approval_tests 0.1.0-dev
approval_tests: ^0.1.0-dev copied to clipboard
Approval Tests implementation in Dart. Inspired by ApprovalTests.
Approval Tests implementation in Dart 🚀
📖 About #
Unit testing asserts can be difficult to use. Approval tests
simplify this by taking a snapshot of the results, and confirming that they have not changed.
In normal unit testing, you say expect(person.getAge(), 5)
. Approvals allow you to do this when the thing that you want to assert is no longer a primitive but a complex object. For example, you can say, Approvals.verify(person)
.
I am writing an implementation of a great tool like Approval Tests in Dart. If anyone wants to help, please text me. 🙏
📦 Installation #
Add the following to your pubspec.yaml
file:
dependencies:
approval_tests: ^0.1.0-dev
📚 How to use #
Comparators #
You can use different comparators to compare files. The default is CommandLineComparator
which compares files in the console.
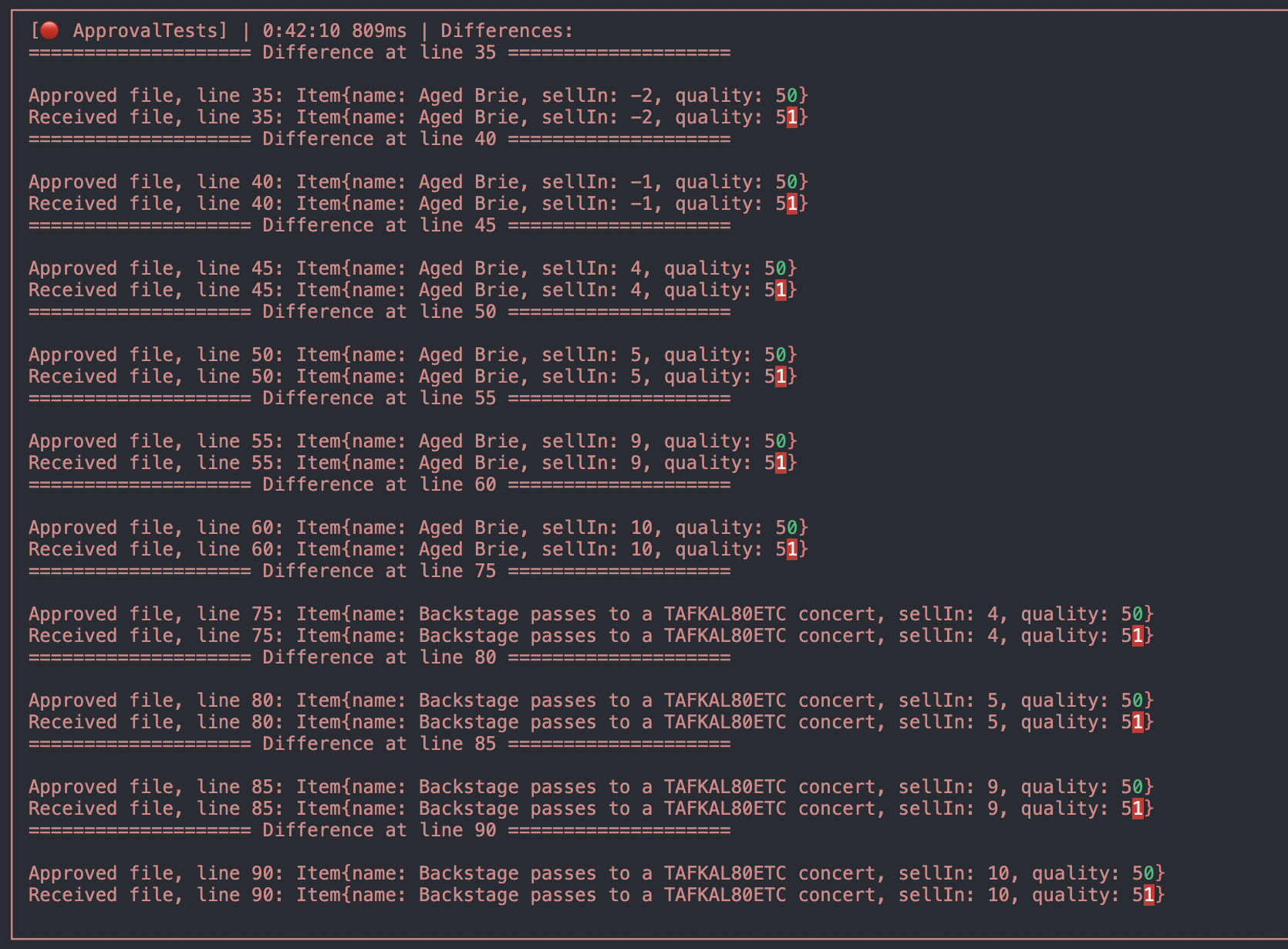
To use IDEComparator
you just need to add it to options
:
options: const Options(
comparator: IDEComparator(
ide: ComparatorIDE.visualStudioCode,
),
),
But before you add an IDEComparator
you need to do the initial customization:
-
Visual Studio Code
- For this method to work, you need to have Visual Studio Code installed on your machine.
