cross_scroll 0.0.6
cross_scroll: ^0.0.6 copied to clipboard
A CrossScroll is a flutter Package that permits it’s child views to be scrolled vertically and Horizontally.
Package: Cross Scroll #
A CrossScroll is a flutter Package that permits it’s child views to be scrolled vertically and Horizontally. This is important because in many cases you need content to be scrolled on both side.
List of features #
- The vertical scrollbar wil never hides when the scrolling on horizontal axis and vice versa. The scrollBar never hides with scrolling on any directions.
- Support track onClick scrolls.
- Support thumb Drag scrolls.
- Support All feature
SingleChildScrollView
have.
Tested Platform #
WEB
- MicroSoft Edge
Windows
- Windows 10
Mobiles
- IOS 15.2
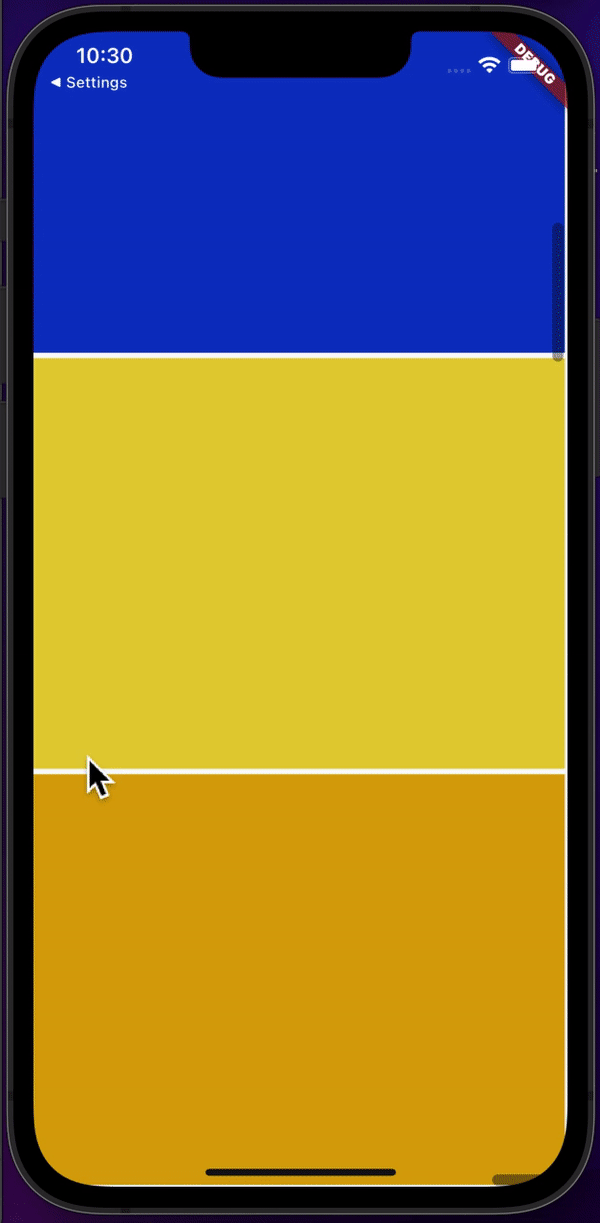
- Android 11
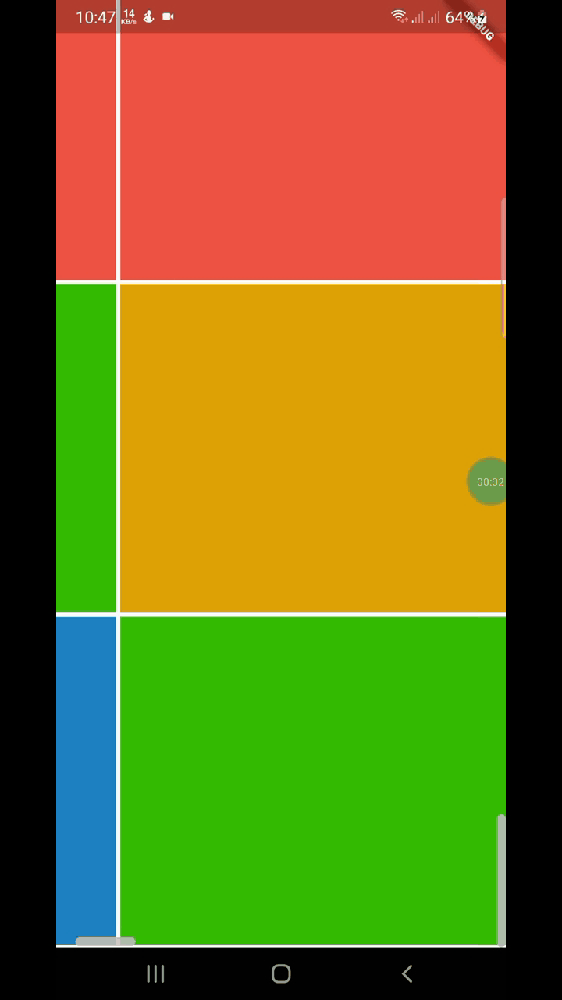
Getting started #
CrossScrollView #
CrossScroll(
child:///your child
)
Modify Thumb and Track
final CrossScrollBar _crossScrollBar= const CrossScrollBar(
isAlwaysShown:true,
hoverThickness: 8,
thickness :8,
radius : Radius.elliptical(8, 8),
showTrackOnHover: true,
trackVisibility: false,
);
Scrolling Behaviours
final CrossScrollStyle _crossScrollStyle = CrossScrollStyle(
physics: BouncingScrollPhysics(),
clipBehavior:Clip.hardEdge,
dragStartBehavior: DragStartBehavior.start,
keyboardDismissBehavior: ScrollViewKeyboardDismissBehavior.manual,
reverse: false,
padding:const EdgeInsets.symmetric(vertical: 2),
);
Full Example
import 'package:cross_scroll/cross_scroll.dart';
