
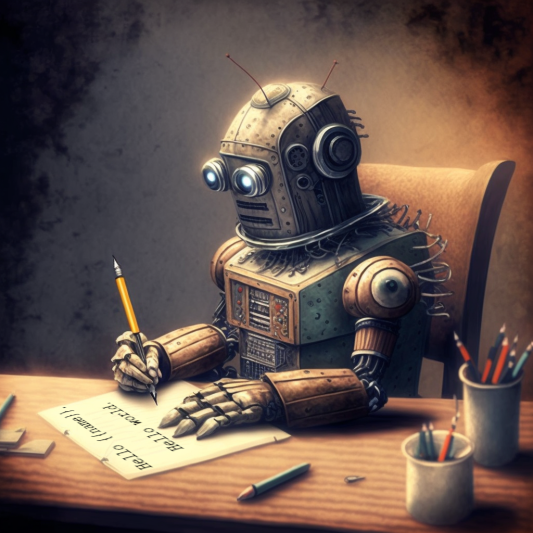
A flexible Dart library to parse templates and render output such as:
- Template expressions that can contain (combinations of):
- Data types
- Constants
- Variables
- Operators
- Functions
- (Template) files can be imported
- All of the above can be customized or you could add your own.
See: Installing
import 'package:shouldly/shouldly.dart';
import 'package:template_engine/template_engine.dart';
void main() {
var engine = TemplateEngine();
var parseResult = engine.parseText('Hello {{name}}.');
var renderResult = engine.render(parseResult, {'name': 'world'});
renderResult.text.should.be('Hello world.');
}
Note the documentation of the template_engine package was generated by itself.
See: tool/generate_documentation.dart
For more see: Examples
Tags are specific texts in templates that are replaced by the
TemplateEngine with other information.
A tag:
- Starts with some bracket and/or character combination, e.g.: {{
- Followed by some contents
- Ends with some closing bracket and/or character combination, e.g.: }}
A tag example: {{customer.name}}
By default the TemplateEngine tags start with {{ and end with }} brackets,
just like the popular template engines Mustache
and Handlebars.
You can also define alternative tag brackets in the TemplateEngine constructor
parameters. See TemplateEngine.tagStart and TemplateEngine.tagEnd.
It is recommended to use a start and end combination that is not used
elsewhere in your templates, e.g.: Do not use < > as tag start and end
if your template contains HTML or XML.
The TemplateEngine comes with default tags. You can replace or add your
own tags by manipulating the the TemplateEngine.tags field.
ExpressionTag |
description: | Evaluates an expression that can contain: * Data Types (e.g. boolean, number or String) * Constants (e.g. pi) * Variables (e.g. person.name ) * Operators (e.g. + - * /) * Functions (e.g. cos(7) ) * or any combination of the above |
expression example: | The volume of a sphere = {{ round( (3/4) * pi * (radius ^ 3) )}}. |
code example: | tag_expression_parser_test.dart |
A data type defines what the
possible values an expression, such as a variable, operator
or a function call, might take.
The TemplateEngine supports several default DataTypes.
You can adopt existing DataTypes or add your own custom DataTypes by
manipulating the TemplateEngine.dataTypes field.
See Example.
String |
description: | A form of data containing a sequence of characters |
syntax: | A string is declared with a chain of characters, surrounded by two single (') or double (") quotes to indicate the start and end of a string. In example: 'Hello' or "Hello" |
example: | string_test.dart |
Number |
description: | A form of data to express the size of something. |
syntax: | A number is declared with: * optional: positive (e.g. +12) or negative (e.g. -12) prefix or no prefix (12=positive) * one or more digits (e.g. 12) * optional fragments (e.g. 0.12) * optional: scientific notation: the letter E is used to mean"10 to the power of." (e.g. 1.314E+1 means 1.314 * 10^1which is 13.14).
|
example: | num_test.dart |
Boolean |
description: | A form of data with only two possible values: true or false |
syntax: | A boolean is declared with the word true or false. The letters are case insensitive. |
example: | bool_test.dart |
A Constant is a value that does not change value over time.
The TemplateEngine comes with several mathematical constants.
You can create and add your own Constants by
manipulating the TemplateEngine.constants field.
