trackier_sdk_flutter 1.6.24
trackier_sdk_flutter: ^1.6.24 copied to clipboard
This is trackier flutter SDK
flutter sdk #
Table of Content #
Integration #
- Add Trackier SDK to your app
- Implement and initialize the SDK
- SDK Signing
- Track Events
- Track Uninstall for Android
- Proguard Settings
Add Trackier SDK to your app #
Add the SDK #
Run this command:
With Flutter:
$ flutter pub add trackier_sdk_flutter
This will add a line like this to your package's pubspec.yaml
dependencies:
trackier_sdk_flutter: ^1.6.24
Then navigate to your project in the terminal and run:
$ flutter packages get
Adding Android install referrer to your app #
Add the Android Install Referrer as a dependency. You can find the latest version here
dependencies {
// make sure to use the latest SDK version:
implementation 'com.android.installreferrer:installreferrer:2.2'
}
Add required permissions #
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
<!-- Optional : -->
<uses-permission android:name="android.permission.READ_PHONE_STATE" />
Getting Google Advertising ID #
- Add the google advertising id dependency in your android/app/build.gradle
dependencies {
// This can be added where the SDK dependency has been added
implementation 'com.google.android.gms:play-services-ads-identifier:18.0.1'
}
- Update your Android Manifest file located in android/app/src/main/AndroidManifest.xml. This is required if your app is targeting devices with android version 12+
<uses-permission android:name="com.google.android.gms.permission.AD_ID"/>
- Add meta data inside the application tag
<meta-data
android:name="com.google.android.gms.version"
android:value="@integer/google_play_services_version" />
Update Pod Dependencies #
For iOS app make sure to go to ios folder and install Cocoapods dependencies:
$ cd ios && pod install
Implement and initialize the SDK #
Retrieve your dev key #
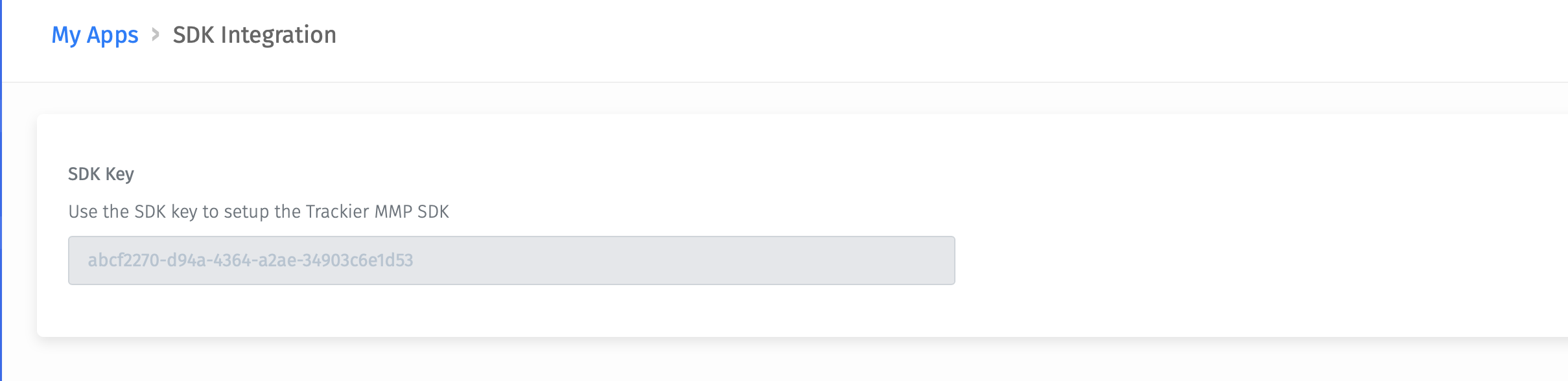
Initialize the SDK #
Import the following libraries
import 'package:trackier_sdk_flutter/trackierfluttersdk.dart';
import 'package:trackier_sdk_flutter/trackierconfig.dart';
import 'package:trackier_sdk_flutter/trackierevent.dart';
Important: it is crucial to use the correct dev key when initializing the SDK. Using the wrong dev key or an incorrect dev key impact all traffic sent from the SDK and cause attribution and reporting issues.
Inside the class, inside initPlatformState function , initialize SDK
TrackerSDKConfig trackerSDKConfig = new TrackerSDKConfig("xxxx-xx-4505-bc8b-xx", "production");
Trackierfluttersdk.initializeSDK(trackerSDKConfig);
- The first argument is your Trackier Sdk api key.
