keyboard_hider 1.0.0
keyboard_hider: ^1.0.0 copied to clipboard
This tiny Flutter package help you hide the keyboard. With convenient helper methods and the KeyboardHider widget.
keyboard_hider
#
This tiny Flutter package help you hide the keyboard. With convenient helper methods and the
KeyboardHider
widget.
Have you ever heard that in Flutter, everything is a widget? I have, so I decided to create a simple widget that helps you hide your users' keyboard easily on your forms.
Important links #
- Read the source code and star the repo on GitHub
- Open an issue on GitHub
- See package on pub.dev
- Read the docs on pub.dev
- Stack Overflow - How can I dismiss the on screen keyboard?
- Flutter Docs -
GestureDetector
,SystemChannels
,SystemChannels.textInput
,FocusScopeNode
,FocusNode.unfocus
If you enjoy using this package, a thumbs up on pub.dev would be highly appreciated! 👍💙🚀
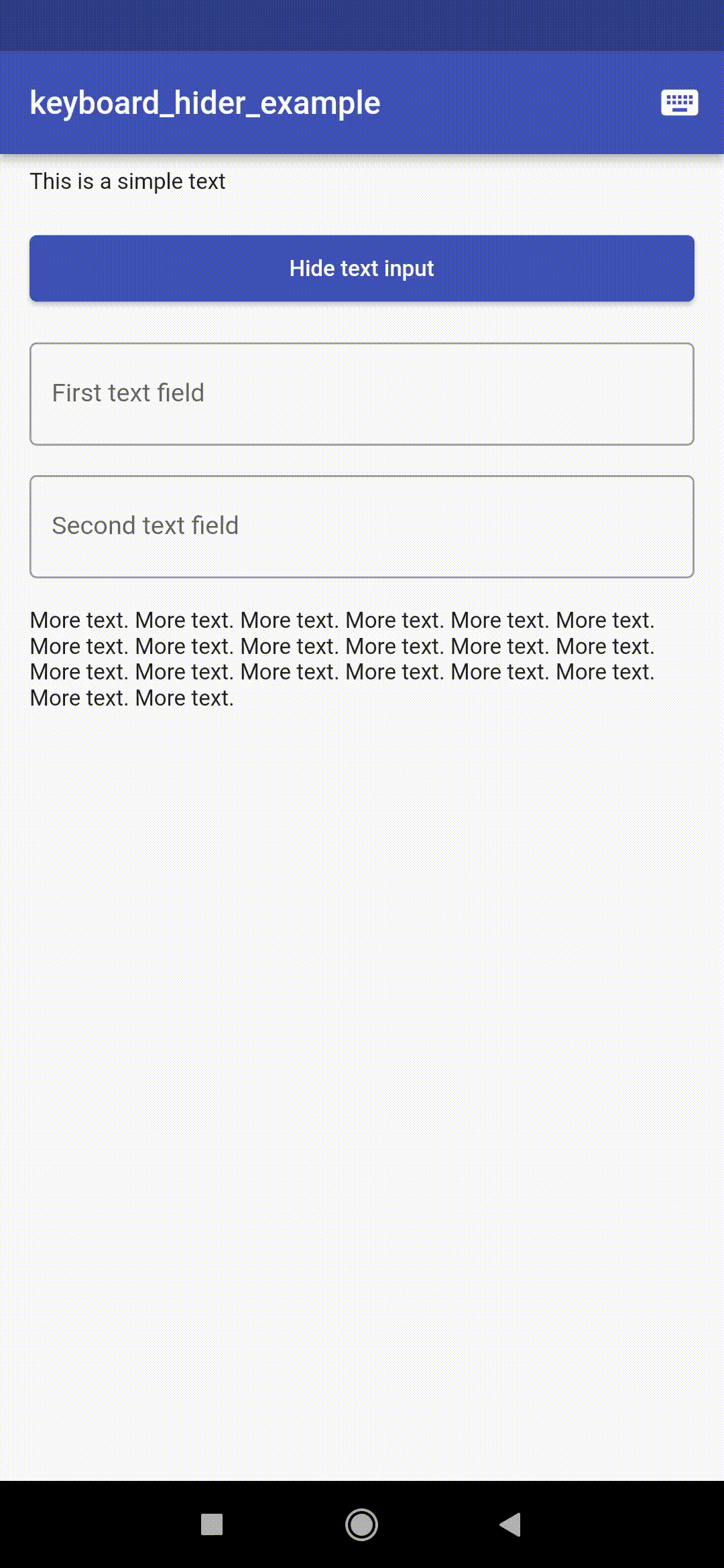
Motivation #
While interacting with our users, we realized that not every user knows their keyboard very well and that they expect the keyboard to be hidden when the click outside a text field. We decided to give our users the option to hide their keyboard for forms when they clicked outside a text field.
I searched how to do that, and I found this question on Stack Overflow.
I wasn't really satisfied with adding repeated code everywhere, as remembering how to configure the GestureDetector
, and what is the correct way to unfocus
(hide the keyboard) is a little annoying.
You can see my answer on Stack Overflow from 2019: I decided to wrap this functionality in a convenient Flutter widget that simplifies hiding the keyboard whenever the user taps on the screen outside a text field (similar to how keyboard hiding works on the web).
Since then, I worked on a couple of projects, and I've seen that this pattern is discussed again and again, so now I decided to publish this package so that I (and everyone else) can quickly and simply add this functionality to our Flutter apps.
Usage #
Wrap your widget that should detect touches with a KeyboardHider
widget and upon touch, it should hide the keyboard if visible.
The widget doesn't interfere with text fields, so your users can seamlessly switch and jump between text fields, even if the text fields are wrapped in a KeyboardHider
widget.
import 'package:keyboard_hider/keyboard_hider.dart';
class YourWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
// When you now tap on the child, the keyboard should be dismissed.
return KeyboardHider(
child: Text('your widgets...'),
);
}
}
The package also includes two helper functions that simplified hiding your keyboard.
The unfocus(BuildContext context)
function takes the context and dismisses the keyboard by un-focusing the context's FocusScopeNode
.
The hideTextInput()
function invokes the TextInput.hide
method on the SystemChannels.textInput
method channel.
If needed, you can also pass a HideMode
value to the KeyboardHider
.
By default, the KeyboardHider
widget uses the unfocus
approach, but you can change that to hideTextInput
:
class YourOtherWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
// When you now tap on the child, the keyboard should be hidden.
return KeyboardHider(
mode: HideMode.hideTextInput,
child: Text('your widgets...'),
);
}
}
With hideTextInput
, the keyboard will be hidden, but it does not unfocus the current focus scope node.
This means that if a text field was in focus, it will stay in focus, only the keyboard will get hidden.
You can find the example app on GitHub and on pub.dev.
If you still have questions about the structure of this package, I encourage you to take another look at the docs.