sky_ui_kit 0.0.2
sky_ui_kit: ^0.0.2 copied to clipboard
A package contains some common widget for faster development.
Overview #
This package contains a set of reusable widget. Provides common widgets that can help to your screen.
Getting Started #
Add dependency to your pubspec.yaml
dependencies:
sky_ui_kit : "^version"
Usage #
Common Widget #
SkyBox #
SkyBox(
borderColor: Colors.grey,
color: Colors.white,
height: 80,
width: 120,
margin: EdgeInsets.zero,
padding: const EdgeInsets.all(8),
borderRadius: BorderRadius.circular(12),
elevation: 9,
child: const SomeWidget(),
onPressed: () {},
);
SkyButton #
Example Button 1
SkyButton(
text: 'Button',
fontSize: 16,
elevation: 4,
borderRadius: 12,
icon: Icons.ac_unit,
iconColor: Colors.white,
wrapContent: true,
borderColor: Colors.yellow,
borderWidth: 3,
onPressed: () {},
),
Example Button 2
SkyButton(
text: 'Button',
iconWidget: const SkyImage(url: 'assets/fork.svg', width: 20),
onPressed: () {}
),
Example Button 3
SkyButton(
text: 'Button',
outlineMode: true,
onPressed: () {},
),
SkyIconButton #
SkyIconButton(
icon: Icons.ac_unit,
size: 80,
iconColor: Colors.black,
color: Colors.orange,
onTap: () {},
);
InfoTable #
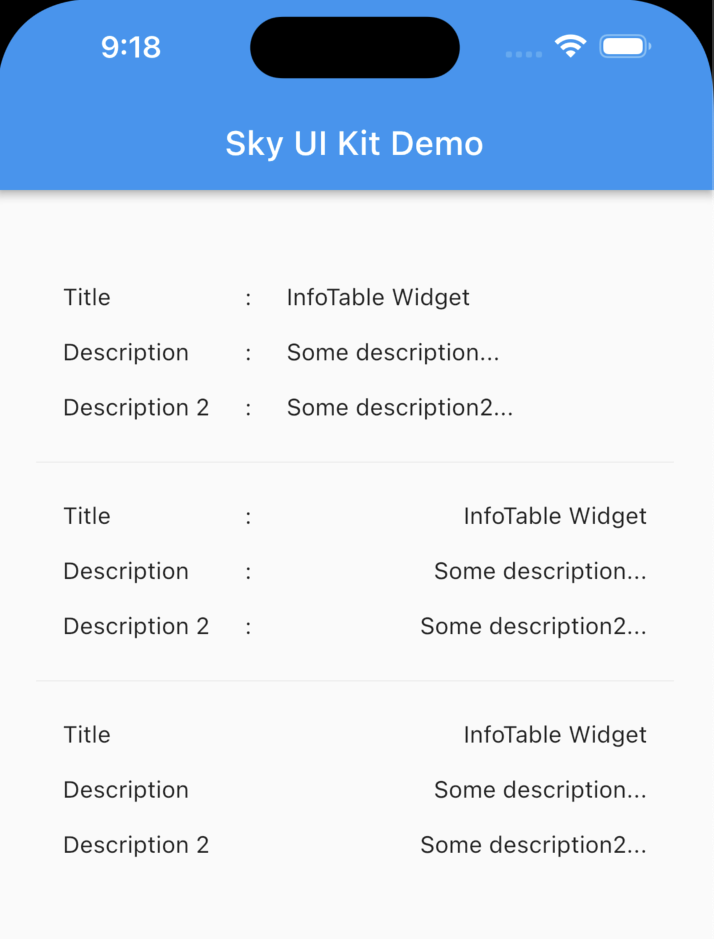
InfoTable(
cellTextAlign: TextAlign.end,
separatorEnabled: false,
data: {
'Title': 'InfoTable Widget',
'Description': 'Some description...',
'Description 2': 'Some description2...',
},
)
KeyboardDismisser #
To use keyboard dismisser you need wrap your parent widget with KeyboardDismisser.
KeyboardDismisser(
child: Scaffold(
appBar: AppBar(
title: const Text('Sky UI Kit Demo'),
),
body: ...
),
),
);
ColoredStatusBar #
To use ColoredStatusBar you need wrap your parent widget it. Even if you use keyboard dismisser, the status bar is still the top of your widget.
ColoredStatusBar(
color: Colors.red,
brightness: Brightness.light,
child: KeyboardDismisser(
child: Scaffold(
appBar: AppBar(
title: const Text('Sky UI Kit Demo'),
),
body: ...
