clean_calendar 2.0.0
clean_calendar: ^2.0.0 copied to clipboard
A brand-new Flutter calendar package that enables you to make a simple, lovely, and customizable calendar.
Word from creator #
Helloπ, Clean Calendar is in the early stages of development and can have many breaking changes. If you have any questions or suggestions, contact me via GitHub issues, email, discord, or any of the ways listed on my GitHub profile.
Yes, giving the package a π(Pub) or β(Github) will encourage me to work on it more.
Package description #
Get ready to enhance your Flutter app with this fantastic calendar package! With its simple yet elegant design, you can create a fully customized calendar that suits your needs. It provides a customizable month view and the ability to highlight dates with streaks. It is just the beginning, and we will add more features.
Features #
- Display dates as streaks
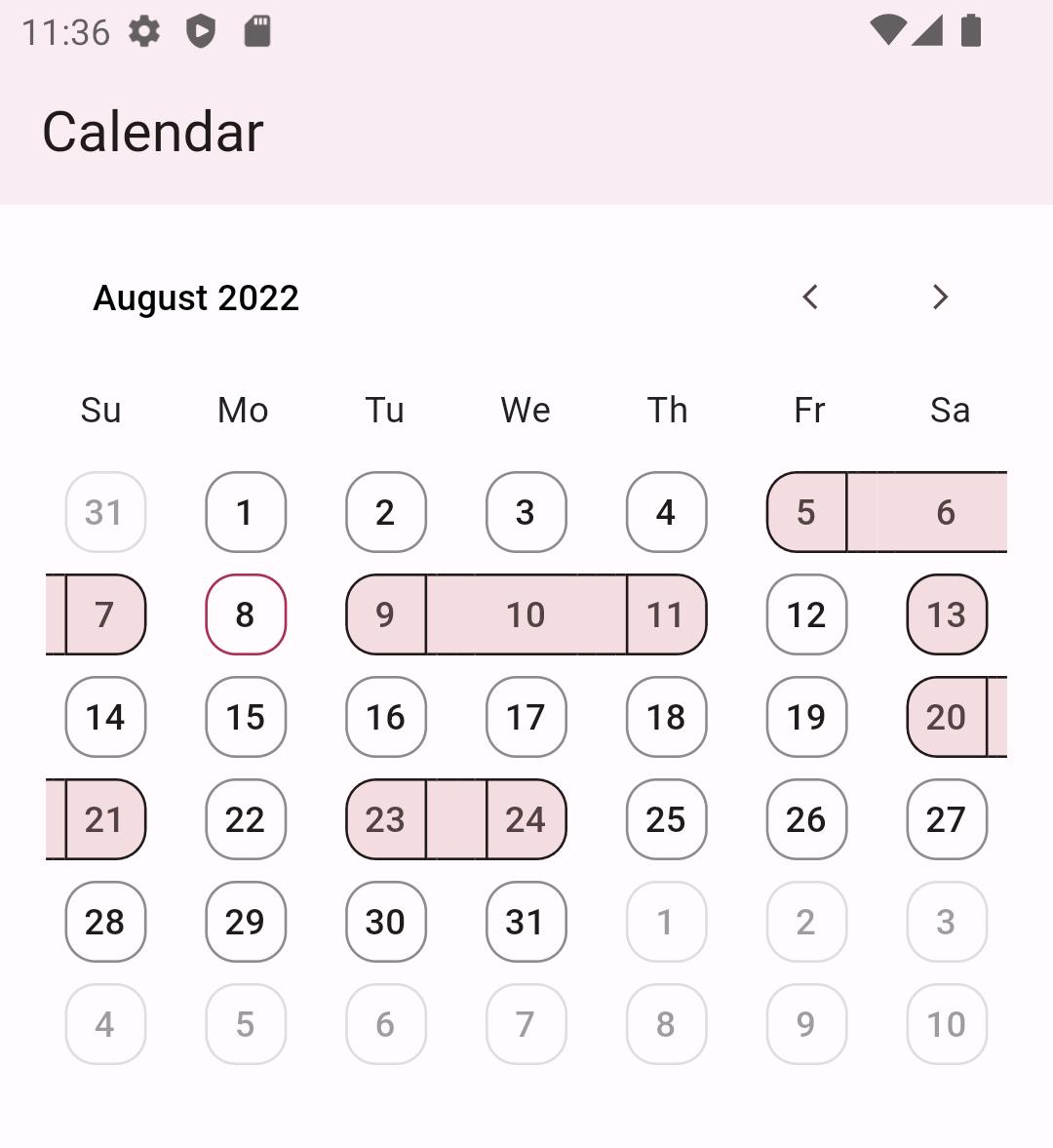
- Monthly calendar
Dark mode | Light mode |
---|---|
![]() |
![]() |
- Weekly calendar
Dark mode | Light mode |
---|---|
![]() |
![]() |
Getting started #
- In pubspec.yaml, add this dependency:
clean_calendar:
- Add this package to your project:
import 'package:clean_calendar/clean_calendar.dart';
Basic Usage #
class Home extends StatelessWidget {
const Home({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
elevation: 2,
title: const Text("Calendar"),
),
body: CleanCalendar(
datesForStreaks: [
DateTime(2022, 8, 5),
DateTime(2022, 8, 6),
DateTime(2022, 8, 7),
DateTime(2022, 8, 9),
DateTime(2022, 8, 10),
DateTime(2022, 8, 11),
DateTime(2022, 8, 13),
DateTime(2022, 8, 20),
DateTime(2022, 8, 21),
DateTime(2022, 8, 23),
DateTime(2022, 8, 24),
],
),
);
}
}
Customisations #
- Customise date boundary behaviour and tap behaviour
CleanCalendar( |
CleanCalendar( |
---|---|
![]() |
![]() |
Note: This dense mode could lead to overflow if the available width to calendar is smaller than 280px so it is advised to disable them in smaller space than 280px.