import 'package:flutter/material.dart';
import 'dart:math' as math;
class Example extends StatelessWidget {
Example({Key? key}) : super(key: key);
final random = math.Random();
final List<Color> colors = const [
Color(0xdfde2929),
Color(0xdfe0c919),
Color(0xfd3cd506),
Color(0xdfaf08ba),
Color(0xffdc0e79),
Color(0xf80bdbab),
Color(0xff0b32c2),
Color(0xfad7a306),
Color(0xdf0877b3),
Color(0xdf5d0ce7),
Color.fromARGB(249, 20, 141, 150),
Color.fromARGB(223, 18, 10, 32),
Color.fromARGB(223, 109, 170, 39),
Color.fromARGB(223, 131, 98, 8),
];
final CrossScrollBar _crossScrollBar = const CrossScrollBar(
isAlwaysShown: true,
hoverThickness: 8,
thickness: 8,
radius: Radius.elliptical(8, 8),
showTrackOnHover: true,
trackVisibility: false,
);
final CrossScrollStyle _crossScrollStyle = CrossScrollStyle(
physics: BouncingScrollPhysics(),
clipBehavior:Clip.hardEdge,
dragStartBehavior: DragStartBehavior.start,
keyboardDismissBehavior: ScrollViewKeyboardDismissBehavior.manual,
reverse: false,
padding:const EdgeInsets.symmetric(vertical: 2),
);
@override
Widget build(BuildContext context) {
return CrossScroll(
horizontalScroll: _crossScrollStyle,
verticalScroll: _crossScrollStyle,
verticalBar: _crossScrollBar,
horizontalBar: _crossScrollBar,
child: Column(
children: [
for (var i = 1; i < 11; i++)
Row(
children: [
for (var i = 1; i < 11; i++)
Container(
margin: const EdgeInsets.all(2),
width: MediaQuery.of(context).size.width / 2,
height: MediaQuery.of(context).size.height / 2,
color: colors[random.nextInt(13).round().toInt()],
),
],
)
],
),
);
}
}