telpo_flutter_sdk 0.1.1
telpo_flutter_sdk: ^0.1.1 copied to clipboard
A Flutter plugin for Telpo devices for handling connectivity and communication with the thermal printer.
telpo_flutter_sdk #
A Flutter plugin for handling connection and communication with Telpo thermal printer devices.
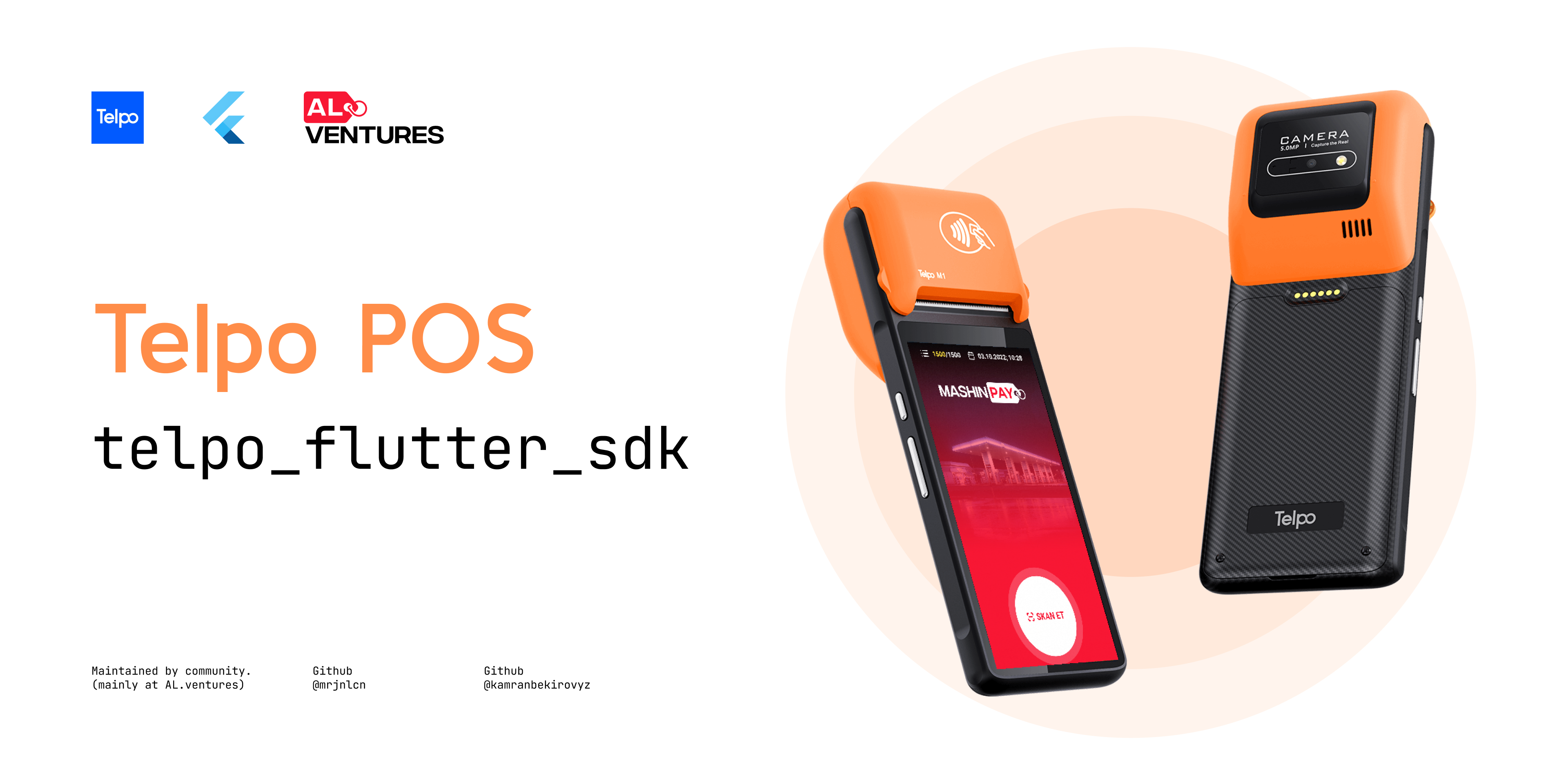
đĄ Motivation #
While developing MASHINPAY payment solution we've purchased Telpo thermal printers ("M1"s specifically) for printing invoice files after users' successful transaction. The Telpo devices come with a native SDK and documentation for it. Since there wasn't an official plugin for Flutter, we had to customize the plugin by ourselves. We've added so many useful methods for better handling exceptions, customizable print layout and etc.
âī¸ Android setup #
- Add
url "https://jitpack.io"
formaven
to project levelbuild.gradle
file (android/build.gradle
).
allprojects {
repositories {
...
maven { url "https://jitpack.io" }
...
}
}
- Set the
minSdkVersion
as 19 in application levelbuild.gradle
file (android/app/build.gradle
).
android {
defaultConfig {
...
minSdkVersion 19
...
}
}
đšī¸ Usage #
Initialization #
To get started, create an instance of TelpoFlutterChannel
:
final _telpoFlutterChannel = TelpoFlutterChannel();
Connecting with Telpo:
final bool connected = await _telpoFlutterChannel.connect();
// Result: true, false.
Checking Telpo's status:
final TelpoStatus status = await _telpoFlutterChannel.checkStatus();
// Result: ok, noPaper, overHeat, cacheIsFull, unknown.
Checking connection status with Telpo:
final bool status = await _telpoFlutterChannel.isConnected();
// Result: true, false.
Printing a sheet:
// Creating an empty sheet
final sheet = TelpoPrintSheet();
// Creating a text element
const textData = PrintData.text(
'TelpoFlutterSdk',
alignment: PrintAlignment.center,
fontSize: PrintedFontSize.size34,
);
// Creating 8-line empty space
const spacing = PrintData.space(step: 8);
// Inserting previously created text element to the sheet.
sheet.addElement(textData);
// Inserting previously created spacing element to the sheet.
sheet.addElement(spacing);
final PrintResult result = await _telpoFlutterChannel.print(sheet);
// Result: success, noPaper, lowBattery, overHeat, dataCanNotBeTransmitted, other.
đ Roadmap #
â
Well-written documentation đ¤
â
Document the platform-specific configurations.
â
Add explanations for Enum
values of PrintResult
and TelpoStatus
.
âŗ Print image file.
âŗ Toggle printing event via NFC. đ¤Š
âŗ Toggle printing event via BlueTooth, may be?
âŗ Checking if Telpo is available on the device?
đ¤ Contributors #
đ Credits #
While we were trying to understand and port the Telpo device to the Flutter framework we got very inspiring code samples and examples from Efikas's plugin (flutter_telpo) for Flutter which can be considered as a basic version of our plugin and our plugin can be considered as more customized of his since both of us used the same native implementation of the Telpo's Android SDK which comes together with the Telpo device.
đ Bugs/Requests #
If you encounter any problems please open an issue. If you feel the library is missing a feature, please raise a ticket on GitHub and we'll look into it. Pull requests are welcome.