smart_text_widget 0.0.3
smart_text_widget: ^0.0.3 copied to clipboard
A custom Flutter text widget that handles null values, empty strings, lists, maps, and numeric values. Supports truncation, scrolling, and error handling.
Smart Text Widget #
smart_text_widget
is a Flutter package that provides a highly customizable RTText
widget. It is designed to handle various scenarios such as null values, empty strings, numbers, lists, maps, and more. The widget also includes features like scrolling, truncation, and dynamic text display.
Features #
- Handles Various Data Types: Supports null, empty strings, numbers, lists, and maps.
- Dynamic Truncation: Set maximum width and truncate text dynamically.
- Scroll Support: Automatically scrolls for long strings or lists.
- Custom Separators for Lists: Choose how to separate list items (
,
or|
). - Error Handling: Displays default or custom error text in case of issues.
Screenshot #
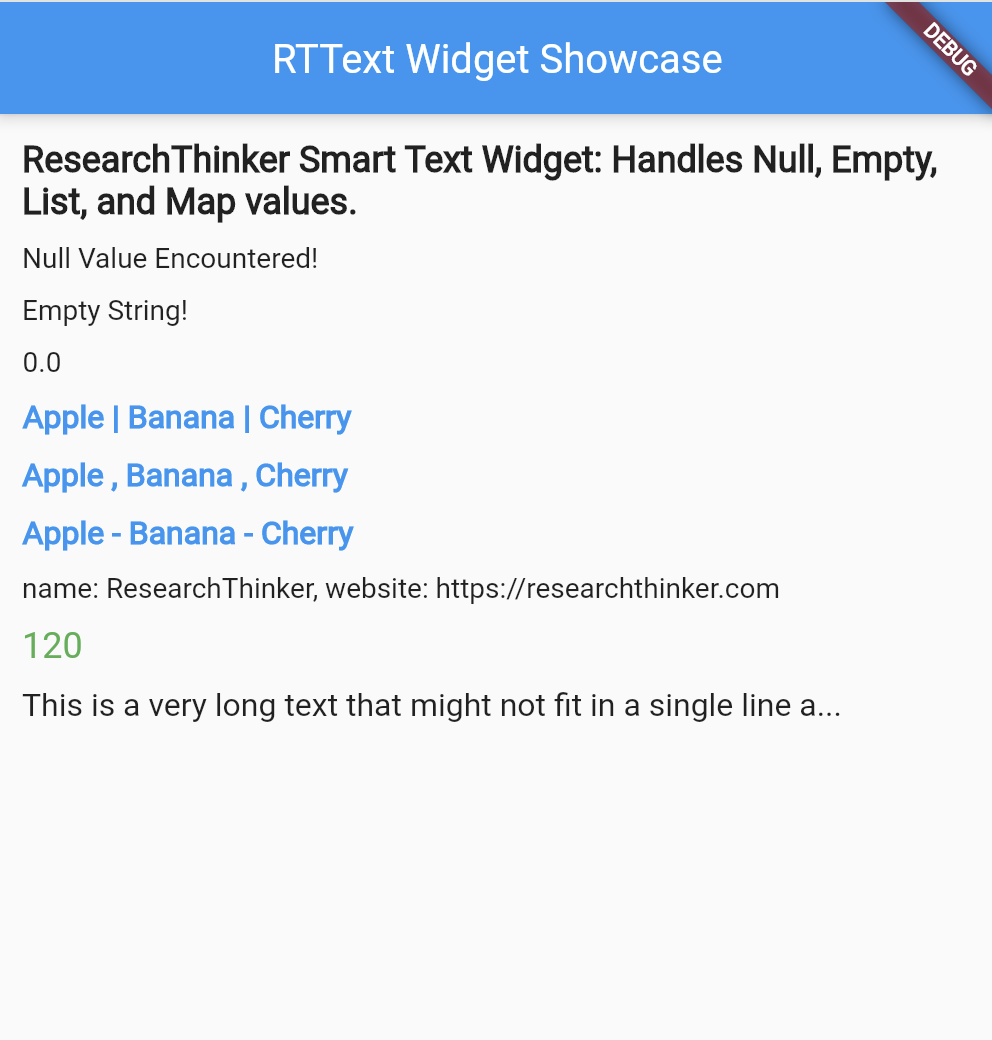
Getting Started #
To use this package, add it to your project by including it in your pubspec.yaml
file:
dependencies:
smart_text_widget: ^0.0.3
Null Value Handling in Text #
The RTText widget can manage null values gracefully. When a null value is passed, you can show a custom error message instead. Here’s how to handle null values effectively:
const RTText(
text: null,
errorTrue: true,
errorValue: "Null Value Encountered!",
truncateEnable: true,
truncateSize: 10,
customEllipsis: "-->",
maxWidth: true,
),
Handling Empty Strings #
If the input is an empty string, you can display a placeholder or a custom message to inform users:
const RTText(
text: "",
errorTrue: true,
errorValue: "No content available!",
maxWidth: true,
),
Displaying Numeric Values #
You can pass numeric values, including integers and decimals, to the RTText widget. The widget will convert them to strings automatically.
