dependency_injection_flutter 2.0.0
dependency_injection_flutter: ^2.0.0 copied to clipboard
dependency injection for flutter. inject factories or singletons. Easy to develop, easy to test.
Dependency Injection for Flutter #
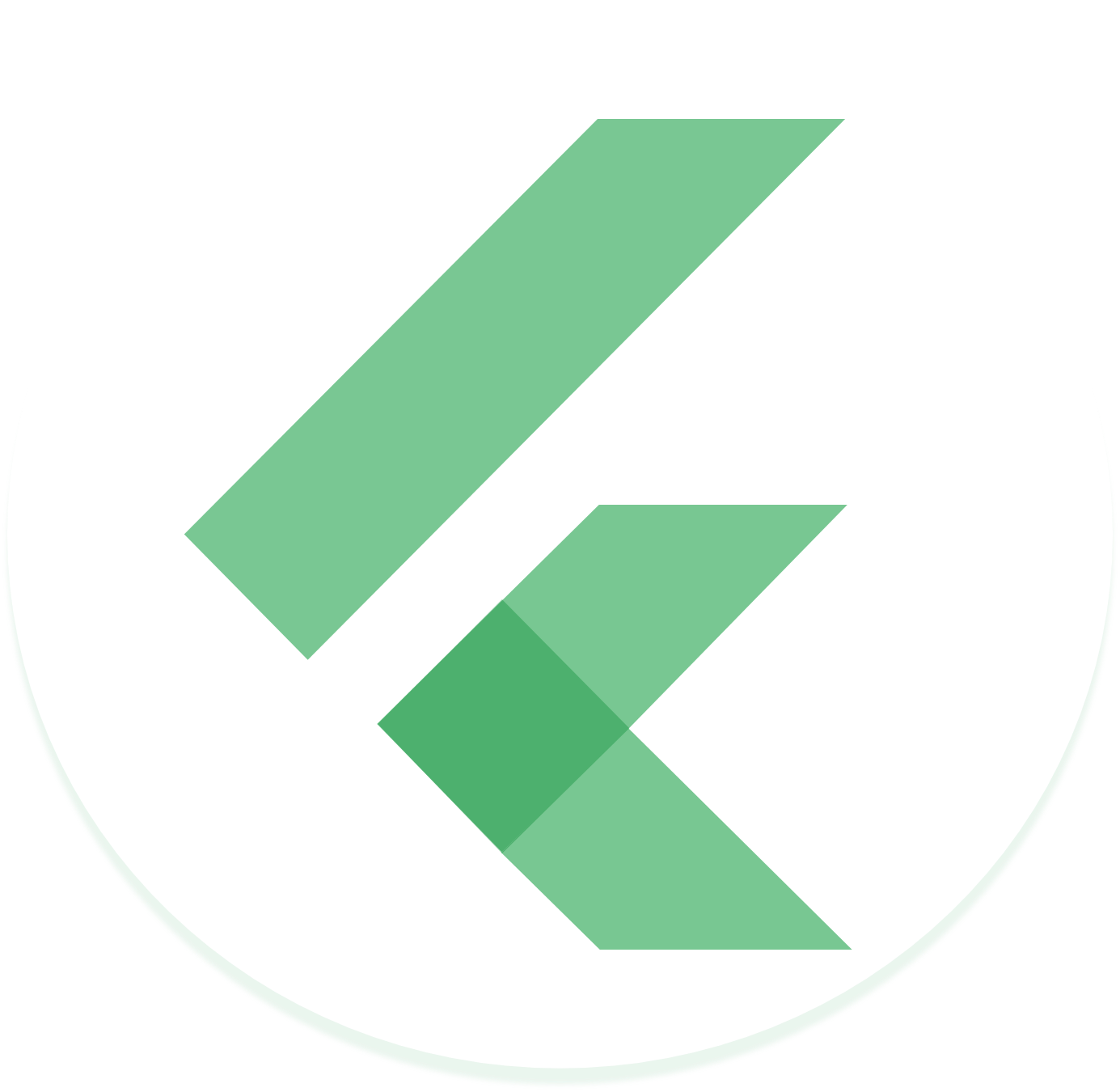
Flutter Brazil
Injecting #
To inject a singleton:
The Singleton instance will be unique. And every time you try to fetch,
Injector will return the very same instance.
Injector.instance.inject<MyInstanceType>(()=>MyInstance());
By default, all injections made by the inject method will have
the type of a Singleton, but you can change it with tye type arg.
Injecting a lazy singleton
A lazy singleton will only be instantiated once will call it from Injector. It helps a lot in saving RAM.
Injector.instance.inject<MyInstanceType>(()=>MyInstance(), type: InjectionType.lazySingleton);
To inject a factory:
A factory is the instance type that will return always as a brand new instance.
It can be useful to get a service for example.
Injector.instance.inject<MyInstanceType>(()=>MyInstance(), type: InjectionType.factory);
and get all of them with:
var myInstance = Injector.instance.get<MyInstanceType>();
Get your controller in your widget
class MyView extends StatelessWidget with InjectionMixin<MyController> {
void doSomething() {
controller.onDoSomething();
}
@override
Widget build(BuildContext context) {
return Container();
}
}
Testing with Injector
One of the great qualities of Injector is to provide an easy way to test your code in unit tests.
In your class test, at the setUpAll body, use:
Injector injector;
setUpAll(() {
injector = Injector.instance;
injector.inject<MyServiceInterface>(()=> MyMockedService());
});
test("Testing the get all", () {
// Since you injected the MyServiceInterface
// it will return a class of MyServiceInterface type,
// but with the MyMockedService implementation.
var myService = injector.get<MyServiceInterface>();
// just as you were going to do in your real MyServiceImpl
var result = myService.getAll();
})