code_text_field 1.0.0-3
code_text_field: ^1.0.0-3 copied to clipboard
A customizable code field supporting syntax highlighting
CodeField #
A customizable code text field supporting syntax highlighting
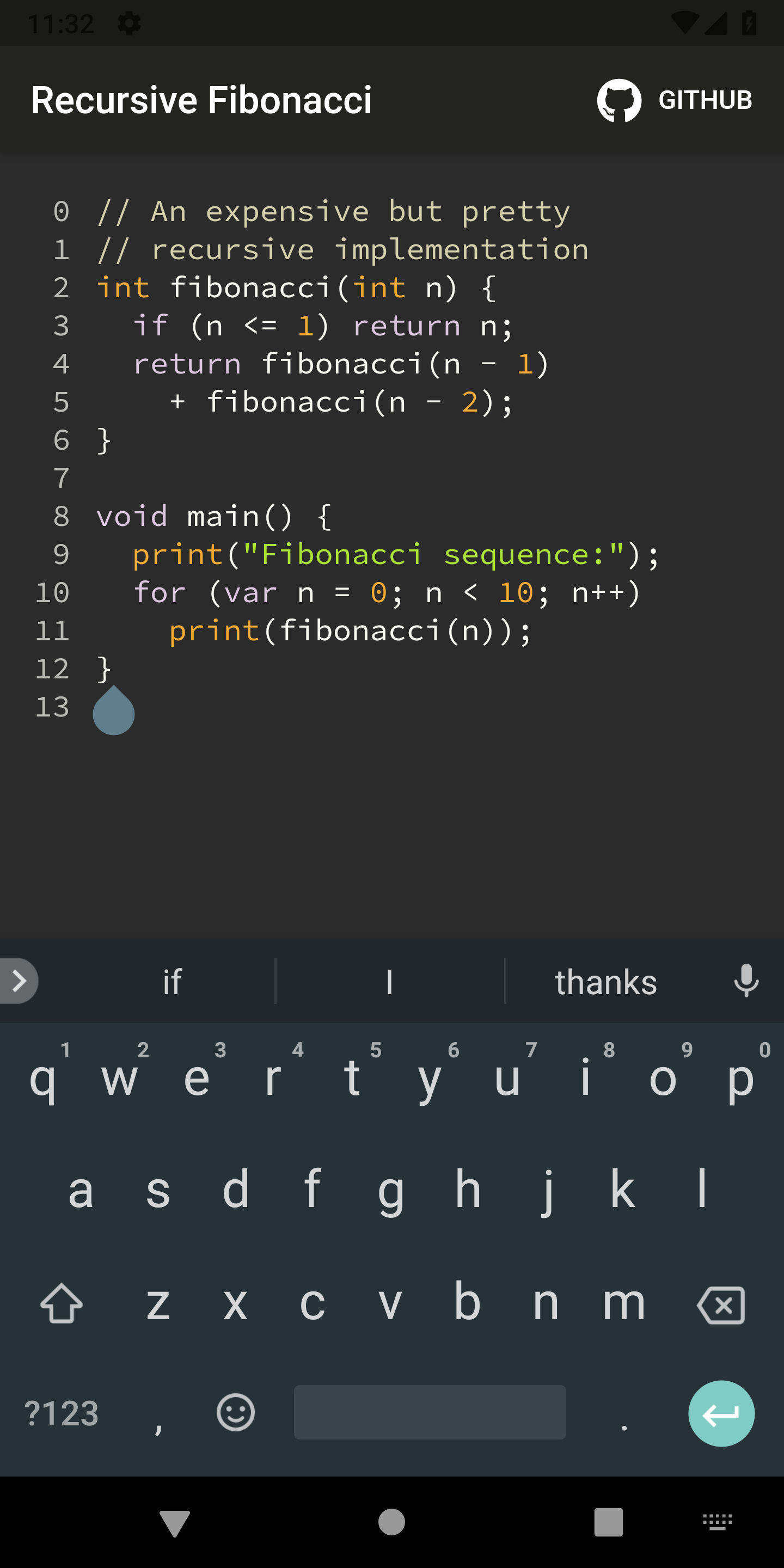
Live demo #
A live demo showcasing a few language / themes combinaisons
Features #
- Code highlight for 189 built-in languages with 90 themes thanks to flutter_highlight
- Easy language highlight customization through the use of theme maps
- Fully customizable code field style through a TextField like API
- Handles horizontal/vertical scrolling and vertical expansion
- Works on Android, iOS, and Web
Installing #
In the pubspec.yaml
of your flutter project, add the following dependency:
dependencies:
...