),
),
),
);
CircleIcon #
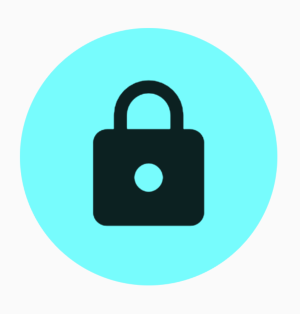
CircleIcon(
icon: Icon(Icons.lock),
backgroundColor: Colors.cyanAccent,
splashColor: Colors.yellow,
size: 54,
onTap: () {},
)
Platform Loading Indicator #
Two style of Loading Indicator in one widget.
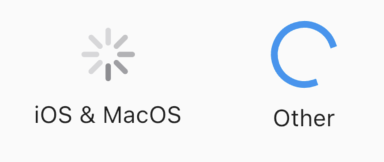
PlatformLoadingIndicator();
Media #
SkyImage #
Use your image source from jpg, png, svg, url, and local path in One Widget.
Handling preview image, empty image, and radius of the image.
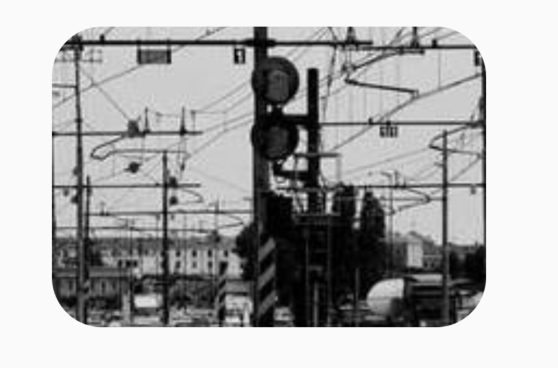
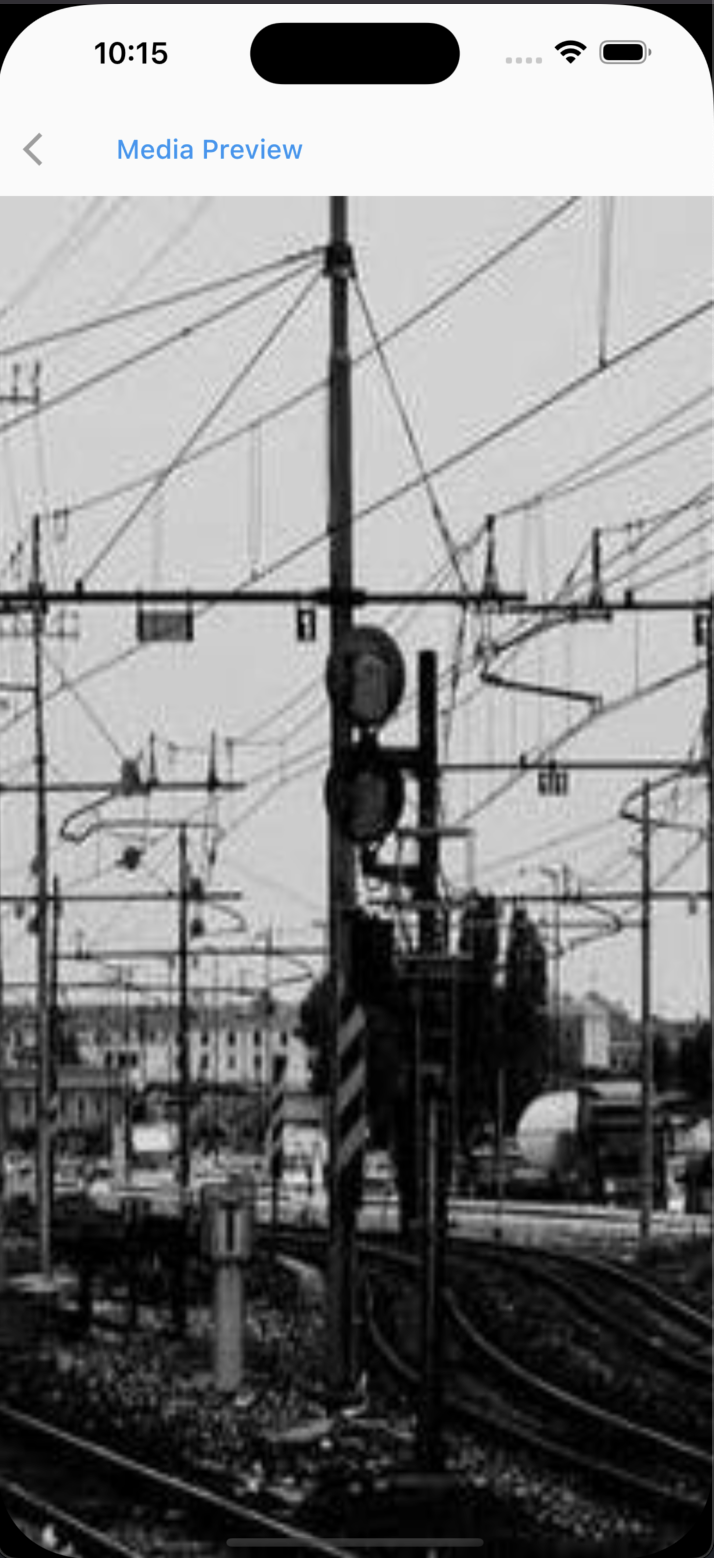
SkyImage(
src: 'https://picsum.photos/200/300.jpg',
width: 260,
height: 180,
borderRadius: BorderRadius.circular(30),
enablePreview: true,
emptyOrNullSrc: 'assets/img_empty.png',
emptyOrNullFit: BoxFit.cover,
)
SkyVideo #
Provides widget that handle video from url or local path
SkyVideo(
url:
'https://flutter.github.io/assets-for-api-docs/assets/videos/bee.mp4',
height: 200,
);
MediaItems #
List
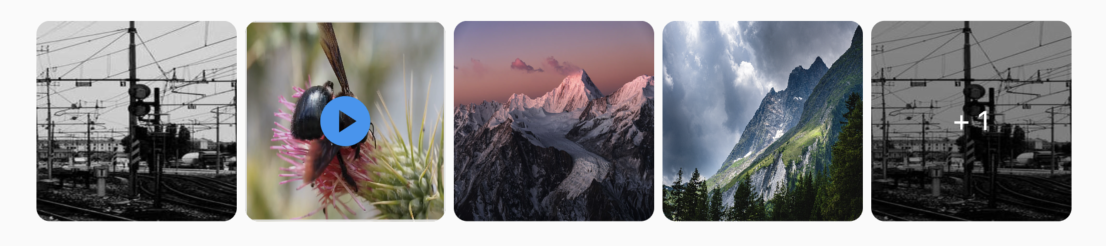
MediaItems(
mediaUrls: [
'https://picsum.photos/200/300.jpg',
'https://flutter.github.io/assets-for-api-docs/assets/videos/bee.mp4',
'https://fujifilm-x.com/wp-content/uploads/2021/01/gfx100s_sample_04_thum-1.jpg',
'https://images.squarespace-cdn.com/content/v1/56169907e4b07744e81fe688/1568573110797-OQQY3Z4EG644FOCS7XQG/Swiss+Alps+-+Mont+Blanc+Massif-1.jpg',
