dart_table 0.0.2
dart_table: ^0.0.2 copied to clipboard
Flutter data table with selection, sorting, filtering, and pagination. Also supports split view.
dart_table #
Flutter data table with selection, sorting, filtering, and pagination. Also supports split view.
📸 Screenshots #
- Table
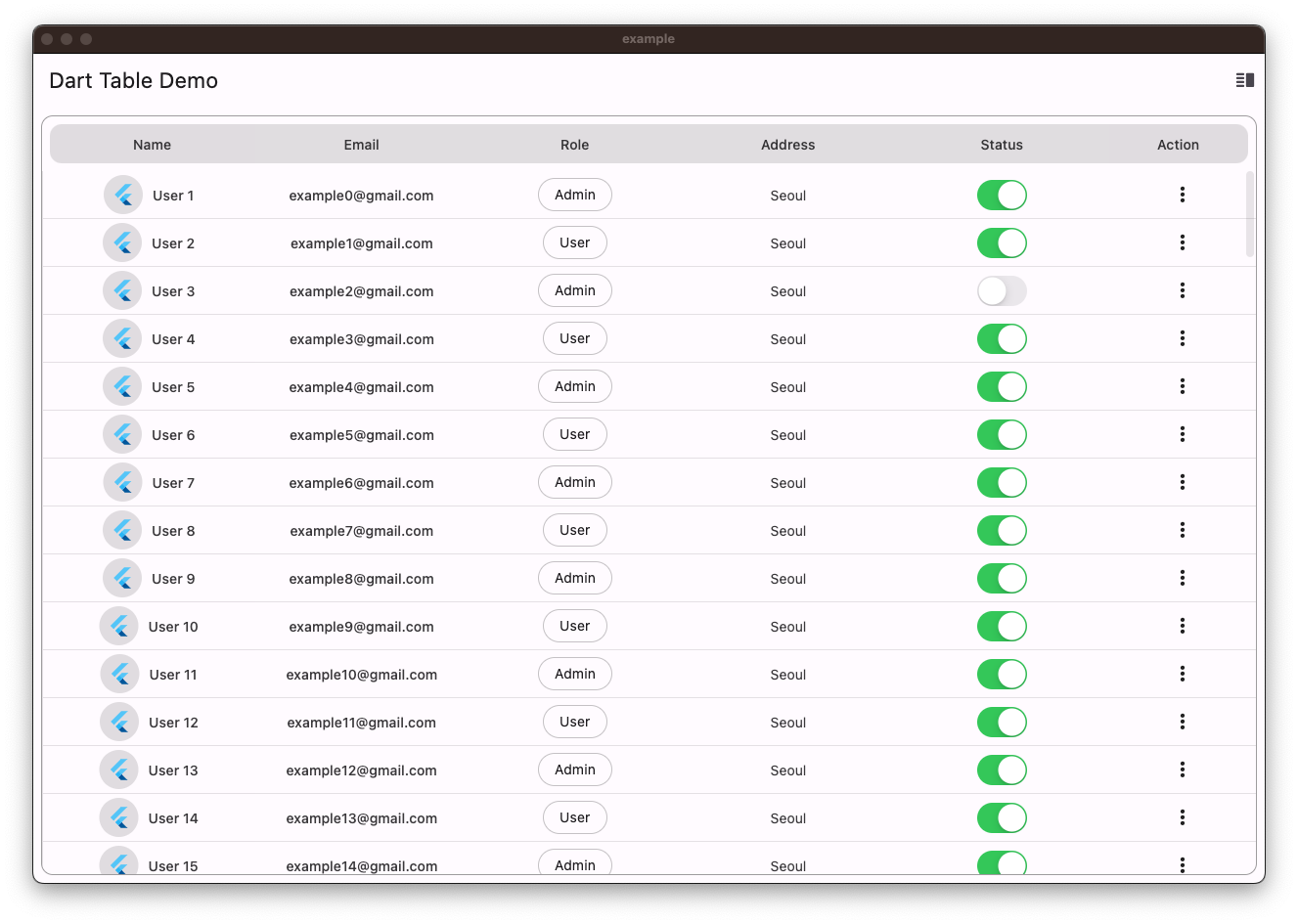
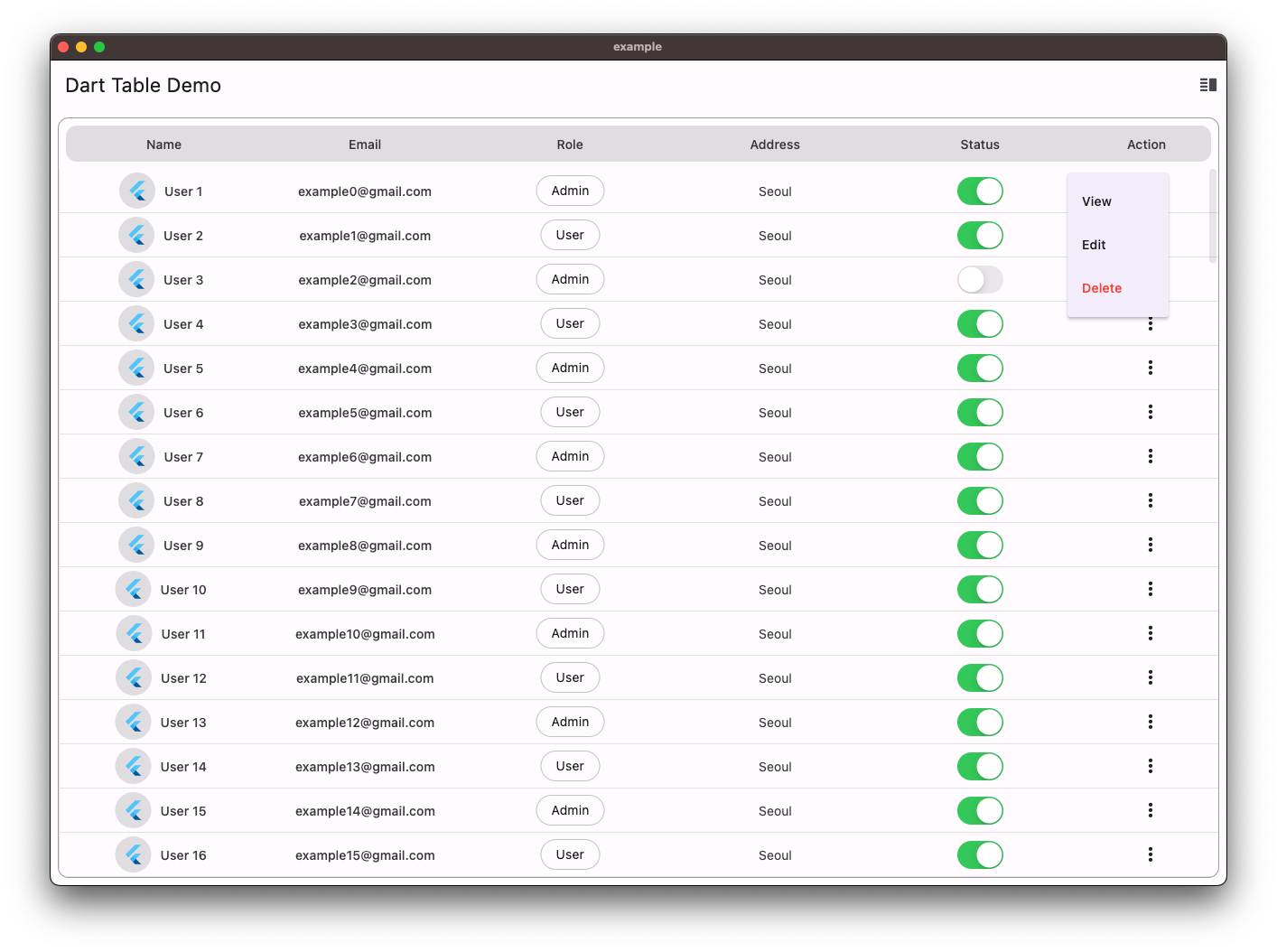
- Table with Split view
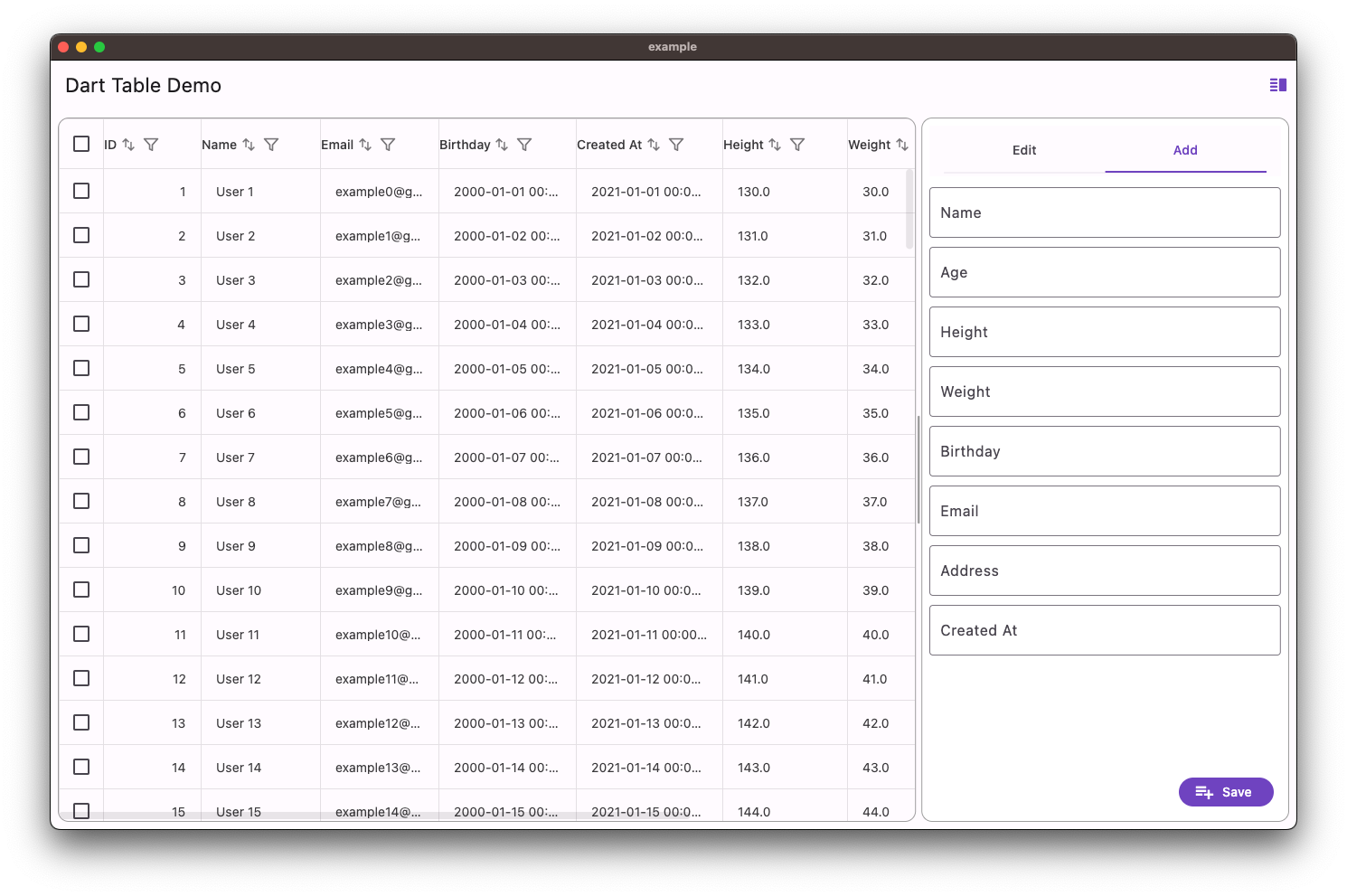
Setup #
Pubspec changes:
dependencies:
dart_table: latest
Getting Started #
import 'package:dart_table/dart_table.dart';
Set up your data model #
class UserModel {
UserModel({
this.id,
this.name,
this.email,
this.image,
this.address,
this.role,
this.status,
this.createdAt,
});
final int? id;
final String? name;
final String? email;
final String? image;
final String? address;
final String? role;
final String? status;
final DateTime? createdAt;
}
Set up your data source #
class UserModelSource extends DataGridSource {
/// Dummy data for example
static List<UserModel> get dummyData => List.generate(
