zego_uikit_prebuilt_call 3.3.13
zego_uikit_prebuilt_call: ^3.3.13 copied to clipboard
PrebuiltCall is a full-featured call kit that provides a ready-made call invitation, voice/video chat, device detection, etc. Add a voice/video call to your app in minutes.
Overview #
Call Kit is a prebuilt feature-rich call component, which enables you to build one-on-one and group voice/video calls into your app with only a few lines of code.
And it includes the business logic with the UI, you can add or remove features accordingly by customizing UI components.
One-on-one call | Group call |
---|---|
![]() |
![]() |
When do you need the Call Kit #
-
Build apps faster and easier
When you want to prototype 1-on-1 or group voice/video calls ASAP
Consider speed or efficiency as the first priority
Call Kit allows you to integrate in minutes
-
Customize UI and features as needed
When you want to customize in-call features based on actual business needs
Less time wasted developing basic features
Call Kit includes the business logic along with the UI, allows you to customize features accordingly
To finest-grained build a call app, you may try our Video Call SDK to make full customization.
Embedded features #
- Ready-to-use 1-on-1/group calls
- Customizable UI styles
- Real-time sound waves display
- Device management
- Switch views during a 1-on-1 call
- Extendable menu bar
- Participant list
- Call invitation
- Custom call ringtones
Recommended resources #
- I want to get started to implement a basic call swiftly
- I want to get the Sample Code
- I want to get started to implement a call with call invitation
- To configure prebuilt UI for a custom experience
Prerequisites #
- Go to ZEGOCLOUD Admin Console, and do the following:
- Create a project, get the AppID and AppSign.
- Activate the In-app Chat service (as shown in the following figure).
Quick start #
Watch the video as belowed:
Integrate the SDK #
Add ZegoUIKitPrebuiltCall as dependencies #
Run the following code in your project root directory:
flutter pub add zego_uikit_prebuilt_call
Import the SDK #
Now in your Dart code, import the prebuilt Call Kit SDK.
import 'package:zego_uikit_prebuilt_call/zego_uikit_prebuilt_call.dart';
Using the ZegoUIKitPrebuiltCall in your project #
- Go to ZEGOCLOUD Admin Console, get the
appID
andappSign
of your project. - Specify the
userID
anduserName
for connecting the Call Kit service. - Create a
callID
that represents the call you want to make. userID
andcallID
can only contain numbers, letters, and underlines (_).- Users that join the call with the same
callID
can talk to each other.
class CallPage extends StatelessWidget {
const CallPage({Key? key, required this.callID}) : super(key: key);
final String callID;
@override
Widget build(BuildContext context) {
return ZegoUIKitPrebuiltCall(
appID: yourAppID, // Fill in the appID that you get from ZEGOCLOUD Admin Console.
appSign: yourAppSign, // Fill in the appSign that you get from ZEGOCLOUD Admin Console.
userID: 'user_id',
userName: 'user_name',
callID: callID,
// You can also use groupVideo/groupVoice/oneOnOneVoice to make more types of calls.
config: ZegoUIKitPrebuiltCallConfig.oneOnOneVideoCall()
..onOnlySelfInRoom = (context) {
if (MiniOverlayPageState.idle !=
ZegoMiniOverlayMachine().state()) {
ZegoMiniOverlayMachine()
.changeState(MiniOverlayPageState.idle);
} else {
Navigator.of(context).pop();
}
},
);
}
}
Now, you can make a new call by navigating to this CallPage
.
Quick start (with call invitation) #
Integrate the SDK #
Add ZegoUIKitPrebuiltCallWithInvitation as dependencies #
- Run this command with Flutter:
flutter pub add zego_uikit_prebuilt_call
flutter pub add zego_uikit_signaling_plugin
- Run the following code in your project root directory to install all dependencies.
flutter pub get
Import the SDK #
Now in your Dart code, import the prebuilt Call Kit SDK.
