djangoflow_auth_google 0.1.0+8
djangoflow_auth_google: ^0.1.0+8 copied to clipboard
djangoflow_auth_google is the Google authentication provider for djangoflow_auth package
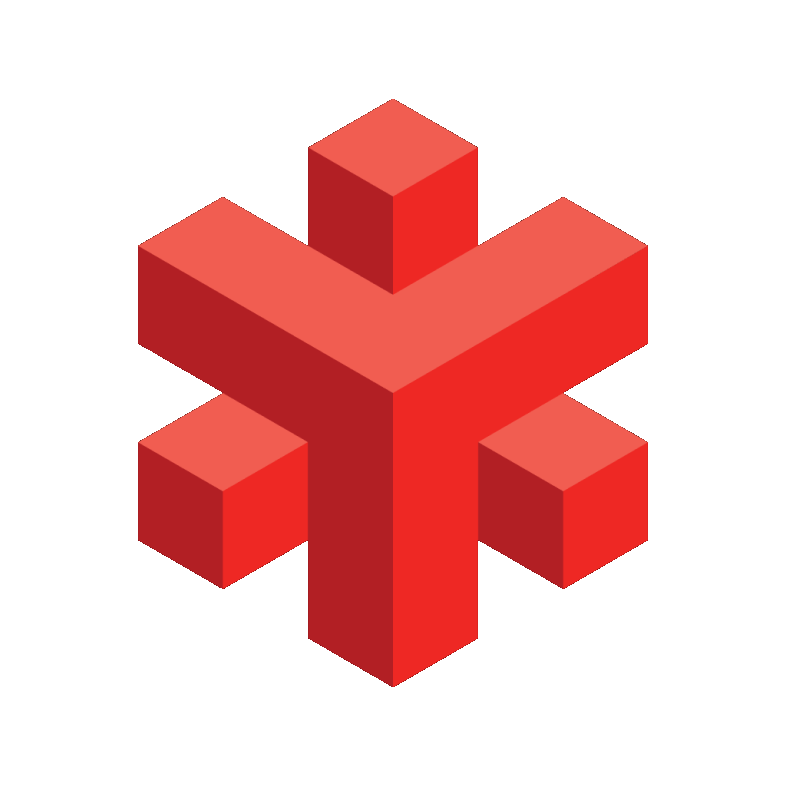
🔑 DjangoFlow Auth Google Package 🔑
djangoflow_auth_google is the Google authentication provider for djangoflow_auth. It allows seamless integration of Google login functionalities with your Flutter app. Securely manage user authentication using Google Sign-In. Simple and effective! 🔒
🚀 Features 🚀
- Integrate Google Sign-In with djangoflow_auth.
- Easy-to-use API for Google authentication in your Flutter app.
- Logout function to securely disconnect Google Sign-In.
📦 Installation 📦
dependencies:
djangoflow_auth: <latest_version>
djangoflow_auth_google: <latest_version>
google_sign_in: <latest_version> # Add the appropriate version of google_sign_in
Run flutter pub get
to fetch the package.
Note: Follow google_sign_in package for platform related configuration.
🔧 Usage 🔧
To perform Google authentication using `djangoflow_auth_google`, you can use the `GoogleSocialLogin` class:import 'package:djangoflow_auth/djangoflow_auth.dart';
import 'package:djangoflow_auth_google/djangoflow_auth_google.dart';
import 'package:google_sign_in/google_sign_in.dart';
void main() {
final googleSignIn = GoogleSignIn(scopes: ['email']); // Set up Google Sign-In scopes
final googleSocialLogin = GoogleSocialLogin(googleSignIn: googleSignIn);
// Perform Google Sign-In
final googleAccount = await googleSocialLogin.login();
// Handle the Google account or perform further actions
if (googleAccount != null) {
// Successfully signed in with Google
// Handle user account or continue with your app
} else {
// Google Sign-In failed
// Handle the error
}
// To disconnect Google Sign-In
await googleSocialLogin.logout();
}
Read more here for detailed example on how to use it with djangoflow_auth
this: https://pub.dev/packages/djangoflow_auth#setting-up-authcubit
🌐 Google Sign-In Button Variants 🌐
`djangoflow_auth_google` package provides different button implementations based on the platform out of the box:- Web: GoogleSignInWebButton - Handles Google Sign-In for web platforms automatically following GSI API.(Uses
GoogleSignInButton
under the hood) - Mobile (iOS/Android): GoogleSignInButton - A customizable Flutter button for Google Sign-In on mobile platforms.
🛠️ Configuration and Customization 🛠️
To customize the appearance of the Google Sign-In button on **Web**, you can use the provided `GSIButtonConfigWrapper` class:import 'package:djangoflow_auth_google/djangoflow_auth_google.dart';
final config = GSIButtonConfigWrapper(
type: GSIWrapperButtonType.standard,
theme: GSIWrapperButtonTheme.filledBlue,
size: GSIWrapperButtonSize.large,
text: GSIWrapperButtonText.signinWith,
);
// Pass the configuration to the Google Sign-In button
final googleSignInButton = GoogleSignInButton(
configurationWrapper: config,
onPressed: () {
// Handle the button press
},
);
You can customize the button type, theme, size, text, shape, logo alignment, locale, and minimum width.
🌐 Google Sign-In Button on Web 🌐
For web platforms, you can use the `GoogleSignInWebButton` widget, which detects the current user's authorization status and conditionally shows the Google Sign-In button or a placeholder. The `enableSilentLogin` parameter can be used to enable silent login on the web.import 'package:djangoflow_auth_google/djangoflow_auth_google.dart';
// with BlocProvider it can be like this
final googleSignIn = (context
.read<AuthCubit>()
.socialLogins
.getSocialLoginByProvider(
ProviderEnum.googleOauth2,
) as GoogleSocialLogin)
.googleSignIn
GoogleSignInWebButton(
googleSignIn: googleSignIn,
enableSilentLogin: true,
onSignIn: (GoogleSignInAccount? googleSignInAccount) {
// Handle the successful Google Sign-In
},
)