See Example.
e |
description: | Base of the natural logarithms. |
return type: | double |
code example: | e_test.dart |
ln10 |
description: | Natural logarithm of 10. |
return type: | double |
code example: | ln10_test.dart |
ln2 |
description: | Natural logarithm of 2. |
return type: | double |
code example: | ln2_test.dart |
log10e |
description: | Base-10 logarithm of e. |
return type: | double |
code example: | log10e_test.dart |
log2e |
description: | Base-2 logarithm of e. |
return type: | double |
code example: | log2e_test.dart |
pi |
description: | The ratio of a circle's circumference to its diameter |
return type: | double |
code example: | pi_test.dart |
A Variable is
a named container for some type of information
(like number, boolean, String etc...)
- Variables are stored as key, value pairs in a dart Map<String, Object> where:
- String=Variable name
- Object=Variable value
- Variables can be used in a tag expression
- Initial variable values are passed to the TemplateEngine.render method
- Variables can be modified during rendering
The variable name:
- must be unique and does not match a other [Tag] syntax
- must start with a letter, optionally followed by letters and or digits
- is case sensitive (convention: use camelCase)
Variables can be nested. Concatenate variable names separated with dot's
to get the variable value of a nested variable.
E.g.:
Variable map: {'person': {'name': 'John Doe', 'age',30}}
Variable Name person.name: refers to the variable value of 'John Doe'
Examples:
A function is a piece of dart code that performs a specific task.
So a function can basically do anything that dart code can do.
A function can be used anywhere in an tag expression
wherever that particular task should be performed.
The TemplateEngine supports several default functions.
You can adopt existing functions or add your own custom functions by
manipulating the TemplateEngine.functionGroups field.
See Example.
exp |
description: | Returns the natural exponent e, to the power of the value |
return type: | number |
expression example: | {{exp(7)}} should render: 1096.6331584284585 |
code example: | exp_test.dart |
parameter: | value | number | mandatory |
log |
description: | Returns the natural logarithm of the value |
return type: | number |
expression example: | {{log(7)}} should render: 1.9459101490553132 |
code example: | log_test.dart |
parameter: | value | number | mandatory |
sin |
description: | Returns the sine of the radians |
return type: | number |
expression example: | {{sin(7)}} should render: 0.6569865987187891 |
code example: | sin_test.dart |
parameter: | radians | number | mandatory |
asin |
description: | Returns the values arc sine in radians |
return type: | number |
expression example: | {{asin(0.5)}} should render: 0.5235987755982989 |
code example: | asin_test.dart |
parameter: | value | number | mandatory |
cos |
description: | Returns the cosine of the radians |
return type: | number |
expression example: | {{cos(7)}} should render: 0.7539022543433046 |
code example: | cos_test.dart |
parameter: | radians | number | mandatory |
acos |
description: | Returns the values arc cosine in radians |
return type: | number |
expression example: | {{acos(0.5)}} should render: 1.0471975511965979 |
code example: | acos_test.dart |
parameter: | value | number | mandatory |
tan |
description: | Returns the the tangent of the radians |
return type: | number |
expression example: | {{tan(7)}} should render: 0.8714479827243188 |
code example: | tan_test.dart |
parameter: | radians | number | mandatory |
atan |
description: | Returns the values arc tangent in radians |
return type: | number |
expression example: | {{atan(0.5)}} should render: 0.4636476090008061 |
code example: | atan_test.dart |
parameter: | value | number | mandatory |
sqrt |
description: | Returns the positive square root of the value. |
return type: | number |
expression example: | {{sqrt(9)}} should render: 3.0 |
code example: | sqrt_test.dart |
parameter: | value | number | mandatory |
round |
description: | Returns the a rounded number. |
return type: | number |
expression example: | {{round(4.445)}} should render: 4 |
code example: | round_test.dart |
parameter: | value | number | mandatory |
length |
description: | Returns the length of a string |
return type: | number |
expression example: | {{length("Hello")}} should render: 5 |
code example: | length_test.dart |
parameter: | string | String | mandatory |
engine.tag.documentation |
description: | Generates markdown documentation of all the tags within a TemplateEngine |
return type: | String |
expression example: | {{ engine.tag.documentation() }} |
parameter: | titleLevel | number | optional (default=1) | The level of the tag title |
engine.dataType.documentation |
description: | Generates markdown documentation of all the data types that can be used within a ExpressionTag of a TemplateEngine |
return type: | String |
expression example: | {{ engine.dataType.documentation() }} |
parameter: | titleLevel | number | optional (default=1) | The level of the tag title |
engine.constant.documentation |
description: | Generates markdown documentation of all the constants that can be used within a ExpressionTag of a TemplateEngine |
return type: | String |
expression example: | {{ engine.constant.documentation() }} |