import 'package:zego_uikit_prebuilt_call/zego_uikit_prebuilt_call.dart';
import 'package:zego_uikit_signaling_plugin/zego_uikit_signaling_plugin.dart';
Integrate the SDK with the call invitation feature #
Props of ZegoUIKitPrebuiltCallWithInvitation component
Property | Type | Required | Description |
---|---|---|---|
appID | int | Yes | The App ID you get from [ZEGOCLOUD Admin Console](https://console.zegocloud.com). |
appSign | String | Yes | The App Sign you get from [ZEGOCLOUD Admin Console](https://console.zegocloud.com). |
userID | String | Yes | `userID` can be something like a phone number or the user ID on your own user system. userID can only contain numbers, letters, and underlines (_). |
userName | String | Yes | `userName` can be any character or the user name on your own user system. |
plugins | List< IZegoUIKitPlugin > | Yes | Fixed value. Set it to `ZegoUIKitSignalingPlugin` as shown in the sample. |
ringtoneConfig | ZegoRingtoneConfig | No | `ringtoneConfig.incomingCallPath` and `ringtoneConfig.outgoingCallPath` is the asset path of the ringtone file, which requires you a manual import. To know how to import, refer to [Custom prebuilt UI](https://docs.zegocloud.com/article/14748). |
requireConfig | ZegoUIKitPrebuiltCallConfig Function( ZegoCallInvitationData)? | No | This method is called when you receive a call invitation. You can control the SDK behaviors by returning the required config based on the data parameter. For more details, see [Custom prebuilt UI](https://docs.zegocloud.com/article/14748). |
notifyWhenAppRunningInBackgroundOrQuit | bool | No | Change `notifyWhenAppRunningInBackgroundOrQuit` to false if you don't need to receive a call invitation notification while your app running in the background or quit. |
isIOSSandboxEnvironment | bool | No | To publish your app to TestFlight or App Store, set the `isIOSSandboxEnvironment` to false before starting building. To debug locally, set it to true. Ignore this when the `notifyWhenAppRunningInBackgroundOrQuit` is false. |
androidNotificationConfig | ZegoAndroidNotificationConfig? | No | This property needs to be set when you are building an Android app and when the `notifyWhenAppRunningInBackgroundOrQuit` is true. `androidNotificationConfig.channelID` must be the same as the FCM Channel ID in [ZEGOCLOUD Admin Console](https://console.zegocloud.com), and the `androidNotificationConfig.channelName` can be an arbitrary value. |
innerText | ZegoCallInvitationInnerText | No | To modify the UI text, use this property. For more details, see [Custom prebuilt UI](https://docs.zegocloud.com/article/14748). |
For more parameters, go to Custom prebuilt UI.
@override
Widget build(BuildContext context) {
return ZegoUIKitPrebuiltCallWithInvitation(
appID: yourAppID,
serverSecret: yourServerSecret,
appSign: yourAppSign,
userID: userID,
userName: userName,
plugins: [ZegoUIKitSignalingPlugin()],
config: ZegoUIKitPrebuiltCallInvitationConfig(
/// For offline call notification
notifyWhenAppRunningInBackgroundOrQuit: true,
/// For publish your app to TestFlight or App Store if you support offline call
isIOSSandboxEnvironment: false,
),
child: YourWidget(),
);
}
- Add the button for making call invitations, and pass in the ID of the user you want to call.
Props of ZegoSendCallInvitationButton
Property | Type | Required | Description |
---|---|---|---|
invitees | List< ZegoUIKitUser > | Yes | The information of the callee. userID and userName are required. For example: [{ userID: inviteeID, userName: inviteeName }] |
isVideoCall | bool | Yes | If true, a video call is made when the button is pressed. Otherwise, a voice call is made. |
resourceID | String? | No | `resourceID` can be used to specify the ringtone of an offline call invitation, which must be set to the same value as the Push Resource ID in [ZEGOCLOUD Admin Console](https://console.zegocloud.com). This only takes effect when the `notifyWhenAppRunningInBackgroundOrQuit` is true. |
timeoutSeconds | int | No | The timeout duration. It's 60 seconds by default. |
For more parameters, go to Custom prebuilt UI.
ZegoSendCallInvitationButton(
/// For offline call notification
resourceID: "zegouikit_call",
isVideoCall: true,
invitees: [
ZegoUIKitUser(
id: targetUserID,
name: targetUserName,
),
...
ZegoUIKitUser(
id: targetUserID,
name: targetUserName,
)
],
)
Now, you can make call invitations by simply clicking on this button.
Configure your project #
- Android
-
If your project is created with Flutter 2.x.x, you will need to open the
your_project/android/app/build.gradle
file, and modify thecompileSdkVersion
to 33. -
Add app permissions.
