiceRoom('replace with your roomId');
Leaving a Voice Chat Room #
You can use the leaveVoiceRoom
API to leave a voice chat room.
import 'package:live_stream_core/live_stream_core.dart';
final result = await controller.leaveVoiceRoom();
Setting the Seat Mode #
You can use the updateRoomSeatMode
API to set the seat mode.
There are two seat modes: Free to Take (freeToTake) and Apply to Take (applyToTake).
In Free to Take mode, audience members can directly take a seat without the host's approval. In Apply to Take mode, audience members must first apply to the host and obtain approval before taking a seat.
import 'package:live_stream_core/live_stream_core.dart';
import 'package:rtc_room_engine/rtc_room_engine.dart';
const seatMode = TUISeatMode.freeToTake;
final result = await controller.updateRoomSeatMode(seatMode);
Applying for a Seat #
You can use the takeSeat
API to apply for a seat.
When using seat-related APIs, the seat index
seatIndex
ranges from[0, maxSeatCount)
.
ThemaxSeatCount
is related to your package's maximum number of multi-guests.
// API Definition
Future<RequestCallback> takeSeat(int seatIndex, int timeout) async;
// API Usage
import 'package:live_stream_core/live_stream_core.dart';
final result = await controller.takeSeat(1, 30);
Voluntarily Leaving a Seat #
You can use the leaveSeat
API to voluntarily leave a seat.
import 'package:live_stream_core/live_stream_core.dart';
final result = await controller.leaveSeat();
Moving Seats #
You can use the moveToSeat
API to switch seat positions while on the seat.
import 'package:live_stream_core/live_stream_core.dart';
final destinationIndex = 2; // Replace with the seat index you want to move to
final result = await controller.moveToSeat(destinationIndex);
Locking a Seat #
You can use the lockSeat
API to lock a seat.
The
lockSeat
API supports the following capabilities in voice chat room scenarios:
- Lock a specific seat to prevent taking it: Set
lockSeat
inTUISeatLockParams
totrue
.- Lock a specific seat to disable audio: Set
lockAudio
inTUISeatLockParams
totrue
.
// API Definition
Future<TUIActionCallback> lockSeat(int index, TUISeatLockParams lockMode) async;
// API Usage
import 'package:live_stream_core/live_stream_core.dart';
import 'package:rtc_room_engine/rtc_room_engine.dart';
final lockMode = TUISeatLockParams();
lockMode.lockSeat = true;
final result = await controller.lockSeat(3, lockMode);
Inviting a User to a Seat #
You can use the takeUserOnSeatByAdmin
API to invite an audience member to a specific seat.
// API Definition
Future<RequestCallback> takeUserOnSeatByAdmin(int seatIndex, String userId, int timeout) async;
// API Usage
import 'package:live_stream_core/live_stream_core.dart';
final result = await controller.takeUserOnSeatByAdmin(4, 'replace with the userId of the audience you want to invite', 30);
Kicking a User Off a Seat #
You can use the kickUserOffSeatByAdmin
API to kick a user with the specified userId
off the seat.
import 'package:live_stream_core/live_stream_core.dart';
final result = await controller.kickUserOffSeatByAdmin('replace with the userId of the audience you want to kick off from seat');
Responding to Seat Requests #
You can use the responseRemoteRequest
API to approve or reject seat requests from a user with the specified userId
.
import 'package:live_stream_core/live_stream_core.dart';
final result = await controller.responseRemoteRequest('replace with userId', true);
Canceling a Seat Request #
You can use the cancelRequest
API to cancel a seat request from a user with the specified userId
.
This API can cancel your own seat request or an invitation request to a specific user.
import 'package:live_stream_core/live_stream_core.dart';
final result = await controller.responseRemoteRequest('replace with userId', true);
Turning On the Microphone #
You can use the startMicrophone
API to turn on the microphone device.
import 'package:live_stream_core/live_stream_core.dart';
final result = await controller.startMicrophone();
Turning Off the Microphone #
You can use the stopMicrophone
API to turn off the microphone device.
import 'package:live_stream_core/live_stream_core.dart';
controller.stopMicrophone();
Muting the Audio Stream #
You can use the muteMicrophone
API to mute the local audio stream.
import 'package:live_stream_core/live_stream_core.dart';
final result = await controller.muteMicrophone();
Unmuting the Audio Stream #
You can use the unmuteMicrophone
API to unmute the local audio stream.
import 'package:live_stream_core/live_stream_core.dart';
final result = await controller.unmuteMicrophone();
Customizing Seat Layout #
You can use the setLayoutMode
API to customize the seat layout.
The
setLayoutMode
API provides three built-in layouts: Focus (focus), Grid (grid), and Vertical (vertical), and also supports custom layouts (free).
// API Definition
void setLayoutMode(LayoutMode layoutMode, SeatWidgetLayoutConfig? layoutConfig);
// API Usage
import 'package:live_stream_core/live_stream_core.dart';
controller.setLayoutMode(LayoutMode.grid, null); // Example: Using the built-in grid layout
final rowConfig = SeatWidgetLayoutRowConfig(
count: 2,
seatSpacing: 20.0,
seatSize: const Size(80, 80),
alignment: SeatWidgetLayoutRowAlignment.spaceBetween);
final layoutConfig = SeatWidgetLayoutConfig(
rowConfigs: [rowConfig, rowConfig]);
controller.setLayoutMode(LayoutMode.free, layoutConfig); // Example: Using a custom layout
Customizing Seat Styles #
You can use the seatWidgetBuilder
parameter of SeatGridWidget
to customize the UI style of specific seats.
// seatWidgetBuilder Definition
typedef SeatWidgetBuilder = Widget Function(
BuildContext context,
ValueNotifier<TUISeatInfo> seatInfoNotifier,
ValueNotifier<int> volumeNotifier);
// Usage Example
import 'package:live_stream_core/live_stream_core.dart';
import 'package:rtc_room_engine/rtc_room_engine.dart';
SeatGridWidget(
controller: controller,
onSeatWidgetTap: (TUISeatInfo seatInfo) {
// debugPrint('click seatWidget index:${seatInfo.index}');
},
seatWidgetBuilder: (
BuildContext context,
ValueNotifier<TUISeatInfo> seatInfoNotifier,
ValueNotifier<int> volumeNotifier) {
// Return your custom seat widget
return Container();
}
)
Recommended Resources #
If you want to quickly integrate a fully-featured interactive live streaming UI into your application, you can integrate the Interactive Live Streaming (tencent_live_uikit) component.
Feedback and Support #
For any inquiries or feedback, please contact: info_rtc@tencent.com.