flutter_context_menu 0.1.3
flutter_context_menu: ^0.1.3 copied to clipboard
Create and display a customizable context menus in your app.
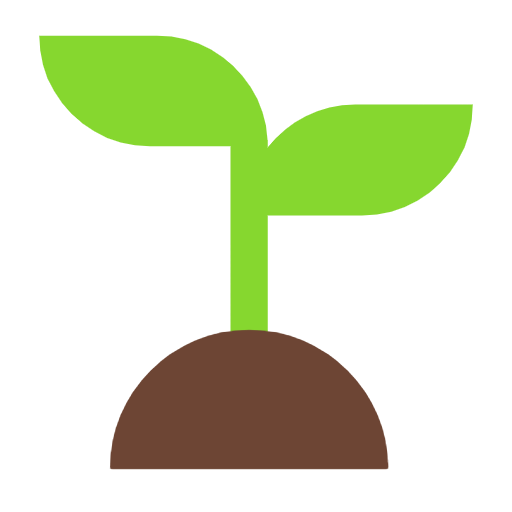
Flutter Context Menu
A Flutter library that provides a flexible and customizable solution for creating and displaying context menus in Flutter applications. It allows you to easily add context menus to your UI, providing users with a convenient way to access additional options and actions specific to the selected item or area.
View Example ยท Report Bug ยท Request Feature
Features #
-
ContextMenu
: The package includes a highly customizable context menu system that can be easily integrated into your Flutter application. It provides a seamless and intuitive user experience, enhancing the usability of your app. -
Hierarchical Structure: The context menu supports a hierarchical structure with submenu functionality. This enables you to create nested menus, providing a clear and organized representation of options and suboptions.
-
Selection Handling: The package includes built-in selection handling for context menu items. It allows you to define callback functions for individual menu items, enabling you to execute specific actions or logic when an item is selected.
-
Customization Options: Customize the appearance and behavior of the context menu to match your app's design and requirements. Modify the style, positioning, animation, and interaction of the menu to create a cohesive user interface.
-
Built-in Components: The package includes built-in components, such as
MenuItem
,MenuDivider
, andMenuHeader
, that can be used in your context menu. -
Cross Platform Support: The package is compatible with multiple platforms, including Android, iOS, Web, and Desktop.
Getting Started #
Installation #
-
Method 1 (Recommended):
run this command in your terminal:
flutter pub add flutter_context_menu
-
Method 2:
add this line to your
pubspec.yaml
dependencies:dependencies: flutter_context_menu: ^0.1.3
then, run this command in your terminal:
flutter pub get
Usage #
-
First, import the package:
import 'package:flutter_context_menu/flutter_context_menu.dart';
-
Then, initialize a
ContextMenu
instance:// define your context menu entries final entries = <ContextMenuEntry>[ const MenuHeader(text: "Context Menu"), MenuItem( label: 'Copy', icon: Icons.copy, onSelected: () { // implement copy }, ), MenuItem( label: 'Paste', icon: Icons.paste, onSelected: () { // implement paste }, ), const MenuDivider(), MenuItem.submenu( label: 'Edit', icon: Icons.edit, items: [ MenuItem( label: 'Undo', value: "Undo", icon: Icons.undo, onSelected: () { // implement undo }, ), MenuItem( label: 'Redo', value: 'Redo', icon: Icons.redo, onSelected: () { // implement redo }, ), ], ), ]; // initialize a context menu final contextMenu = ContextMenu( entries: entries, position: const Offset(300, 300), padding: const EdgeInsets.all(8.0), );
-
Finally, to show the context menu, there are two ways:
-
Method 1: Directly calling one of the show methods.
This will show the context menu at the manually specified position.
showContextMenu(context, contextMenu: myContextMenu); // or final selectedValue = await myContextMenu.show(context);
-
Method 2: Using the
ContextMenuRegion
widget to show the context menu when the user right-clicks or long-presses the region area.This will show the context menu where the user clicks when the
position
property is not specified in theContextMenu
constructor.... @override Widget build(BuildContext context) { return Column( children: [ ContextMenuRegion( contextMenu: contextMenu, onItemSelected: (value) { print(value); }, child: Container( color: Colors.indigo, height: 300, width: 300, child: const Center( child: Text( 'Right click or long press!', ), ), ), ) ], ); }
-
Customization #
Theme: By default, the context menu and its items uses the
MaterialApp
's theme data for styling. However, you can customize the appearance and behavior of the context menu by modifying the theme data. Or individually by specifying aBoxDecoration
in theboxDecoration
property of theContextMenu
.
Custom Entries: You can create your own context menu entries by subclassing the
ContextMenuEntry
class. This allows you to customize the appearance, behavior, and functionality of the context menu items.
Learn More #
- More info: Full documentation will be provided, inshallah ๐
- See: Full Example
Feedback and Contributions #
If you have any suggestions or feedback, please open an issue or create a pull request.
If you like this package, please star it and follow me on Twitter and GitHub.
License #
This project is licensed under the BSD 3-Clause License.
Made with โค๏ธ in Egypt ๐ช๐ฌ by Salah Rashad #FreePalestine ๐ต๐ธ