smooth_corner 1.1.0
smooth_corner: ^1.1.0 copied to clipboard
A rectangular border with variable smoothness imitated from Figma.
smooth_corner #
The smooth rounded corners of iOS are implemented in Flutter, imitating Figma's "corner smoothing" function. The algorithm also comes from Figma's blog "Desperately seeking squircles".
The principle is spliced by two segments of Bezier curve and one segment of arc, which is the same as Figma, with variable smoothness. When the parameter smoothness is 0, it is a normal rounded rectangle. When the parameter is 1, the rounded corners are only It is composed of two Bezier curves and is close to a hyperellipse.
You can view the Debug rounded corner widget and picture examples that I wrote in the sample project, adjust the smoothness and rounded corner size, and you can see the changes in the rounded corner curve.
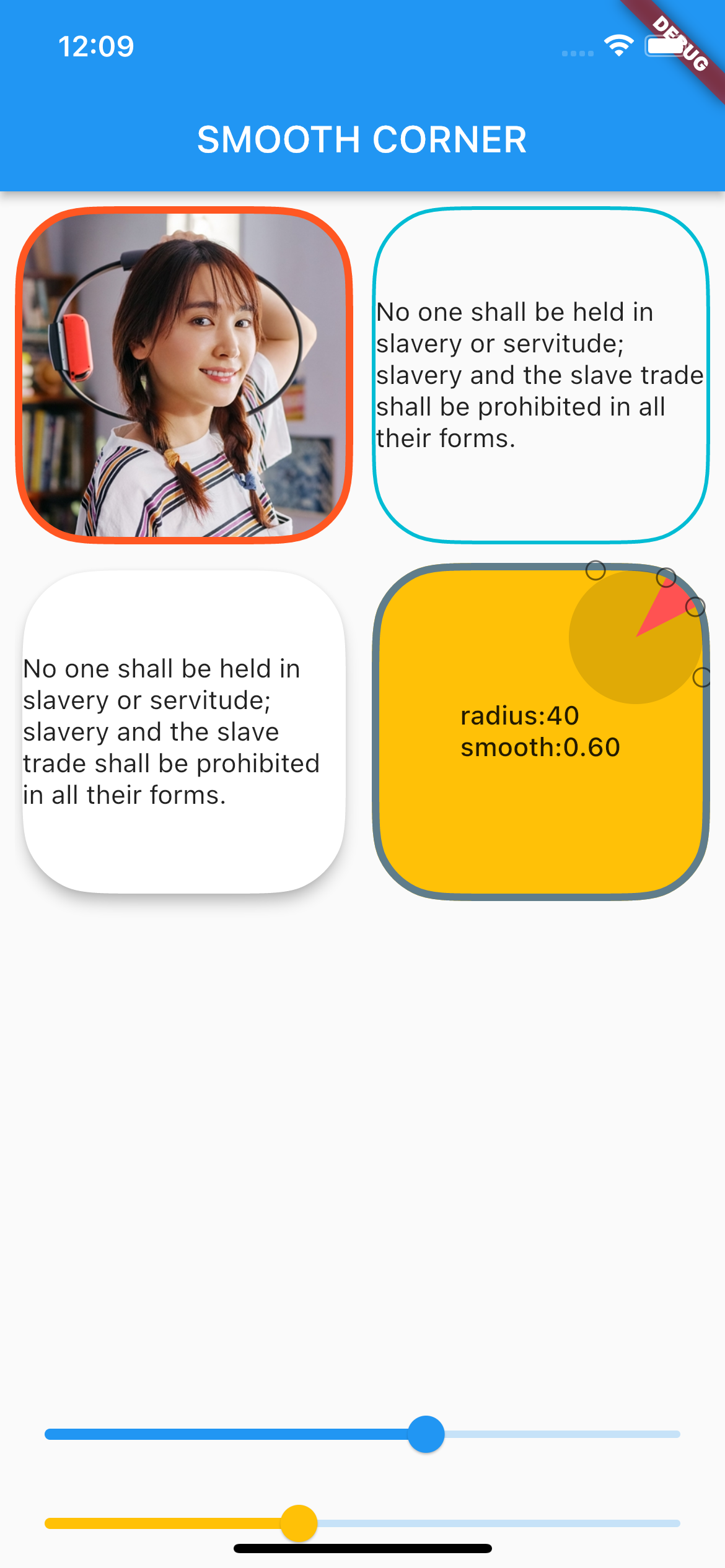
Usage #
Add dependency #
dependencies:
smooth_corner: ^1.0.0
Built-in Widgets #
The library provides several commonly used widgets that can adjust smooth rounded corners.
These widgets provide three additional parameters "smoothness, side, borderRadius" to control smooth rounded corners and borders.
smoothness
represents smoothness, the range is [0, 1.0]. According to Figma's standard, the rounded curve closest to iOS when the smoothness is equal to 0.6.
The type of side
is BorderSide
, which is used to set the border.
borderRadius
is used to set the corner radius. Note that if the x and y values of the radius are not equal, the smallest value will be selected.
SmoothContainer
SmoothContainer
contains all the parameters of Container
.
SmoothContainer(
width: 200,
height: 200,
smoothness: 0.6,
side: BorderSide(color: Colors.cyan, width: 2),
borderRadius: BorderRadius.circular(40),
child: child,
alignment: Alignment.center,
),
SmoothClipRRect
In order to achieve that the image can also add a border, SmoothClipRRect
is provided.
SmoothClipRRect(
smoothness: 0.6,
side: BorderSide(color: Colors.deepOrange, width: 4),
borderRadius: BorderRadius.circular(40),
child: Image.network(
url,
fit: BoxFit.cover,
),
SmoothCard
SmoothCard
contains all the parameters of Card
SmoothCard(
smoothness: 0.6,
borderRadius: BorderRadius.circular(40),
elevation: 6,
child: child,
),
Custom widget #
The smooth rounding capabilities of the above widgets are all implemented by SmoothRectangleBorder
, you can use this ShapeBorder to customize your smooth rounded widgets freely.
ShapeDecoration
Container(
width: 200,
height: 200,
alignment: Alignment.center,
decoration: ShapeDecoration(
shape: SmoothRectangleBorder(
borderRadius: BorderRadius.circular(40),
smoothness: 0.6,
),
color: Colors.amber,
),
child: Text(''),
),
ShapeBorderClipper
Container(
width: 200,
height: 200,
child: ClipPath(
clipper: ShapeBorderClipper(
shape: SmoothRectangleBorder(
smoothness: 0.6,
borderRadius:
BorderRadius.circular(40),
),
),
child: Image.network(url),
),
),