smart_auth 3.2.0
smart_auth: ^3.2.0 copied to clipboard
Wrapper of Android SMS User Consent API, SMS Retriever API to read one time sms code, get user phone number, OTP, OTC, sms autofill, android autofill
Flutter package for listening SMS code on Android, suggesting phone number, email, saving a credential.
If you need pin code input like shown below, take a look at the Pinput package.
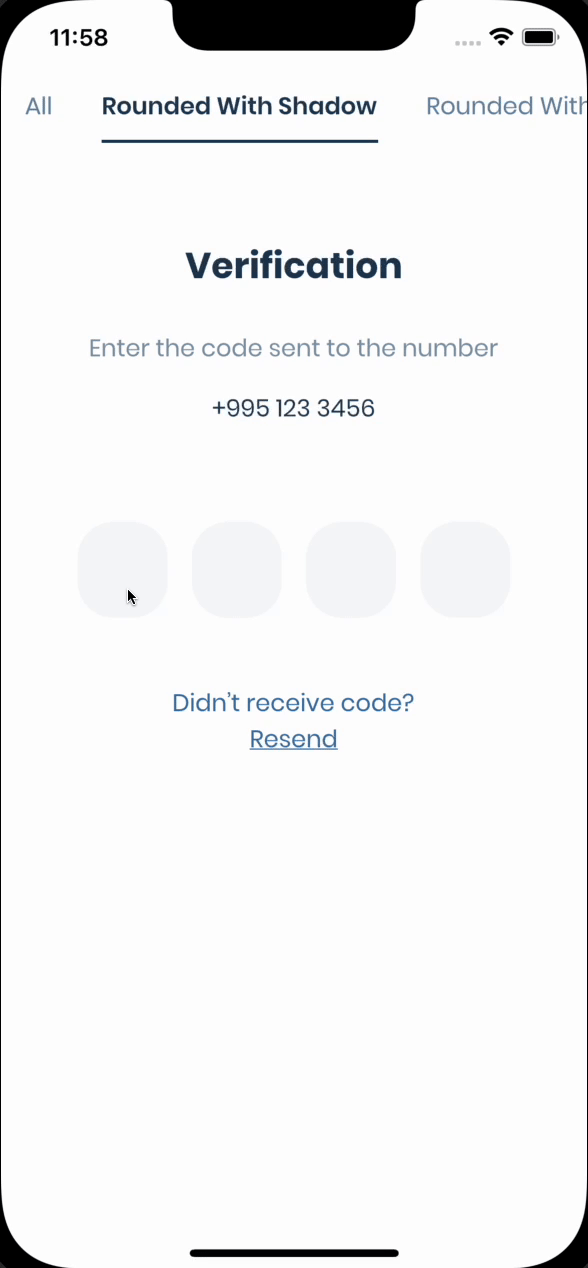
Features: #
Support #
Discord Channel
Don't forget to give it a star ⭐
If you want to contribute to this project, please read the contribution guide.
Requirements #
1. Set kotlin version to 1.8.0 or above and gradle plugin version to 8.3.2
If you are using legacy imperative apply
// android/build.gradle
buildscript {
ext.kotlin_version = '1.8.0'
...others
dependencies {
classpath 'com.android.tools.build:gradle:8.3.2'
classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version"
}
}
If you are using new declarative plugin approach
// android/settings.gradle
plugins {
id "org.jetbrains.kotlin.android" version "1.8.0" apply false
id "com.android.application" version "8.3.2" apply false
...others
}
2. Set gradle version to 8.4.0 or above - more about gradle versions
// android/gradle/wrapper/gradle-wrapper.properties
distributionUrl=https\://services.gradle.org/distributions/gradle-8.4-all.zip
3. Set Java version to 11
// android/app/build.gradle
compileOptions {
sourceCompatibility = JavaVersion.VERSION_11
targetCompatibility = JavaVersion.VERSION_11
}
kotlinOptions {
jvmTarget = '11'
}
Getting Started #
Create instance of SmartAuth #
final smartAuth = SmartAuth.instance;
Request phone number hint - Docs #
The Phone Number Hint API, a library powered by Google Play services, provides a frictionless way to show a user’s (SIM-based) phone numbers as a hint.
The benefits to using Phone Number Hint include the following:
- No additional permission requests are needed
- Eliminates the need for the user to manually type in the phone number
- No Google account is needed
- Not directly tied to sign in/up workflows
- Wider support for Android versions compared to Autofill
void requestPhoneNumberHint() async {
final res = await smartAuth.requestPhoneNumberHint();
if (res.hasData) {
// Use the phone number
} else {
// Handle error
}
}
Get SMS with User Consent API #
The SMS User Consent API complements the SMS Retriever API by allowing an app to prompt the user to grant access to the content of a single SMS message. When a user gives consent, the app will then have access to the entire message body to automatically complete SMS verification. The verification flow looks like this:
- A user initiates SMS verification in your app. Your app might prompt the user to provide a phone
number manually or request the phone number hint by calling
requestPhoneNumberHint
method. - Your app makes a request to your server to verify the user's phone number. Depending on what information is available in your user database, this request might include the user's ID, the user's phone number, or both.
- At the same time, your app calls the
getSmsWithUserConsentApi
to show the user a dialog to grant access to the SMS message. - Your server sends an SMS message to the user that includes a one-time code to be sent back to your server.
- When the user's device receives the SMS message, the
getSmsWithUserConsentApi
will extract the one-time code from the message text and you have to send it back to your server. - Your server receives the one-time code from your app, verifies the code, and finally records that the user has successfully verified their account.
void getSmsWithUserConsentApi() async {
final res = await smartAuth.getSmsWithUserConsentApi();
if (res.hasData) {
final code = res.requireData.code;
/// The code can be null if the SMS was received but the code was not extracted from it
if (code == null) return;
// Use the code
} else if (res.isCanceled) {
// User canceled the dialog
} else {
// handle the error
}
}
Get SMS with SMS Retriever API #
With the SMS Retriever API, you can perform SMS-based user verification in your Android app automatically, without requiring the user to manually type verification codes, and without requiring any extra app permissions. When you implement automatic SMS verification in your app, the verification flow looks like this:
- A user initiates SMS verification in your app. Your app might prompt the user to provide a phone
number manually or request the phone number hint by calling
requestPhoneNumberHint
method. - Your app makes a request to your server to verify the user's phone number. Depending on what information is available in your user database, this request might include the user's ID, the user's phone number, or both.
- At the same time, your app calls the
getSmsWithRetrieverApi
to begin listening for an SMS response from your server. - Your server sends an SMS message to the user that includes a one-time code to be sent back to your server, and a hash that identifies your app.
- When the user's device receives the SMS message, Google Play services uses the app hash to determine that the message is intended for your app, and makes the message text available to your app through the SMS Retriever API.
- The
getSmsWithRetrieverApi
will extract the one-time code from the message text and you have to send it back to your server. - Your server receives the one-time code from your app, verifies the code, and finally records that the user has successfully verified their account.
void getSmsWithRetrieverApi() async {
final res = await smartAuth.getSmsWithRetrieverApi();
if (res.hasData) {
final code = res.requireData.code;
/// The code can be null if the SMS was received but the code was not extracted from it
if (code == null) return;
// Use the code
} else {
// handle the error
}
}
Dispose #
The plugin automatically removes listeners after receiving the code, if not you can remove them by
calling the removeUserConsentApiListener
or removeSmsRetrieverApiListener
method.
void removeSmsListener() {
smartAuth.removeUserConsentApiListener();
// or
smartAuth.removeSmsRetrieverApiListener();
}