google_fonts 6.2.1
google_fonts: ^6.2.1 copied to clipboard
A Flutter package to use fonts from fonts.google.com. Supports HTTP fetching, caching, and asset bundling.
google_fonts #
A Flutter package to use fonts from fonts.google.com.
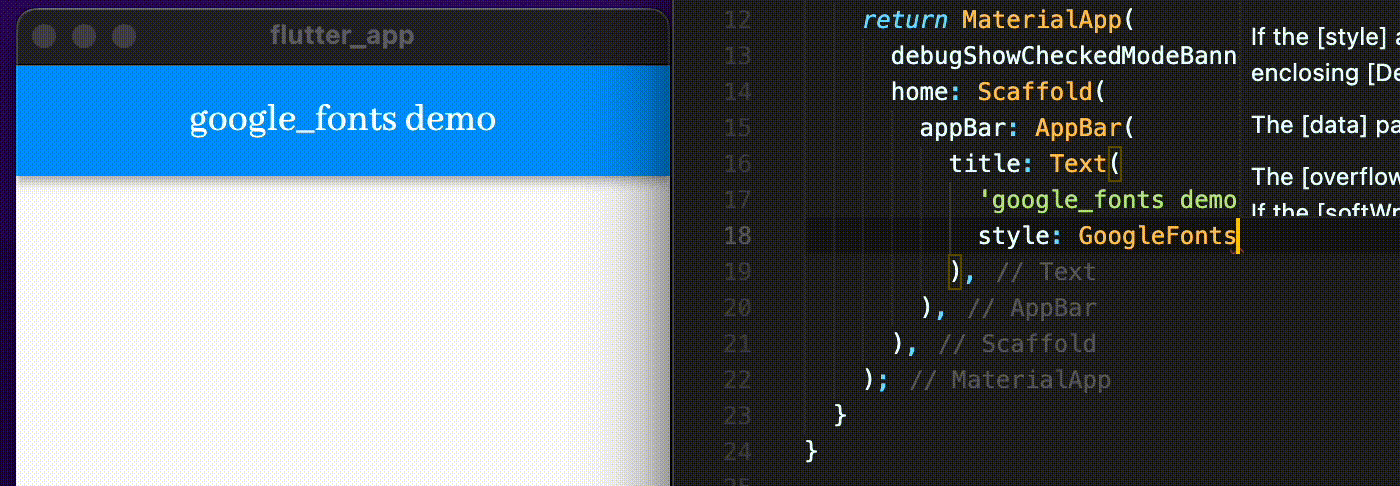
Features #
- HTTP fetching at runtime, ideal for development. Can also be used in production to reduce app size
- Font file caching, on device file system
- Font bundling in assets. Matching font files found in assets are prioritized over HTTP fetching. Useful for offline-first apps.
Usage #
For example, say you want to use the Lato font from Google Fonts in your Flutter app.
-
Add the
google_fonts
package to your pubspec dependencies. -
Import
GoogleFonts
.import 'package:google_fonts/google_fonts.dart';
copied to clipboard
Text styles #
To use GoogleFonts
with the default TextStyle
:
Text(
'This is Google Fonts',
style: GoogleFonts.lato(),
),
Or, if you want to load the font dynamically:
Text(
'This is Google Fonts',
style: GoogleFonts.getFont('Lato'),
),
To use GoogleFonts
with an existing TextStyle
:
Text(
'This is Google Fonts',
style: GoogleFonts.lato(
textStyle: TextStyle(color: Colors.blue, letterSpacing: .5),
),
),
or
Text(
'This is Google Fonts',
style: GoogleFonts.lato(textStyle: Theme.of(context).textTheme.headline4),
),
To override the fontSize
, fontWeight
, or fontStyle
:
Text(
'This is Google Fonts',
style: GoogleFonts.lato(
textStyle: Theme.of(context).textTheme.displayLarge,
fontSize: 48,
fontWeight: FontWeight.w700,
fontStyle: FontStyle.italic,
),
),
Text themes #
You can also use GoogleFonts.latoTextTheme()
to make or modify an entire text theme to use the "Lato" font.
...
return MaterialApp(
theme: _buildTheme(Brightness.dark),
);
}
ThemeData _buildTheme(brightness) {
var baseTheme = ThemeData(brightness: brightness);
return baseTheme.copyWith(
textTheme: GoogleFonts.latoTextTheme(baseTheme.textTheme),
);
}
Or, if you want a TextTheme
where a couple of styles should use a different font:
final textTheme = Theme.of(context).textTheme;
MaterialApp(
theme: ThemeData(
textTheme: GoogleFonts.latoTextTheme(textTheme).copyWith(
bodyMedium: GoogleFonts.oswald(textStyle: textTheme.bodyMedium),
),
),
);
Visual font swapping #
To avoid visual font swaps that occur when a font is loading, use FutureBuilder and GoogleFonts.pendingFonts().
See the example app.
HTTP fetching #
For HTTP fetching to work, certain platforms require additional steps when running the app in debug and/or release mode. For example, macOS requires the following be present in the relevant .entitlements file:
<key>com.apple.security.network.client</key>
<true/>
Learn more at https://docs.flutter.dev/development/data-and-backend/networking#platform-notes.
Bundling fonts when releasing #
The google_fonts
package will automatically use matching font files in your pubspec.yaml
's
assets
(rather than fetching them at runtime via HTTP). Once you've settled on the fonts
you want to use:
- Download the font files from https://fonts.google.com.
You only need to download the weights and styles you are using for any given family.
Italic styles will include
Italic
in the filename. Font weights map to file names as follows:
{
FontWeight.w100: 'Thin',
FontWeight.w200: 'ExtraLight',
FontWeight.w300: 'Light',
FontWeight.w400: 'Regular',
FontWeight.w500: 'Medium',
FontWeight.w600: 'SemiBold',
FontWeight.w700: 'Bold',
FontWeight.w800: 'ExtraBold',
FontWeight.w900: 'Black',
}
- Move those fonts to some asset folder (e.g.
google_fonts
). You can name this folder whatever you like and use subdirectories.
- Ensure that you have listed the asset folder (e.g.
google_fonts/
) in yourpubspec.yaml
, underassets
.
Note: Since these files are listed as assets, there is no need to list them in the fonts
section
of the pubspec.yaml
. This can be done because the files are consistently named from the Google Fonts API
(so be sure not to rename them!)
See the API docs to completely disable HTTP fetching.
Licensing Fonts #
The fonts on fonts.google.com include license files for each font. For
example, the Lato font comes with an OFL.txt
file.
Once you've decided on the fonts you want in your published app, you should add the appropriate licenses to your flutter app's LicenseRegistry.
For example:
void main() {
LicenseRegistry.addLicense(() async* {
final license = await rootBundle.loadString('google_fonts/OFL.txt');
yield LicenseEntryWithLineBreaks(['google_fonts'], license);
});
runApp(...);
}
Testing #
See example/test for testing examples.