open_with 0.1.3
open_with: ^0.1.3 copied to clipboard
Open any File in Flutter app using Open With option
Open With #
This package allows to open any file in your flutter application by using open with option. #
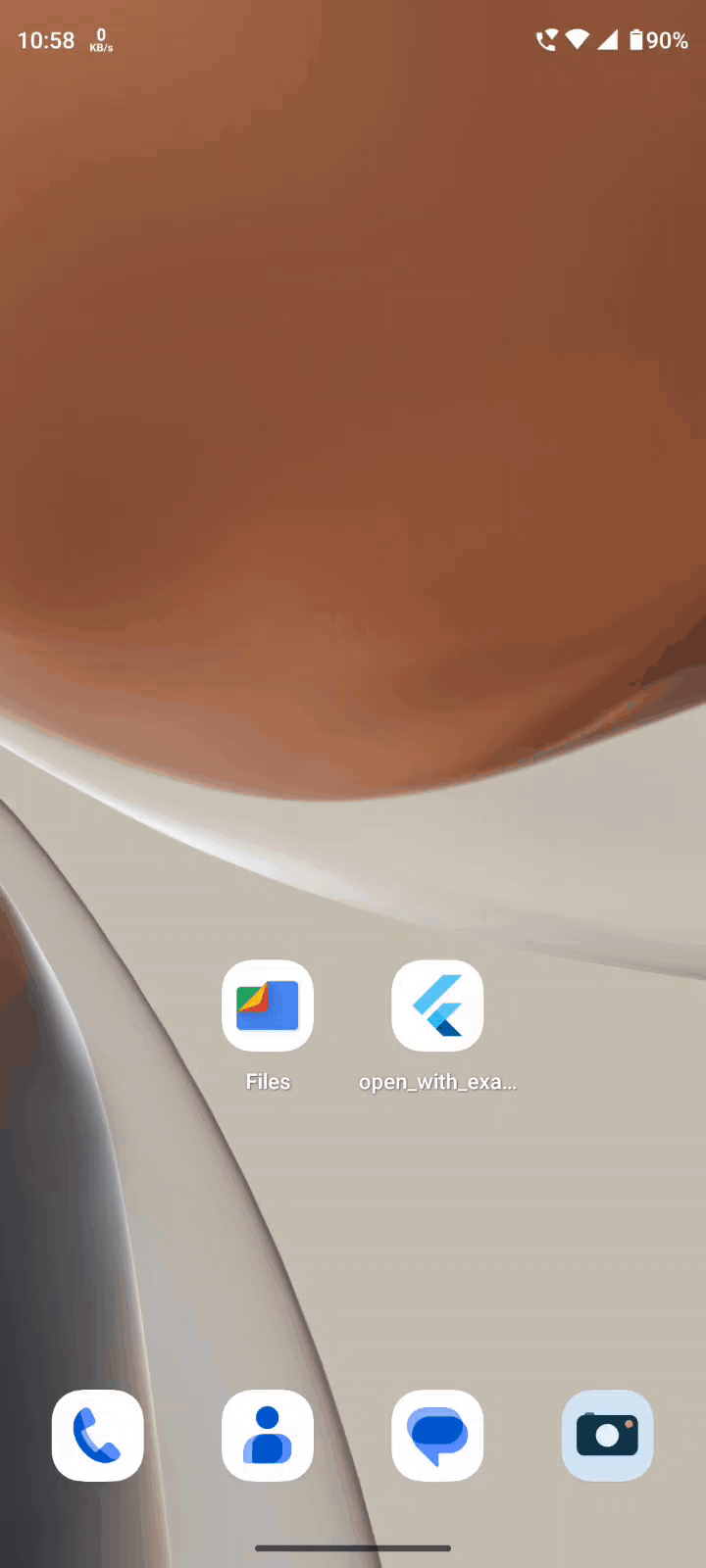
How to Use #
Add in your android\app\src\main\AndroidManifest.xml #
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.BROWSABLE" />
<category android:name="android.intent.category.DEFAULT" />
<data android:scheme="content" />
<data android:scheme="file" />
<data android:mimeType="application/pdf" />
</intent-filter>
Like this
<application
<activity
<intent-filter>
<action android:name="android.intent.action.MAIN"/>
<category android:name="android.intent.category.LAUNCHER"/>
</intent-filter>
-------------------------- Add intent-filter -------------------
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.BROWSABLE" />
<category android:name="android.intent.category.DEFAULT" />
<data android:scheme="content" />
<data android:scheme="file" />
<data android:mimeType="application/pdf" />
</intent-filter>
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.BROWSABLE" />
<category android:name="android.intent.category.DEFAULT" />
<data android:scheme="content" />
<data android:scheme="file" />
<data android:mimeType="application/msword" />
</intent-filter>
------------------------------------------------------------------
</activity>
</application>
After add code in AndroidManifest.xml uninstall your app and run again.
For Open Diffrent Files #
Change The android:mimeType="" according to the file Type.
Find Diffrent android:mimeType
link 1 : click here
link 2 : click here
main.dart #
import 'package:flutter/material.dart';
import 'package:open_with/open_with.dart';
void main() async {
WidgetsFlutterBinding.ensureInitialized();
IntentDetails? intent = await getIntent();
if (intent != null) {
return runApp(
MaterialApp(
home: IntentPage(intentDetails: intent),
),
);
} else {
return runApp(
const MaterialApp(home: HomePage()),
);
}
}
intent_page.dart #
import 'package:open_with/open_with.dart';
class IntentPage extends StatefulWidget {
IntentDetails intentDetails;
IntentPage({Key? key, required this.intentDetails}) : super(key: key);
@override
State<IntentPage> createState() => _IntentPageState();
}
class _IntentPageState extends State<IntentPage> {
late IntentDetails intentDetails;
@override
void initState() {
super.initState();
intentDetails = widget.intentDetails;
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Padding(
padding: const EdgeInsets.all(10),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
mainAxisSize: MainAxisSize.max,
children: [
const Text("DO Something Using intent Details"),
Text("\nName : ${intentDetails.name}"),
Text("Type : ${intentDetails.type}"),
Text("Size : ${(intentDetails.size)}"),
Text("lastModifiedDate : ${(intentDetails.lastModifiedDate)}"),
Text("Path : ${(intentDetails.path)}"),
],
),
),
);
}
}
home_page.dart #
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Text("Home Page", style: Theme.of(context).textTheme.titleLarge),
),
);
}
}