decoding_text_effect 1.1.1
decoding_text_effect: ^1.1.1 copied to clipboard
A Flutter package for DecodingTextEffect widget. There are 5 Decode Effects that you can choose from.

This flutter package contains a widget called DecodingTextEffect which is having some cool Decode Effects for texts.
Installing #
1. Depend on it #
Add this to your package's pubspec.yaml
file:
dependencies:
decoding_text_effect: ^1.0.0
2. Install it #
You can install packages from the command line:
$ flutter pub get
3. Import it #
Now in your Dart
code, you can use:
import 'package:decoding_text_effect/decoding_text_effect.dart';
Documentation #
Decoding effects occurs only for following two cases,
- When the widget is rendered for the very first time.
- When the value of
originalString
parameter gets changed.
DecodingTextEffect(
this.originalString, {
@required this.decodeEffect,
Key key,
this.textStyle,
this.textAlign,
this.refreshDuration,
this.eachCount = 5,
this.onTap,
this.onFinished,
}) : assert(
originalString != null,
'A non-null String must be provided to a Decoding Text Effect Widget.',
),
super(key: key);
Usage #
List<String> myText = [
'Decoding Text\nEffect',
'Welcome to\nthe Dart Side!',
'I have 50\nwatermelons',
'Quick Maths,\n2 + 2 = 4'
];
1. DecodeEffect.fromStart #

Container(
height: 200,
width: 350,
color: Colors.pink[100],
padding: EdgeInsets.all(50),
child: DecodingTextEffect(
myText[index],
decodeEffect: DecodeEffect.fromStart,
textStyle: TextStyle(fontSize: 30),
),
),
2. DecodeEffect.fromEnd #

Container(
height: 200,
width: 350,
color: Colors.yellow[100],
padding: EdgeInsets.all(50),
child: DecodingTextEffect(
myText[index],
decodeEffect: DecodeEffect.fromEnd,
textStyle: TextStyle(fontSize: 30),
),
),
3. DecodeEffect.toMiddle #

Container(
height: 200,
width: 350,
color: Colors.green[100],
padding: EdgeInsets.all(50),
child: DecodingTextEffect(
myText[index],
decodeEffect: DecodeEffect.to_middle,
textStyle: TextStyle(fontSize: 30),
),
),
4. DecodeEffect.random #
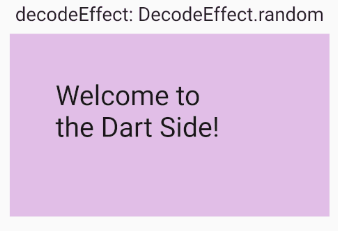
Container(
height: 200,
width: 350,
color: Colors.purple[100],
padding: EdgeInsets.all(50),
child: DecodingTextEffect(
myText[index],
decodeEffect: DecodeEffect.random,
textStyle: TextStyle(fontSize: 30),
),
),
5. DecodeEffect.all #

Container(
height: 200,
width: 350,
color: Colors.blue[100],
padding: EdgeInsets.all(50),
child: DecodingTextEffect(
myText[index],
decodeEffect: DecodeEffect.all,
textStyle: TextStyle(fontSize: 30),
),
),
refreshDuration and eachCount #
The refreshDuration
is an optional argument that is having a default value of Duration(milliseconds: 60)
.
Shorter the value of refreshDuration, faster will be the effect and hence decreasing the duration of effect. refreshDuration
is also the time gap between two consecutive setState()
function calls.
The eachCount
is also an optional argument that is having a default value of 5
. It is the number of random characters that will be shown before showing the original character and then moving on to decode next character and this cycles repeat until the completion of effect.
Demo #
Source code of the below app is available in the example directory of this package's github repository.

Below are some other demonstration of DecoratingTextEffect widget. But the source code of below apps are not in this repository.
![]() |
![]() |