system_tray 0.0.9
system_tray: ^0.0.9 copied to clipboard
system_tray that makes it easy to customize tray and work with your Flutter desktop app.
system_tray #
A Flutter package that that enables support for system tray menu for desktop flutter apps. on Windows, macOS and Linux.
Install #
In the pubspec.yaml of your flutter project, add the following dependency:
dependencies:
...
system_tray: ^0.0.9
In your library add the following import:
import 'package:system_tray/system_tray.dart';
Prerequisite #
Linux #
sudo apt-get install appindicator3-0.1 libappindicator3-dev
Example App #
Windows #
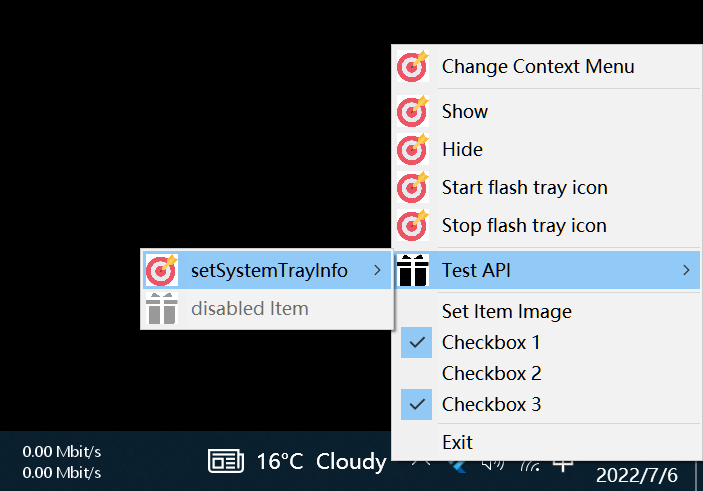
macOS #
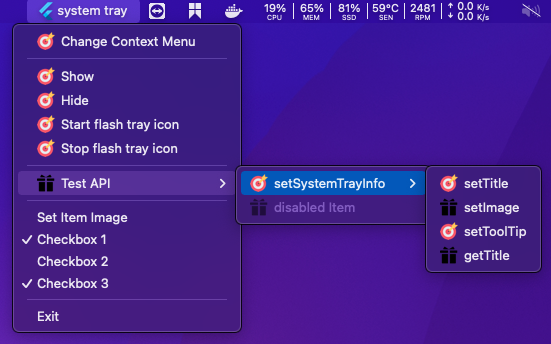
Linux #
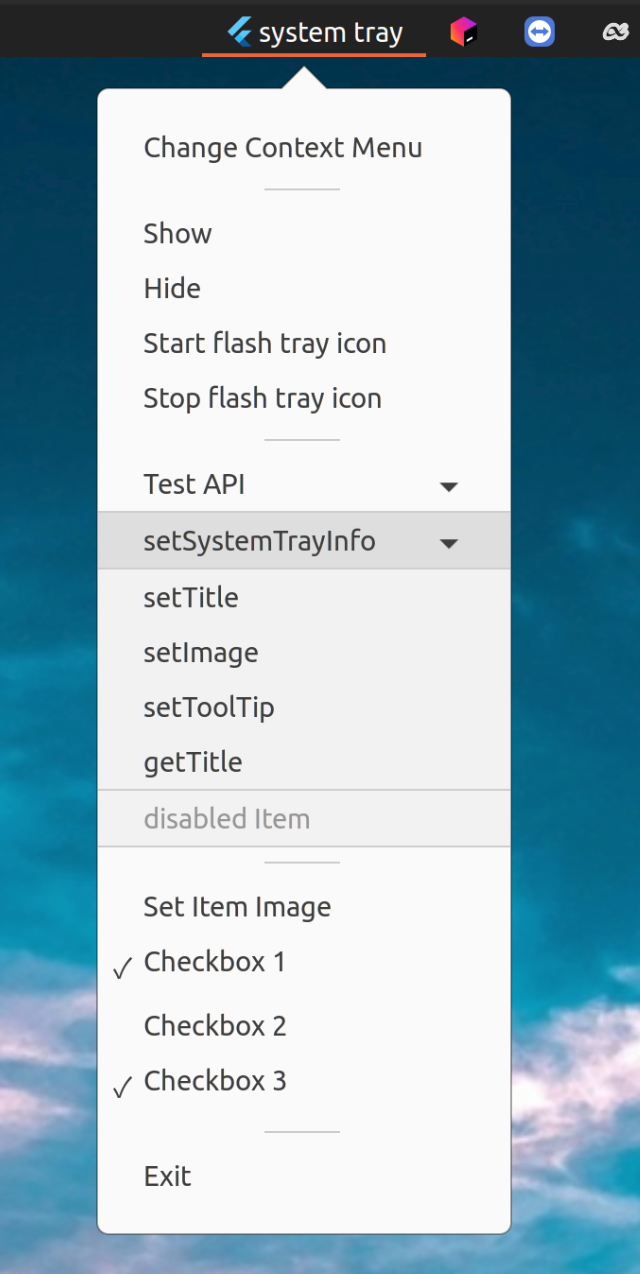
API #
Method | Description | Windows | macOS | Linux |
---|---|---|---|---|
initSystemTray | Initialize system tray | ✔️ | ✔️ | ✔️ |
setSystemTrayInfo | Modify the tray info |
|
|
|
setContextMenu | Set the tray context menu | ✔️ | ✔️ | ✔️ |
popUpContextMenu | Popup the tray context menu | ✔️ | ✔️ | ➖ |
registerSystemTrayEventHandler | Register system tray event |
|
|
➖ |
Usage #
Smallest example:
Future<void> initSystemTray() async {
String path =
Platform.isWindows ? 'assets/app_icon.ico' : 'assets/app_icon.png';
if (Platform.isMacOS) {
path = 'AppIcon';
}
final menu = [
MenuItem(label: 'Show', onClicked: _appWindow.show),
MenuItem(label: 'Hide', onClicked: _appWindow.hide),
MenuItem(label: 'Exit', onClicked: _appWindow.close),
];
// We first init the systray menu and then add the menu entries
await _systemTray.initSystemTray(
title: "system tray",
iconPath: path,
);
await _systemTray.setContextMenu(menu);
// handle system tray event
_systemTray.registerSystemTrayEventHandler((eventName) {
debugPrint("eventName: $eventName");
if (eventName == "leftMouseDown") {
} else if (eventName == "leftMouseUp") {
_systemTray.popUpContextMenu();
} else if (eventName == "rightMouseDown") {
} else if (eventName == "rightMouseUp") {
_appWindow.show();
}
});
}
Flashing icon example:
Future<void> initSystemTray() async {
String path =
Platform.isWindows ? 'assets/app_icon.ico' : 'assets/app_icon.png';
if (Platform.isMacOS) {
path = 'AppIcon';
}
final menu = [
MenuItem(label: 'Show', onClicked: _appWindow.show),