code_text_field: <latest_version>
In your library add the following import:
import 'package:code_text_field/code_field.dart';
Simple example #
A CodeField widget works with a CodeController which dynamically parses the text input according to a language and renders it with a theme map
import 'package:flutter/material.dart';
import 'package:code_text_field/code_field.dart';
// Import the language & theme
import 'package:highlight/languages/dart.dart';
import 'package:flutter_highlight/themes/monokai-sublime.dart';
class CodeEditor extends StatefulWidget {
@override
_CodeEditorState createState() => _CodeEditorState();
}
class _CodeEditorState extends State<CodeEditor> {
CodeController? _codeController;
@override
void initState() {
super.initState();
final source = "void main() {\n print(\"Hello, world!\");\n}";
// Instantiate the CodeController
_codeController = CodeController(
text: source,
language: dart,
theme: monokaiSublimeTheme,
);
}
@override
void dispose() {
_codeController?.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return CodeField(
controller: _codeController!,
textStyle: TextStyle(fontFamily: 'SourceCode'),
);
}
}
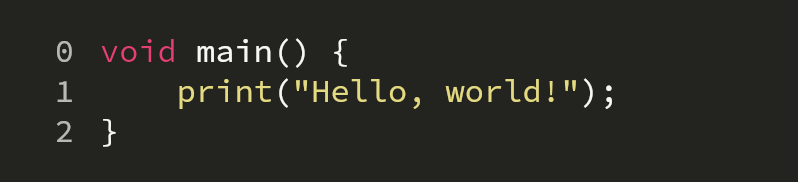
Here, the monospace font Source Code Pro has been added to the assets folder and to the pubspec.yaml file
Parser options #
On top of a language definition, world-wise styling can be specified in the stringMap field
_codeController = CodeController(
//...
stringMap: {
"Hello": TextStyle(fontWeight: FontWeight.bold, color: Colors.red),
"world": TextStyle(fontStyle: FontStyle.italic, color: Colors.green),
},
);
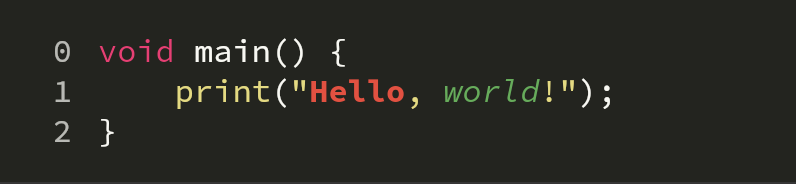
More complex regexes may also be used with the patternMap. When a language is used though, its regexes patterns take precedence over patternMap and stringMap.
_codeController = CodeController(
//...
patternMap: {
r"\B#[a-zA-Z0-9]+\b":
TextStyle(fontWeight: FontWeight.bold, color: Colors.purpleAccent),
},
);
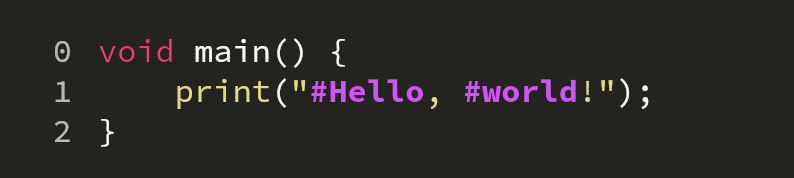
Both patternMap and stringMap can be used without specifying a language
_codeController = CodeController(
text: source,
patternMap: {
r'".*"': TextStyle(color: Colors.yellow),
r'[a-zA-Z0-9]+\(.*\)': TextStyle(color: Colors.green),
},
stringMap: {
"void": TextStyle(fontWeight: FontWeight.bold, color: Colors.red),
"print": TextStyle(fontWeight: FontWeight.bold, color: Colors.blue),
},
);
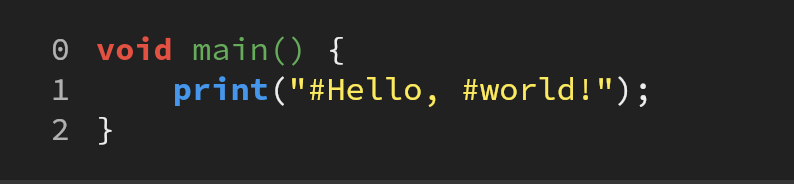
API #
CodeField #
CodeField({
Key? key,
required this.controller,
this.minLines,
this.maxLines,
this.expands = false,
this.background,
this.decoration,
this.textStyle,
this.padding = const EdgeInsets.symmetric(horizontal: 8.0),
this.scrollPadding = const EdgeInsets.symmetric(vertical: 8.0),
this.lineNumberStyle = const LineNumberStyle(),
this.cursorColor,
this.textSelectionTheme,
})
LineNumberStyle({
this.width = 42.0,
this.textAlign = TextAlign.right,
this.padding = const EdgeInsets.only(right: 10.0),
this.textStyle,
this.background,
})
CodeController #
CodeController({
String? text,
this.language,
this.theme,
this.patternMap,
this.stringMap,
})
Limitations #
- Autocomplete disabling on android doesn't work yet
- The TextField cursor doesn't seem to be handling space keys properly on the web platform. Pending issue resolution