infinite_scroll_pagination 2.1.0+1
infinite_scroll_pagination: ^2.1.0+1 copied to clipboard
Load and display pages of items as the user scrolls down your screen.
Infinite Scroll Pagination #
Unopinionated, extensible and highly customizable package to help you lazily load and display small chunks of data as the user scrolls down the screen – known as infinite scrolling pagination, endless scrolling pagination, auto-pagination, lazy loading pagination, progressive loading pagination, etc.
Designed to feel like part of the own Flutter framework.
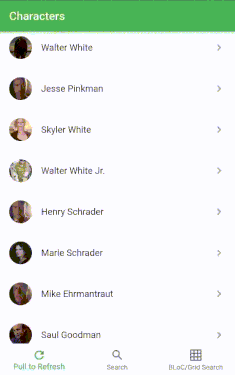
Tutorial #
By raywenderlich.com (step-by-step, hands-on, in-depth and illustrated).
Usage #
class CharacterListView extends StatefulWidget {
@override
_CharacterListViewState createState() => _CharacterListViewState();
}
class _CharacterListViewState extends State<CharacterListView> {
static const _pageSize = 20;
final PagingController<int, CharacterSummary> _pagingController =
PagingController(firstPageKey: 0);
@override
void initState() {
_pagingController.addPageRequestListener((pageKey) {
_fetchPage(pageKey);
});
super.initState();
}
Future<void> _fetchPage(int pageKey) async {
try {
final newItems = await RemoteApi.getCharacterList(pageKey, _pageSize);
final isLastPage = newItems.length < _pageSize;
if (isLastPage) {
_pagingController.appendLastPage(newItems);
} else {
final nextPageKey = pageKey + newItems.length;
_pagingController.appendPage(newItems, nextPageKey);
}
} catch (error) {
_pagingController.error = error;
}
}
@override
Widget build(BuildContext context) =>
// Don't worry about displaying progress or error indicators on screen; the
// package takes care of that. If you want to customize them, use the
// [PagedChildBuilderDelegate] properties.
PagedListView<int, CharacterSummary>(
pagingController: _pagingController,
builderDelegate: PagedChildBuilderDelegate<CharacterSummary>(
itemBuilder: (context, item, index) => CharacterListItem(
character: item,
),
),
);
@override
void dispose() {
_pagingController.dispose();
super.dispose();
}
}
For more usage examples, please take a look at our cookbook or check out the example project.
Features #
-
Architecture-agnostic: Works with any state management approach, from setState to BLoC. Not even Future usage is assumed.
-
Layout-agnostic: Out-of-the-box widgets corresponding to GridView, SliverGrid, ListView and SliverList – including
.separated
constructors. Not enough? You can easily create a custom layout. -
API-agnostic: By letting you in complete charge of your API calls, Infinite Scroll Pagination works with any pagination strategy.
-
Highly customizable: You can change everything. Provide your own progress, error and empty list indicators. Too lazy to change? The defaults will cover you.
-
Extensible: Seamless integration with pull-to-refresh, searching, filtering and sorting.
-
Listen to state changes: In addition to displaying widgets to inform the current status, such as progress and error indicators, you can also use a listener to display dialogs/snackbars/toasts or execute any other action.
API Overview #
Motivation #
Flutter indeed makes our job way easier than other toolkits when talking about infinite scrolling. It's when we combine that with automated pagination and status indicators that things get complicated.
ListView.builder builds your items on demand, but it doesn't help you with fetching them or displaying status indicators.
Your listing will go through several stages: first page loading, first page error, subsequent page loading, subsequent page error, empty list and completed list. Infinite Scroll Pagination takes care of orchestrating between them, rendering each one and letting you know when more data is needed.