event_bus_plus 0.7.0
event_bus_plus: ^0.7.0 copied to clipboard
Event Bus for Dart.
EventBus: Events for Dart/Flutter #
EventBus is an open-source library for Dart and Flutter using the publisher/subscriber pattern for loose coupling. EventBus enables central communication to decoupled classes with just a few lines of code β simplifying the code, removing dependencies, and speeding up app development.
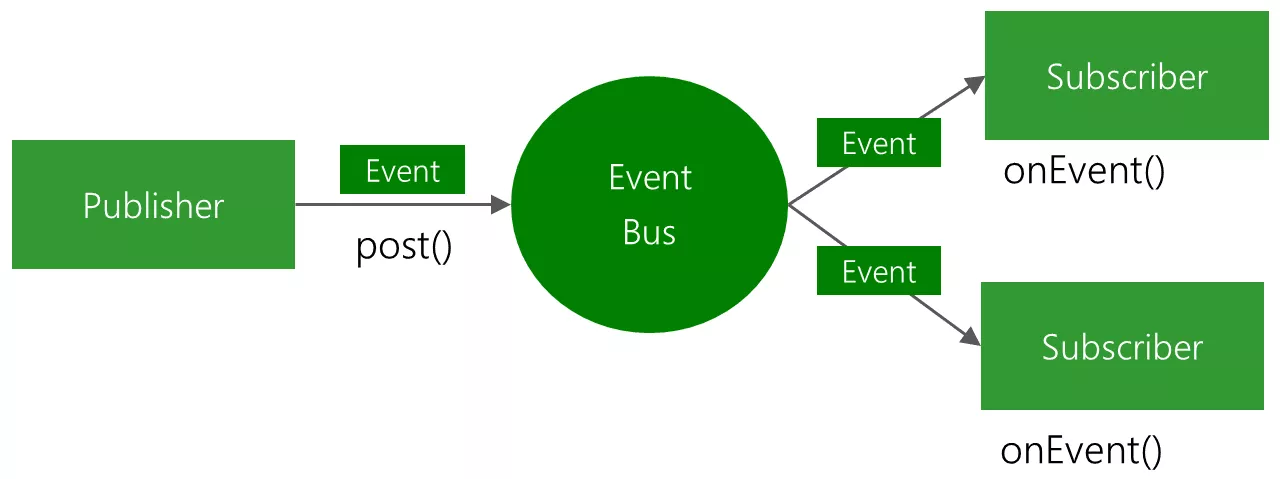
Your benefits using EventBus: It⦠#
- simplifies the communication between components;
- decouples event senders and receivers;
- performs well with UI artifacts (e.g. Widgets, Controllers);
- avoids complex and error-prone dependencies and life cycle issues.
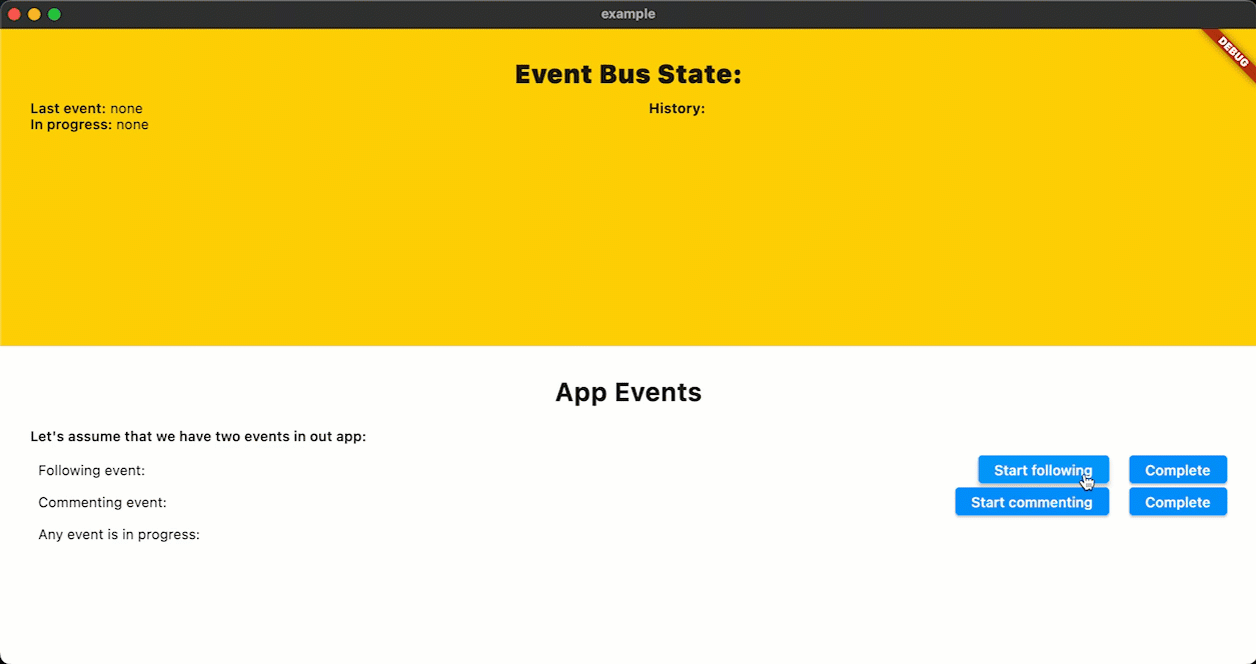
Define the app's events #
// Initialize the Service Bus
IAppEventBus eventBus = AppEventBus();
// Define your app events
final event = FollowEvent('@devcraft.ninja');
final event = CommentEvent('Awesome package π');
Subscribe #
// listen the latest event
final sub = eventBus.last$
.listen((AppEvent event) { /*do something*/ });
// Listen particular event
final sub2 = eventBus.on<FollowAppEvent>()
.listen((e) { /*do something*/ });
Publish #
// fire the event
eventBus.fire(event);
Watch events in progress #
// start watch the event till its completion
eventBus.watch(event);
// and check the progress
eventBus.isInProgress<FollowAppEvent>();
// or listen stream to check the processing
eventBus.inProgress$.map((List<AppEvent> events) =>
events.whereType<FollowAppEvent>().isNotEmpty);
// complete
_eventBus.complete(event);
// or complete with completion event
_eventBus.complete(event, nextEvent: SomeAnotherEvent);
History #
final events = eventBus.history;
Mapping #
final eventBus = bus = EventBus(
map: {
SomeEvent: [
(e) => SomeAnotherEvent(),
],
},
);
Contributing #
We accept the following contributions:
- Improving the documentation
- Reporting issues
- Fixing bugs