parameter: | titleLevel | number | optional (default=1) | The level of the tag title |
engine.function.documentation |
description: | Generates markdown documentation of all the functions that can be used within a ExpressionTag of a TemplateEngine |
return type: | String |
expression example: | {{ engine.function.documentation() }} |
parameter: | titleLevel | number | optional (default=1) | The level of the tag title |
engine.operator.documentation |
description: | Generates markdown documentation of all the operators that can be used within a ExpressionTag of a TemplateEngine |
return type: | String |
expression example: | {{ engine.operator.documentation() }} |
parameter: | titleLevel | number | optional (default=1) | The level of the tag title |
engine.example.documentation |
description: | Generates markdown documentation of all the examples. This could be used to generate example.md file. |
return type: | String |
expression example: | {{ engine.example.documentation() }} |
parameter: | titleLevel | number | optional (default=1) | The level of the tag title |
template.source |
description: | Gives the relative path of the current template |
return type: | String |
expression example: | {{template.source()}} |
code example: | template_test.dart |
import |
description: | Imports, parses and renders a template file |
return type: | String |
expression example: | {{import('doc/template/common/generated_comment.template')}} |
code example: | import_test.dart |
parameter: | source | String | mandatory | The project path of the template file |
import.pure |
description: | Imports a file as is (without parsing and rendering) |
return type: | String |
expression example: | {{import.pure('test/src/template_engine_template_example_test.dart')}} |
code example: | import_pure_test.dart |
parameter: | source | String | mandatory | The project path of the file |
An operator behaves generally like functions,
but differs syntactically or semantically.
Common simple examples include arithmetic (e.g. addition with +) and
logical operations (e.g. &).
An operator can be used anywhere in an tag expression
wherever that particular Operator should be performed.
The TemplateEngine supports several standard operators.
You can adopt existing operators or add your own custom operators by
manipulating the TemplateEngine.operatorGroups field.
See custom_operator_test.dart.
operator: ( ... ) |
description: | Groups expressions together so that the are calculated first |
expression example: | {{(2+1)*3}} should render: 9 |
code example: | parentheses_test.dart |
operator: + |
parameter type: number |
description: | Optional prefix for positive numbers |
expression example: | {{+3}} should render: 3 |
code example: | positive_test.dart |
operator: - |
parameter type: number |
description: | Prefix for a negative number |
expression example: | {{-3}} should render: -3 |
code example: | negative_test.dart |
operator: ! |
parameter type: boolean |
description: | Prefix to invert a boolean, e.g.: !true =false |
expression example: | {{!true}} should render: false |
code example: | not_test.dart |
operator: ^ |
parameter type: number |
description: | Calculates a number to the power of the exponent number |
expression example: | {{2^3}} should render: 8 |
code example: | num_power_test.dart |
parameter type: boolean |
description: | Logical XOR with two booleans |
expression example: | {{true^false}} should render: true |
code example: | bool_xor_test.dart |
operator: * |
parameter type: number |
description: | Multiplies 2 numbers |
expression example: | {{2*3}} should render: 6 |
code example: | num_multiply_test.dart |
operator: / |
parameter type: number |
description: | Divides 2 numbers |
expression example: | {{6/4}} should render: 1.5 |
code example: | num_divide_test.dart |
operator: % |
parameter type: number |
description: | Calculates the modulo (rest value of a division) |
expression example: | {{8%3}} should render: 2 |
code example: | num_modulo_test.dart |
operator: & |
parameter type: boolean |
description: | Logical AND operation on two booleans |
expression example: | {{true&true}} should render: true |
code example: | bool_and_test.dart |
parameter type: String |
description: | Concatenates two strings |
expression example: | {{"Hel"&"lo"}} should render: Hello |
code example: | string_concatenate_test.dart |
operator: + |
parameter type: number |
description: | Adds two numbers |
expression example: | {{2+3}} should render: 5 |
code example: | num_addition_test.dart |
parameter type: String |
description: | Concatenates two strings |
expression example: | {{"Hel"+"lo"}} should render: Hello |
code example: | string_concatenate_test.dart |
operator: - |
parameter type: number |
description: | Subtracts two numbers |
expression example: | {{5-3}} should render: 2 |
code example: | num_subtract_test.dart |
operator: | |
parameter type: boolean |
description: | Logical OR operation on two booleans |
expression example: | {{false|true}} should render: true |
code example: | bool_or_test.dart |
operator: = |
description: | Assigns a value to a variable. A new variable will be created when it did not exist before, otherwise it will be overridden with a new value. |
expression example: | {{x=2}}{{x=x+3}}{{x}} should render: 5 |
code example: | assignment_test.dart |