Open the file your_project/app/src/main/AndroidManifest.xml
, and add the following code:
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
<uses-permission android:name="android.permission.RECORD_AUDIO" />
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.MODIFY_AUDIO_SETTINGS" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_PHONE_STATE" />
<uses-permission android:name="android.permission.WAKE_LOCK" />
<!-- only invitation -->
<uses-permission android:name="android.permission.VIBRATE"/>
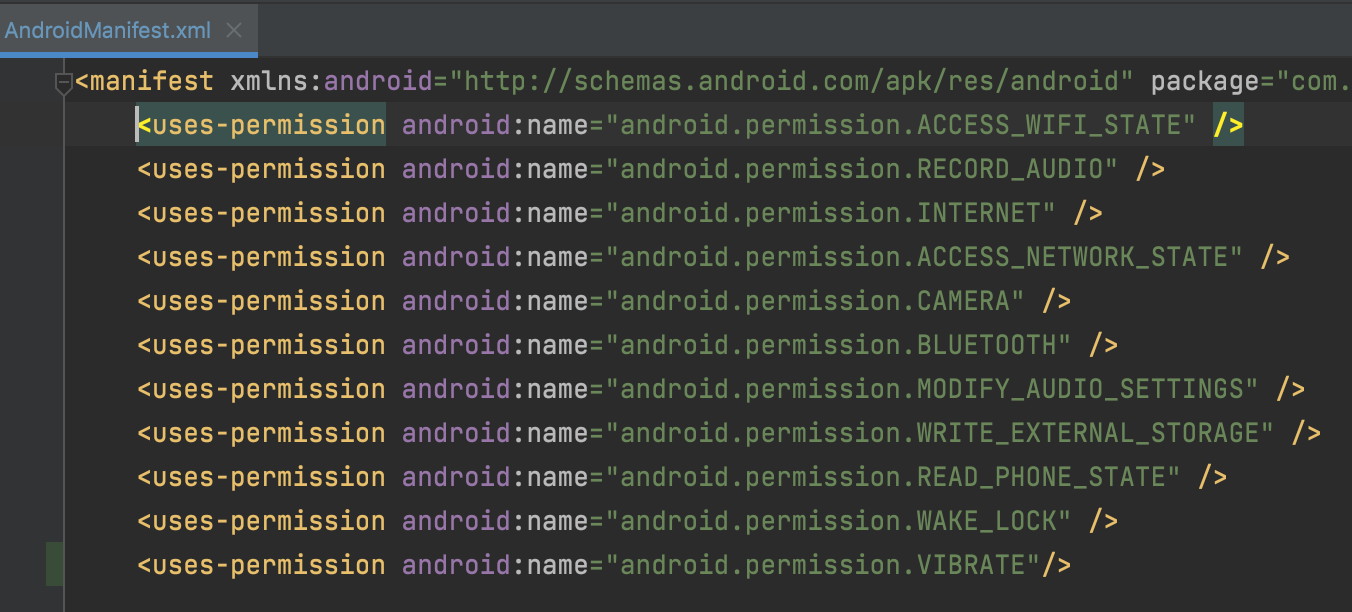
- Prevent code obfuscation.
To prevent obfuscation of the SDK public class names, do the following:
a. In your project's your_project > android > app
folder, create a proguard-rules.pro
file with the following content as shown below:
-keep class **.zego.** { *; }
-keep class **.**.zego_zpns.** { *; }
b. Add the following config code to the release
part of the your_project/android/app/build.gradle
file.
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
- iOS
- Need add app permissions, open ·your_project/ios/Runner/Info.plist·, add the following code inside the "dict" tag:
<key>NSCameraUsageDescription</key>
<string>We require camera access to connect to a call</string>
<key>NSMicrophoneUsageDescription</key>
<string>We require microphone access to connect to a call</string>
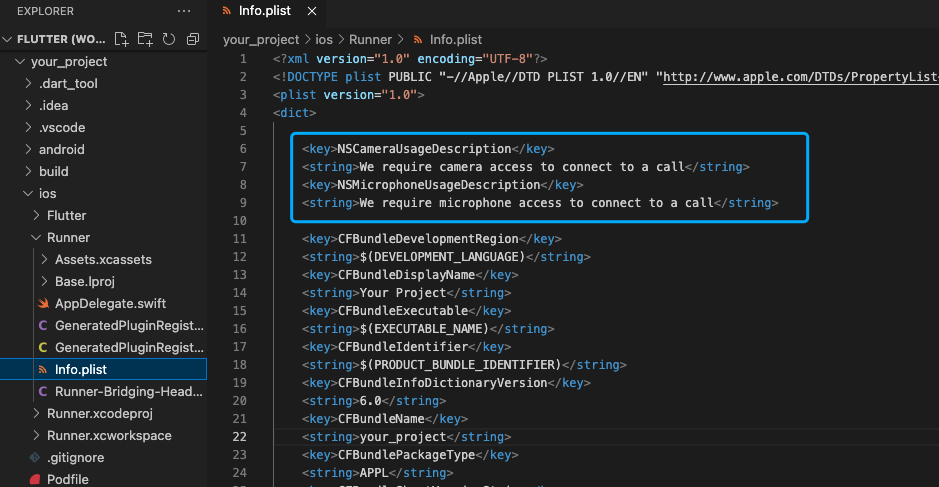
- To use the
notifications
and build your app correctly, navigate to the Build Settings tab, and set the following build options for your target app.
