date_form_field 0.0.2
date_form_field: ^0.0.2 copied to clipboard
DateFormField wraps a TextField and integrates it with the enclosing Form. This provides additional functionality, such as validation and integration with other FormField widgets.
date_form_field #
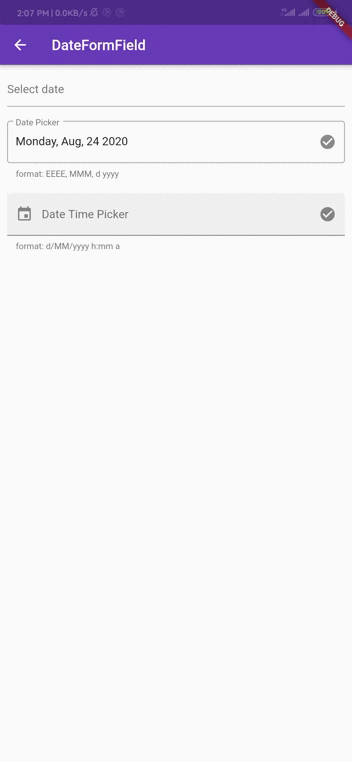
DateFormField wraps a TextField and integrates it with the enclosing Form. This provides additional functionality, such as validation and integration with other FormField widgets.
Getting Started #
Add this to your package's pubspec.yaml file:
dependencies:
date_form_field: ^0.0.2
Usage #
import package
import 'package:date_form_field/date_form_field.dart';
DateFormField(
showPicker: showPicker,
onDateChanged: (DateTime date) {
// your code
},
)
Future<DateTime> showPicker() async {
DateTime date = await showDatePicker(
context: context,
initialDate: firstDate,
firstDate: firstDate,
lastDate: lastDate,
);
return date;
}
More complete example:
DateFormField(
format: 'd/MM/yyyy h:mm a',
initialValue: new DateTime.now().toString(),
onSaved: (String dateStr) {
// your code
},
onChanged: (String dateStr) {
// your code
},
onDateChanged: (DateTime date) {
// your code
},
validator: (value) {
if (value.isEmpty) return 'Date can\'t be blank.';
return null;
},
decoration: InputDecoration(
labelText: 'Date',
suffixIcon: Icon(
Icons.event,
),
),
showPicker: () {
return showDateTimePicker(
context: context,
);
},
)
showDateTimePicker #
usage #
DateFormField(
showPicker: showPicker,
onDateChanged: (DateTime date) {
// your code
},
)
Future<DateTime> showPicker() async {
DateTime date = await showDateTimePicker(
context: context, // required
initialDate: firstDate, // optional
firstDate: firstDate, // optional
lastDate: lastDate, // optional
);
return date;
}
function #
Future<DateTime> showDateTimePicker({
@required BuildContext context,
DateTime initialDate,
DateTime firstDate,
DateTime lastDate,
DateTime currentDate,
DatePickerEntryMode initialDatePickerEntryMode: DatePickerEntryMode.calendar,
TimePickerEntryMode initialTimePickerEntryMode: TimePickerEntryMode.dial,
SelectableDayPredicate selectableDayPredicate,
String helpText,
String cancelText,
String confirmText,
Locale locale,
bool useRootNavigator: true,
RouteSettings routeSettings,
TextDirection textDirection,
TransitionBuilder builder,
DatePickerMode initialDatePickerMode: DatePickerMode.day,
String errorFormatText,
String errorInvalidText,
String fieldHintText,
String fieldLabelText,
})