- Disable and hide different types of dates
CleanCalendar( |
CleanCalendar( |
---|---|
![]() |
![]() |
Also, Available for other types of dates : -
Selected Dates | Visible Month Dates | Current Date | Streak Dates |
---|---|---|---|
CleanCalendar( |
CleanCalendar( |
CleanCalendar( |
CleanCalendar( |
- Customise dates look by changing its properties
CleanCalendar( |
CleanCalendar( |
CleanCalendar( |
---|---|---|
![]() |
![]() |
![]() |
- Customise weekdays header by changing its properties
CleanCalendar( |
---|
![]() |
- Customise navigator header by changing its properties
CleanCalendar( |
---|
![]() |
Date Selection #
- Multiple date selection with customization
CleanCalendar( |
---|
![]() |
-
Single date selection
For single date selection modify the above example code
onSelectedDates
logic to store only single latest date as selected. -
Range, multiple ranges (coming soon)
Changing calendar view type #
Currently mothly and weekly views are added. You can change them as shown below:
CleanCalendar(
// Setting DatePickerCalendarView.weekView for weekly view
datePickerCalendarView: DatePickerCalendarView.weekView,
)
Note: datePickerCalendarView
defaults to DatePickerCalendarView.monthView.
Changing calendar start weekday #
You can change the claendar start weekday as shown below:
CleanCalendar(
// Setting start weekday
startWeekday: WeekDay.monday,
)
Note: startWeekday
defaults to WeekDay.sunday.
Changing weekday and month symbols #
You can change the claendar months and weekdays symbols as shown below:
CleanCalendar(
// Setting custom weekday symbols
weekdaysSymbol: const Weekdays(sunday: "s", monday: "m", tuesday: "t", wednesday: "w", thursday: "t", friday: "f", saturday: "s"),
// Setting custom month symbols
monthsSymbol: const Months(january: "Jan", february: "Feb", march: "Mar", april: "Apr", may: "May", june: "Jun", july: "Jul", august: "Aug", september: "Sep", october: "Oct", november: "Nov", december: "Dec"),
)
Note: weekdaysSymbol
defaults to Mo, Tu, We, Th, Fr, Sa, Su from monday to sunday and monthsSymbol
defaults to January, February, March, April, May, June, July, August, September, October, November, December.
Accessing selected dates #
To access selected dates use the onSelectedDates
callback but keep in mind that this callback always provides a list of dates as shown below:
CleanCalendar(
...
onSelectedDates: (List<DateTime> selectedDates) {
// Called every time dates are selected or deselected.
print(selectedDates);
},
)
Note: Even for single selection mode it provides a list with just one date and for multiple selection it provides a list with multiple dates.
Providing pre-selected dates #
You can provide pre-selected dates by providing a list of dates to selectedDates
as shown below:
CleanCalendar(
...
selectedDates: [
DateTime(2022, 8, 6),
DateTime(2022, 8, 9),
DateTime(2022, 8, 11),
],
)
Note: Even with selection mode set to single or disable it will still show the provided pre-selected dates as selected. And I think is the correct behavious as sometimes we might want to show selected dates while also preventing users from interacting with them.
Providing start and end dates #
You can provide start and end dates for calendar view as shown below:
CleanCalendar(
...
// Setting start date of calendar.
startDateOfCalendar: DateTime(2022,6,5),
// Setting end date of calendar.
endDateOfCalendar: DateTime(2022,10,15),
)
Note: startDateOfCalendar
defaults to DateTime.utc(0000, 1, 1) and endDateOfCalendar
defaults to DateTime.utc(9999, 1, 1).
Providing initial month view for calendar #
You can provide initial month view for calendar by providing date of that month as shown below:
CleanCalendar(
...
// Setting initial month view of calendar.
initialViewMonthDateTime: DateTime(2022,9,5),
)
Note: initialViewMonthDateTime
defaults to current date and if current is not between startDateOfCalendar and endDateOfCalendar then defaults to start date..
Providing current date for calendar #
You can provide current date for calendar as shown below:
CleanCalendar(
...
// Setting current date of calendar.
currentDateOfCalendar: DateTime(2022,9,5),
)
Note: currentDateOfCalendar
defaults to DateTime.now().
Listening for calendar view week or month change #
You can listen and get the current calendar view date on calendar view change. Useful in case you want to change your ui acording to the dates visible to user.
CleanCalendar(
...
onCalendarViewDate: (DateTime calendarViewDate) {
// Called every time view is changed like scrolled etc.
print(calendarViewDate);
},
)
Note: For now onCalendarViewDate
will only provide the view starting date. Later I may can add an option to get all dates of current view depending on users request. Also, onCalendarViewDate
is called only on change of view like scroll, etc and will not be called on the creation of calendar as at thst time we already know the date.
Additional information #
Support is surely planned for these:
-
Complete documentation.
-
Option to disable user interactions on whole view, past dates, and dates after-before end-start date. (π)
-
More customization and premade templates for calendar. (π’)
-
More calendar view types. (π’)
-
Date selection options such as range, and multiple range selections. (π’)
-
A calendar option for choosing a view by year, month, or day. (π’)
-
Support for dates to be displayed in events style. (π’)
-
Dry mode, which optimizes performance for low-end devices by removing or substituting heavy animations and widgets. (π’)
And, if I'm forgetting something crucial/important/necessary then please let me know.