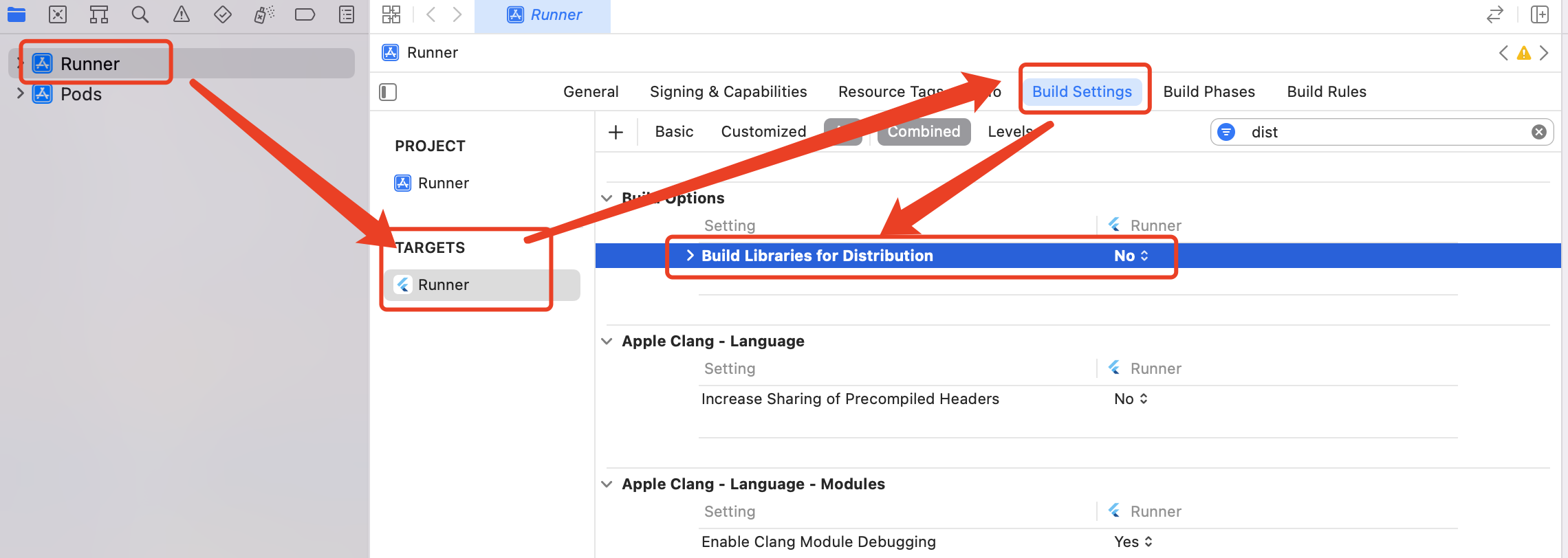
Refer to and set the following build options:
-
In the Runner Target:
a. Build Libraries for Distribution ->
NO
b. Only safe API extensions ->
NO
c. iOS Deployment Target ->
11 or greater
-
In other Targets:
a. Build Libraries for Distribution ->
NO
b. Only safe API extensions ->
YES
Enable offline call invitation #
If you want to receive call invitation notifications, do the following:
-
Click the button below to contact ZEGOCLOUD Technical Support.
-
Then, follow the instructions in the video below.
- iOS:
Resource may help: Apple Developer
- Android:
- Add this line to your project's
my_project/android/app/build.gradle
file as instructed.
implementation 'com.google.firebase:firebase-messaging:21.1.0'
- In your project's
/app/src/main/res/raw
directory, create akeep.xml
file with the following contents:
<?xml version="1.0" encoding="utf-8"?>
<resources xmlns:tools="http://schemas.android.com/tools"
tools:keep="@raw/*">
</resources>
Resource may help: Firebase Console
Run & Test #
Now you have finished all the steps!
You can simply click the Run or Debug to run and test your App on your device.
Related guide #
Resources #
Click to get the complete sample code.