const RTText(
text: 42,
maxWidth: true,
),
Handling Lists #
The RTText widget can display lists of strings. Use the scroll parameter to allow scrolling if the list is long.
const RTText(
text: ["Apple", "Banana", "Cherry"],
scroll: true,
listSeparator: " | ",
textAlign: TextAlign.center,
maxWidth: true,
),
Handling Maps #
You can also pass a map to display key-value pairs as text. Customize the error message if needed.
const RTText(
text: {"name": "John", "age": 30},
errorTrue: false,
maxWidth: true,
),
Truncating Long Text #
If your text exceeds a specific length, you can enable truncation. The widget will display an ellipsis (...) after the specified length.
const RTText(
text: "This is a very long text that needs to be truncated after a certain length.",
truncateEnable: true,
truncateSize: 12,
customEllipsis: "...",
maxWidth: true,
),
Scrollable Long Strings #
For long strings that need to be displayed within a specific width, enable scrolling to enhance user experience.
const RTText(
text: "This is a long string that should be scrollable within its container.",
scroll: true,
maxWidth: true,
),
Full Code #
Here's a simple example of how to use SmartText in your Flutter application:
import 'package:flutter/material.dart';
import 'package:smart_text_widget/smart_text_widget.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'RTText Widget Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: Scaffold(
appBar: AppBar(
title: const Text('RTText Widget Showcase'),
),
body: const SingleChildScrollView(
child: Padding(
padding: EdgeInsets.all(12.0),
child: RTTextShowcase(),
),
),
),
);
}
}
class RTTextShowcase extends StatelessWidget {
const RTTextShowcase({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
const Text(
'ResearchThinker Smart Text Widget: Handles Null, Empty, List, and Map values.',
style: TextStyle(fontSize: 18, fontWeight: FontWeight.bold),
),
const SizedBox(height: 10),
// Null Value Example
const RTText(
text: null,
errorTrue: true,
errorValue: "Null Value Encountered!",
truncateEnable: true,
truncateSize: 10,
customEllipsis: "-->",
maxWidth: true,
),
const SizedBox(height: 10),
const RTText( // Empty String Example
text: "",
errorTrue: true,
errorValue: "Empty String!",
maxWidth: true,
),
const SizedBox(height: 10),
const RTText( // Zero Value Example
text: "0.0",
truncateEnable: true,
truncateSize: 4,
textAlign: TextAlign.center,
maxWidth: true,
customEllipsis: " ==> ",
),
const SizedBox(height: 10),
const RTText( // List Example with Separator
text: ["Apple", "Banana", "Cherry"],
scroll: true,
listSeparator: " | ",
textAlign: TextAlign.center,
textStyle: TextStyle(fontSize: 16, color: Colors.blue, fontWeight: FontWeight.bold),
),
const SizedBox(height: 10),
const RTText(
text: ["Apple", "Banana", "Cherry"],
scroll: true,
listSeparator: " , ",
textAlign: TextAlign.center,
textStyle: TextStyle(fontSize: 16, color: Colors.blue, fontWeight: FontWeight.bold),
),
const SizedBox(height: 10),
const RTText(
text: ["Apple", "Banana", "Cherry"],
scroll: true,
listSeparator: " - ",
textAlign: TextAlign.center,
textStyle: TextStyle(fontSize: 16, color: Colors.blue, fontWeight: FontWeight.bold),
),
const SizedBox(height: 10),
const RTText( // Map Example
text: {"name": "ResearchThinker", "website": "https://researchthinker.com"},
errorTrue: false,
maxWidth: true,
emptyPlaceholder: "No data available",
textAlign: TextAlign.right,
),
const SizedBox(height: 10),
const RTText( // Dynamic Value Example
text: 120,
textStyle: TextStyle(color: Colors.green, fontSize: 18),
textAlign: TextAlign.left,
maxWidth: true,
),
const SizedBox(height: 10),
const RTText( // Large String with Scroll and Max Width
text: "This is a very long text that might not fit in a single line and needs to be scrolled or truncated.",
scroll: true,
maxWidth: true,
truncateSize: 62,
truncateEnable: true,
textStyle: TextStyle(fontSize: 16, fontWeight: FontWeight.w400),
textAlign: TextAlign.justify,
),
],
);
}
}
Support and Feedback #
For any queries, suggestions, or feedback, feel free to reach out to us via email or visit our website:
- Email: contact@researchthinker.com
- Website: Visit Research Thinker
If you encounter any issues or have specific requests related to this package, feel free to reach out to us via email or visit our website at ResearchThinker or submit a feature request.
License #
This project is licensed under the MIT License - see the LICENSE file for details.