- And you need to have the
code
command available in your terminal. - To enable the
code
command, pressCmd + Shift + P
and typeShell Command: Install 'code' command in PATH
.
-
IntelliJ IDEA
- For this method to work, you need to have IntelliJ IDEA installed on your machine.
- And you need to have the
idea
command available in your terminal. - To enable the
idea
command, you need to create the command-line launcher usingTools - Create Command-line Launcher
in IntelliJ IDEA.
-
Android Studio
- For this method to work, you need to have Android Studio installed on your machine.
- And you need to have the
studio
command available in your terminal. - To enable the
studio
command, you need to create the command-line launcher usingTools - Create Command-line Launcher
in Android Studio.
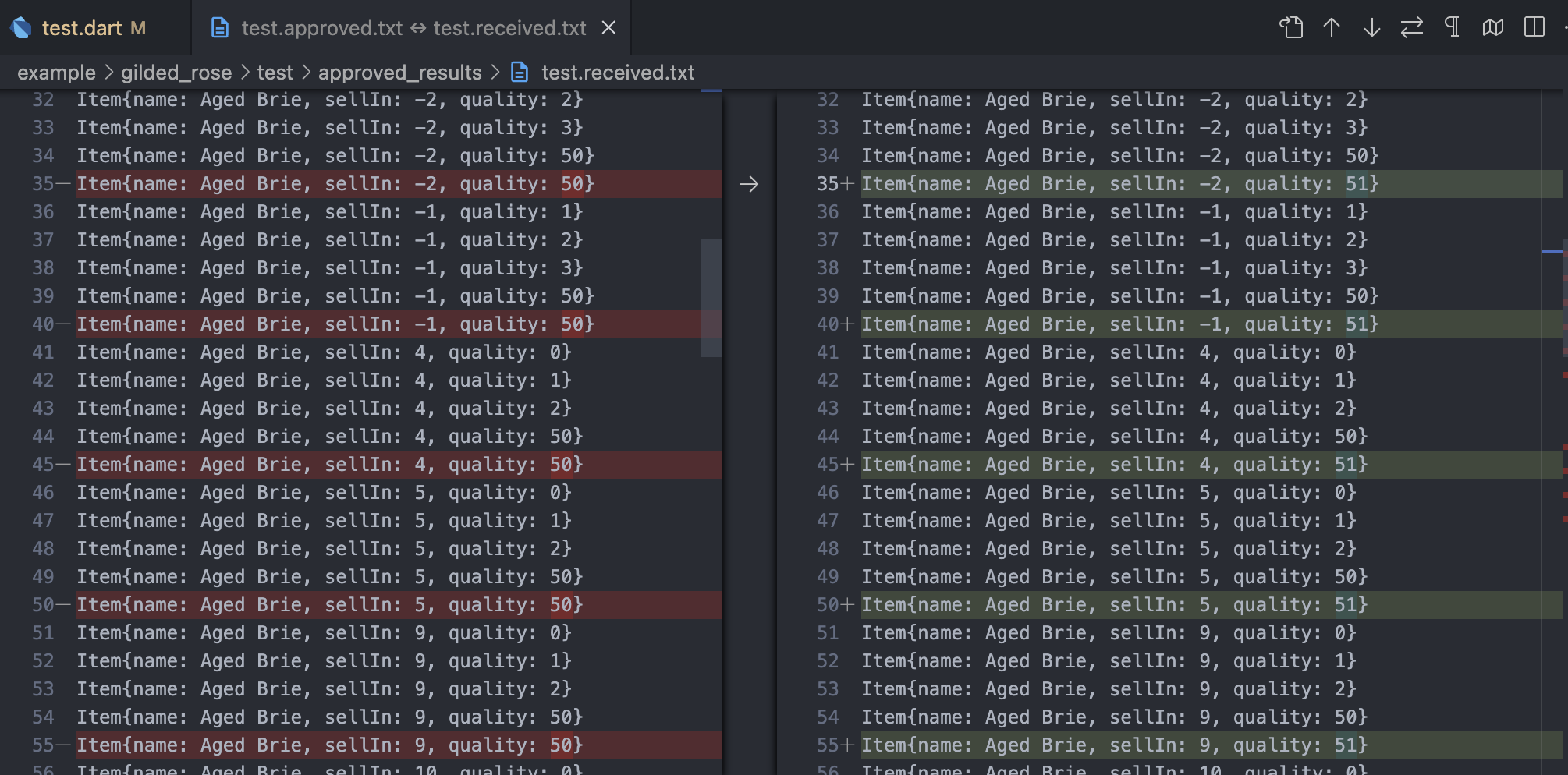
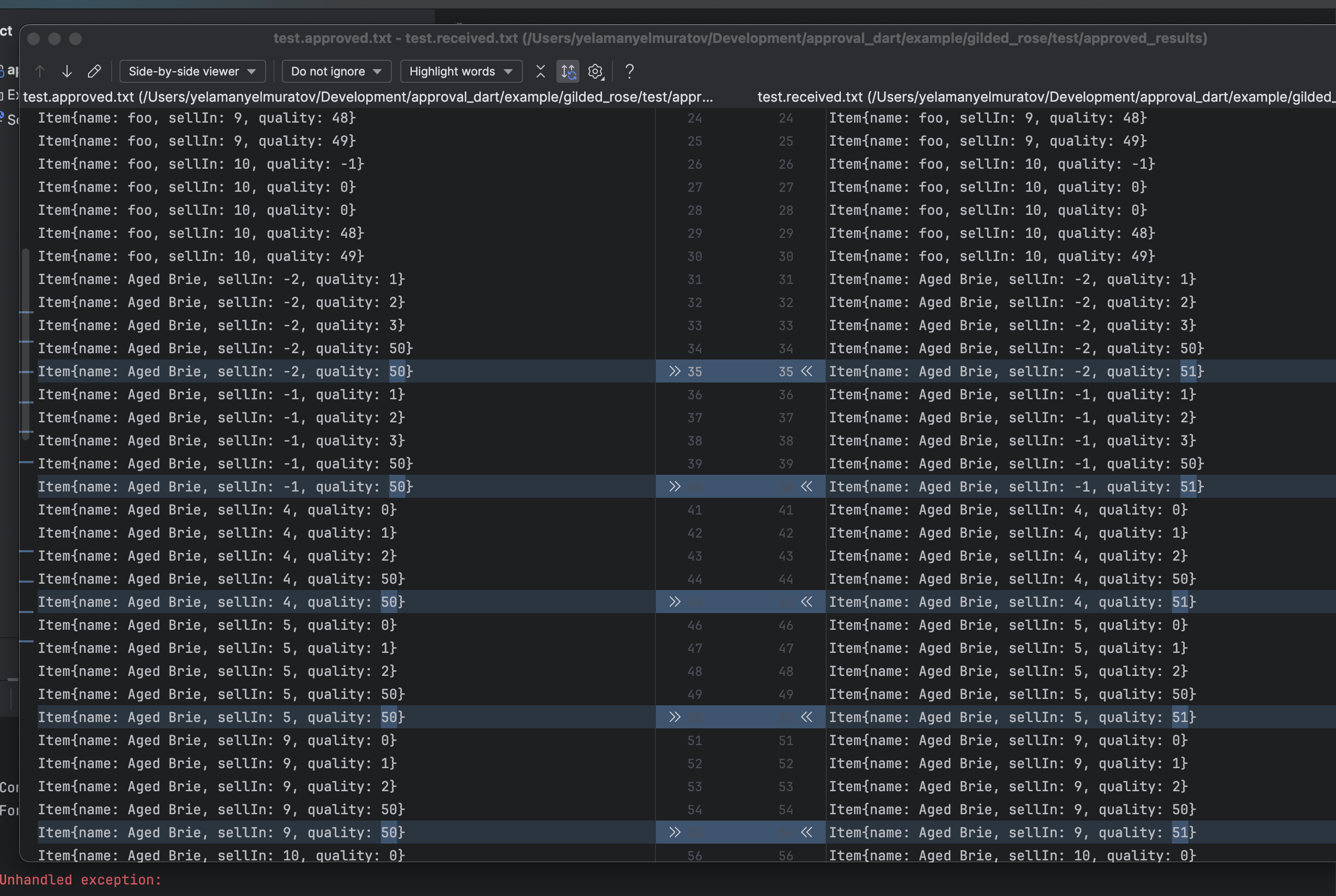
📝 Examples #
JSON example #
import 'package:approval_tests/approval_dart.dart';
