gamepads_windows 0.1.1
gamepads_windows: ^0.1.1 copied to clipboard
Windows implementation of gamepads, a Flutter plugin to handle gamepad input across multiple platforms.
gamepads #
A Flutter plugin to handle gamepad input across multiple platforms.
Note: This plugin is still in beta. All APIs are subject to change. Any feedback is appreciated.
Gamepads is a Flutter plugin to handle gamepad (or joystick) input across multiple platforms.
It supports multiple simultaneously connected gamepads, and will automatically detect and listen to new connections.
Getting Started #
The list
method will list all currently connected gamepads:
final gamepads = await Gamepads.list();
// ...
This uses the data class GamepadController
, which has an id
and a user-facing name
.
And the events
stream will broadcast input events from all gamepads:
Gamepads.events.listen((event) {
// ...
});
You can also listen to events only for a specific gamepad with eventsByGamepad
.
Events are described by the data class GamepadEvent
:
class GamepadEvent {
/// The id of the gamepad controller that fired the event.
final String gamepadId;
/// The timestamp in which the event was fired, in milliseconds since epoch.
final int timestamp;
/// The [KeyType] of the key that was triggered.
final KeyType type;
/// A platform-dependant identifier for the key that was triggered.
final String key;
/// The current value of the key.
final double value;
// ...
}
Next Steps #
As mentioned, this is still a WIP library. Not only APIs are expected to change if needed, but we plan to add more features, like:
- stream to listen for connecting/disconnecting gamepads
- get current state of a gamepad
- add support for web and even mobile
If you are interested in helping, please reach out! You can use GitHub or our Discord server.
Support #
The simplest way to show us your support is by giving the project a star! ⭐
If you want, you can also support us monetarily by donating through OpenCollective:

Through GitHub Sponsors:
Or by becoming a patron on Patreon:
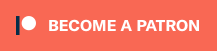