- The second argument is environment which can be either "development" or "production" or "testing"
- After that pass the sdkConfig reference to TrackierSDK.initialize method.
Assosiate User Info during initialization of sdk #
To assosiate Customer Id , Customer Email and Customer additional params during initializing sdk
TrackierSDK.setUserId("XXXXXXXX");
TrackierSDK.setUserEmail("abc@gmail.com");
TrackierSDK.initialize(sdkConfig);
Note #
For additional user details , make a map and pass it in setUserAdditionalDetails function.
var userAdditonalDetail = Map<String, Object>();
userAdditonalDetail["phoneNumber"] = 9876453210;
Trackierfluttersdk.setUserAdditonalDetail(userAdditonalDetail);
- The first method is to associate user Id in which
- The second method is to associate user email in which
SDK Signing #
TrackerSDKConfig trackerSDKConfig =
new TrackerSDKConfig("xx-5865-44fc-8fca-xx", "production");
trackerSDKConfig.setAppSecret("xxx", "xxx-xx");
Track Events #
Retrieve Event Id from dashboard
Track Simple Event #
TrackierEvent trackierEvent = new TrackierEvent("eventID");
trackierEvent.orderId = "orderID";
trackierEvent.param1 = "param1";
trackierEvent.param2 = "param2";
trackierEvent.setEventValue("ev1", "eventValue1");
trackierEvent.setEventValue("ev2", 1);
Trackierfluttersdk.trackerEvent(trackierEvent);
- Argument in Trackier event class is event Id which you want to call event for.
- You can associate inbuilt params with the event , in-built param list are below:- orderId, currency, currency, param1, param2, param3 ,param4, param5, param6, param7, param8, param9, param10
Track with Currency & Revenue Event #
TrackierEvent trackierEvent = new TrackierEvent("eventID");
trackierEvent.revenue = 10.0;
trackierEvent.currency = "INR";
trackierEvent.orderId = "orderID";
trackierEvent.param1 = "param1";
trackierEvent.param2 = "param2";
trackierEvent.setEventValue("ev1", "eventValue1");
trackierEvent.setEventValue("ev2", 1);
Trackierfluttersdk.trackerEvent(trackierEvent);
Add custom params with event #
var eventCustomParams = Map<String, Object>();
eventCustomParams.put("customParam1",XXXXX)
eventCustomParams.put("customParam2",XXXXX)
event.ev = eventCustomParams
TrackierSDK.trackEvent(event)
- First create a map
- Pass its reference to event.ev param of event
- Pass event reference to trackEvent method of TrackerSDK
Track Uninstall for Android #
Before you begin
- Install
firebase_core
and add the initialization code to your app if you haven't already. - Add your app to your Firebase project in the Firebase console.
Add the Analytics SDK to your app
-
From the root of your Flutter project, run the following command to install the plugin:
flutter pub add firebase_analytics
-
Once complete, rebuild your Flutter application:
flutter run
-
Once installed, you can access the
firebase_analytics
plugin by importing it in your Dart code:import 'package:firebase_analytics/firebase_analytics.dart';
-
Create a new Firebase Analytics instance by calling the
instance
getter onFirebaseAnalytics
:FirebaseAnalytics analytics = FirebaseAnalytics.instance;
-
Use the
analytics
instance obtained above to set the following user property:var trackierId = await Trackierfluttersdk.getTrackierId(); analytics.setUserProperty(name: "ct_objectId", value: trackierId);
-
Adding the above code to your app sets up a common identifier.
-
Set the
app_remove
event as a conversion event in Firebase. -
Use the Firebase cloud function to send uninstall information to Trackier MMP.
-
You can find the support article here.
Proguard Settings #
If your app is using proguard then add these lines to the proguard config file
-keep class com.trackier.sdk.** { *; }
-keep class com.google.android.gms.common.ConnectionResult {
int SUCCESS;
}
-keep class com.google.android.gms.ads.identifier.AdvertisingIdClient {
com.google.android.gms.ads.identifier.AdvertisingIdClient$Info getAdvertisingIdInfo(android.content.Context);
}
-keep class com.google.android.gms.ads.identifier.AdvertisingIdClient$Info {
java.lang.String getId();
boolean isLimitAdTrackingEnabled();
}
-keep public class com.android.installreferrer.** { *; }