import 'package:test/test.dart';
void main() {
group('Approval Tests for Complex Objects', () {
test('test complex JSON object', () {
var complexObject = {
'name': 'JsonTest',
'features': ['Testing', 'JSON'],
'version': 0.1,
};
ApprovalTests.verifyAsJson(complexObject);
});
});
}

Gilded Rose #
void main() {
// Define all test cases
const allTestCases = [
["foo", "Aged Brie", "Backstage passes to a TAFKAL80ETC concert", "Sulfuras, Hand of Ragnaros"],
[-1, 0, 5, 6, 10, 11],
[-1, 0, 1, 49, 50]
];
group('Approval Tests for Gilded Rose', () {
test('should verify all combinations of test cases', () {
// Perform the verification for all combinations
ApprovalTests.verifyAllCombinations(
inputs: allTestCases,
options: const Options(
comparator: IDEComparator(
ide: ComparatorIDE.visualStudioCode,
),
),
processor: processItemCombination,
file: "example/gilded_rose/test/approved_results/test",
);
});
});
}
// Function to process each combination and generate output for verification
String processItemCombination(Iterable<List<dynamic>> combinations) {
final receivedBuffer = StringBuffer();
for (var combination in combinations) {
// Extract data from the current combination
String itemName = combination[0];
int sellIn = combination[1];
int quality = combination[2];
// Create an Item object representing the current combination
Item testItem = Item(itemName, sellIn: sellIn, quality: quality);
// Passing testItem to the application
GildedRose app = GildedRose(items: [testItem]);
// Updating quality of testItem
app.updateQuality();
// Adding the updated item to expectedItems
receivedBuffer.writeln(testItem.toString());
}
// Return a string representation of the updated item
return receivedBuffer.toString();
}
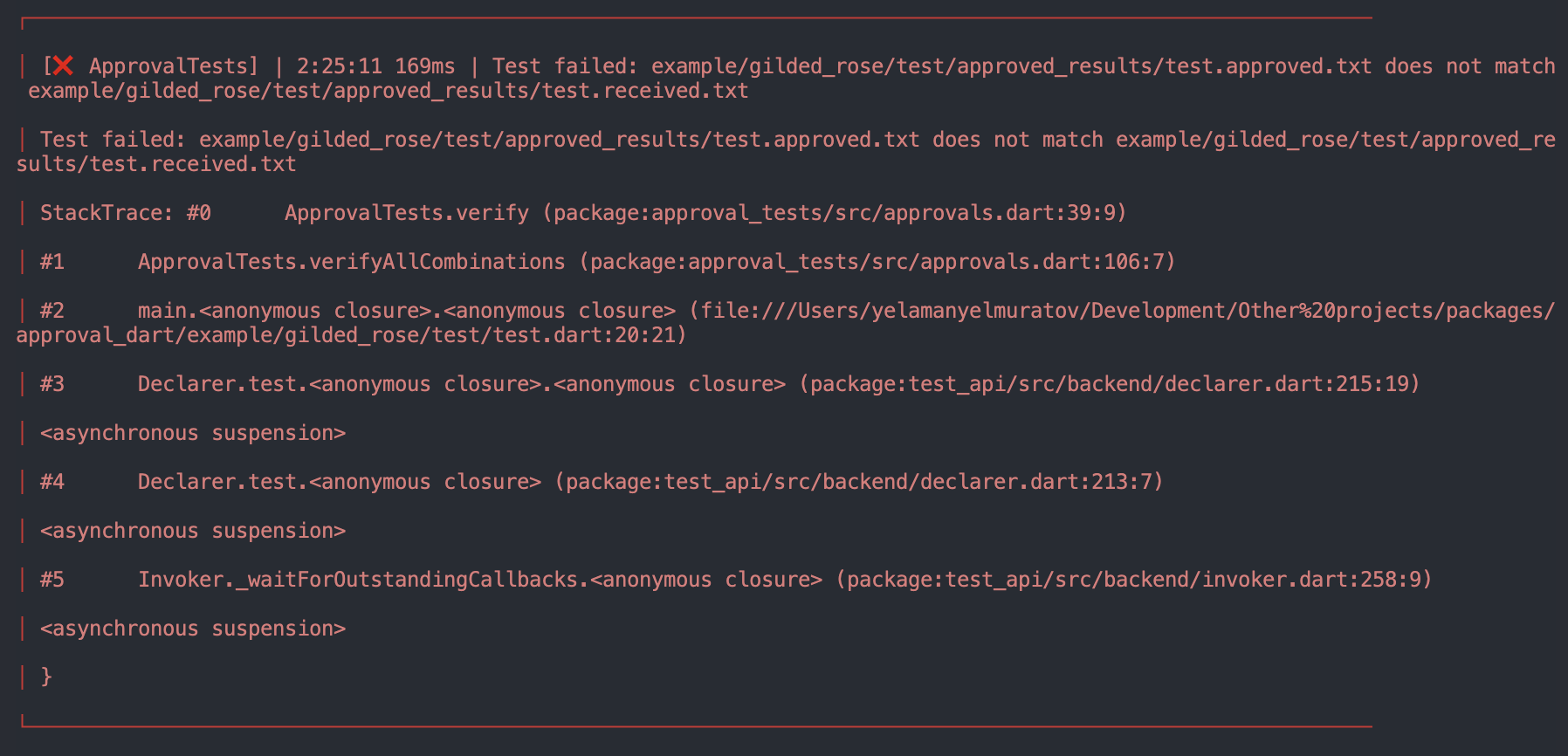
❓ Which File Artifacts to Exclude from Source Control #
You must add any approved
files to your source control system. But received
files can change with any run and should be ignored. For Git, add this to your .gitignore
:
*.received.*
✉️ For More Information #
Questions? #
Ask me on Telegram: @yelmuratoff
.
Email: yelamanyelmuratov@gmail.com
Video Tutorials #
- Getting Started with ApprovalTests.Swift
- How to Verify Objects (and Simplify TDD)
- Verify Arrays and See Simple, Clear Diffs
- Write Parameterized Tests by Transforming Sequences
- Wrangle Legacy Code with Combination Approvals
You can also watch a series of short videos about using ApprovalTests in .Net on YouTube.
Podcasts #
Prefer learning by listening? Then you might enjoy the following podcasts:
🤝 Contributing #
Show some 💙 and star the repo to support the project! 🙌
The project is in the process of development and we invite you to contribute through pull requests and issue submissions. 👍
We appreciate your support. 🫰