MenuItem(label: 'Hide', onClicked: _appWindow.hide),
MenuItem(
label: 'Start flash tray icon',
onClicked: () {
debugPrint("Start flash tray icon");
_timer ??= Timer.periodic(
const Duration(milliseconds: 500),
(timer) {
_toogleTrayIcon = !_toogleTrayIcon;
_systemTray.setSystemTrayInfo(
iconPath: _toogleTrayIcon ? "" : path,
);
},
);
},
),
MenuItem(
label: 'Stop flash tray icon',
onClicked: () {
debugPrint("Stop flash tray icon");
_timer?.cancel();
_timer = null;
_systemTray.setSystemTrayInfo(
iconPath: path,
);
},
),
MenuSeparator(),
SubMenu(
label: "Test API",
children: [
SubMenu(
label: "setSystemTrayInfo",
children: [
MenuItem(
label: 'set title',
onClicked: () {
final String text = WordPair.random().asPascalCase;
debugPrint("click 'set title' : $text");
_systemTray.setSystemTrayInfo(
title: text,
);
},
),
MenuItem(
label: 'set icon path',
onClicked: () {
debugPrint("click 'set icon path' : $path");
_systemTray.setSystemTrayInfo(
iconPath: path,
);
},
),
MenuItem(
label: 'set tooltip',
onClicked: () {
final String text = WordPair.random().asPascalCase;
debugPrint("click 'set tooltip' : $text");
_systemTray.setSystemTrayInfo(
toolTip: text,
);
},
),
],
),
MenuItem(label: 'disabled Item', enabled: false),
],
),
MenuSeparator(),
MenuItem(
label: 'Exit',
onClicked: _appWindow.close,
),
];
// We first init the systray menu and then add the menu entries
await _systemTray.initSystemTray(
title: "system tray",
iconPath: path,
toolTip: "How to use system tray with Flutter",
);
await _systemTray.setContextMenu(menu);
// handle system tray event
_systemTray.registerSystemTrayEventHandler((eventName) {
debugPrint("eventName: $eventName");
if (eventName == "leftMouseDown") {
} else if (eventName == "leftMouseUp") {
_systemTray.popUpContextMenu();
} else if (eventName == "rightMouseDown") {
} else if (eventName == "rightMouseUp") {
_appWindow.show();
}
});
}
Addition #
Recommended library that supports window control:
Q&A #
- Q: If you encounter the following compilation error
A: add libc++.tbdUndefined symbols for architecture x86_64: "___gxx_personality_v0", referenced from: ...
1. open example/macos/Runner.xcodeproj 2. add 'libc++.tbd' to TARGET runner 'Link Binary With Libraries'