120,
(index) => UserModel(
id: index + 1,
name: 'User ${index + 1}',
image: "https://mohesu.com/200/300?random=$index",
email: "example$index@gmail.com",
address: "Address $index",
role: index % 2 == 0 ? "Admin" : "User",
status: index != 2 ? "Active" : "Inactive",
createdAt: DateTime(2021, 1, 1).add(Duration(days: index)),
),
).toList();
static List<String> get attributes => [
"name",
"email",
"role",
"address",
"status",
"action",
];
static List<GridColumn> get columns => attributes.map<GridColumn>(
(attribute) {
final index = attributes.indexOf(attribute);
return GridColumn(
columnName: attribute,double.nan,
label: Text(
attribute.substring(0, 1).toUpperCase() +
attribute.substring(1),
),
);
},
).toList();
List<DataGridRow> dataGridRows = dummyData
.map<DataGridRow>(
(user) => DataGridRow(
cells: [
DataGridCell<String>(
columnName: 'name',
value: user.name,
),
DataGridCell<String>(
columnName: 'email',
value: user.email,
),
DataGridCell<String>(
columnName: 'role',
value: user.role,
),
DataGridCell<String>(
columnName: 'address',
value: user.address,
),
DataGridCell<String>(
columnName: 'status',
value: user.status,
),
const DataGridCell<String>(
columnName: 'action',
value: 'action',
),
],
),
)
.toList();
@override
List<DataGridRow> get rows => dataGridRows;
@override
DataGridRowAdapter? buildRow(DataGridRow row) {
return DataGridRowAdapter(
cells: row.getCells().map<Widget>(
(dataGridCell) {
return Container(
alignment: Alignment.center,
padding: const EdgeInsets.symmetric(horizontal: 16.0),
child: Text(
dataGridCell.value.toString(),
overflow: TextOverflow.ellipsis,
),
);
},
).toList(),
);
}
}
Usage of [DartTable] #
DartTable(
columns: UserModelSource.columns,
source: UserModelSource.dummyData,
/// Right side widget of [SplitView] (optional)
child: Container(
color: Colors.white,
child: Center(
child: Text('Right side'),
),
),
);
- For more details, please refer to the example folder.
💰You can help me by Donating #
👨🏻💻Contribute to the project #
All contributions are welcome.
👨🏻💻Contributors #
Made with contrib.rocks.