'https://picsum.photos/200/300.jpg',
'assets/img_sample.png',
],
itemsSpacing: 12,
maxItem: 5,
)
Grid
Media item as a grid view
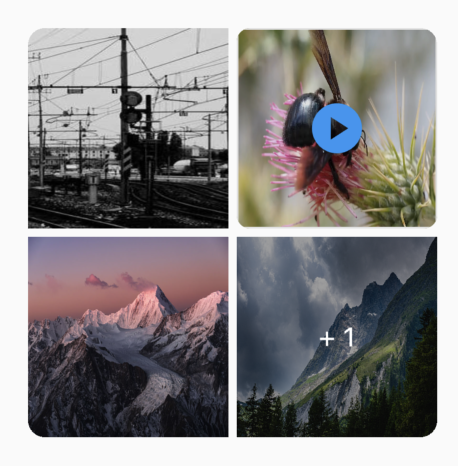
MediaItems(
mediaUrls: [
'https://picsum.photos/200/300.jpg',
'https://flutter.github.io/assets-for-api-docs/assets/videos/bee.mp4',
'https://fujifilm-x.com/wp-content/uploads/2021/01/gfx100s_sample_04_thum-1.jpg',
'https://images.squarespace-cdn.com/content/v1/56169907e4b07744e81fe688/1568573110797-OQQY3Z4EG644FOCS7XQG/Swiss+Alps+-+Mont+Blanc+Massif-1.jpg',
'assets/img_sample.png',
],
maxItem: 4,
isGrid: true,
size: 120,
isSwipePreview: true,
)
Collection View #
In this section (collection view) we use example data especially for GroupedListView.
We give you an example data below.
The sample model data given is :
class CityModel {
String province;
String city;
CityModel(this.province, this.city);
}
And the dummy data list is :
List<CityModel> dataCity = [
CityModel('Jakarta', 'SCBD'),
CityModel('Jakarta', 'Tebet'),
CityModel('Jakarta', 'Gambir'),
CityModel('Lampung', 'Bandar Lampung'),
CityModel('Lampung', 'Pringsewu'),
CityModel('Bandung', 'Setrasari'),
CityModel('Bandung', 'Gedebage'),
CityModel('Bandung', 'Cihanjuang'),
CityModel('Yogyakarta', 'Bantul'),
CityModel('Yogyakarta', 'Sleman'),
];
GroupedListView #
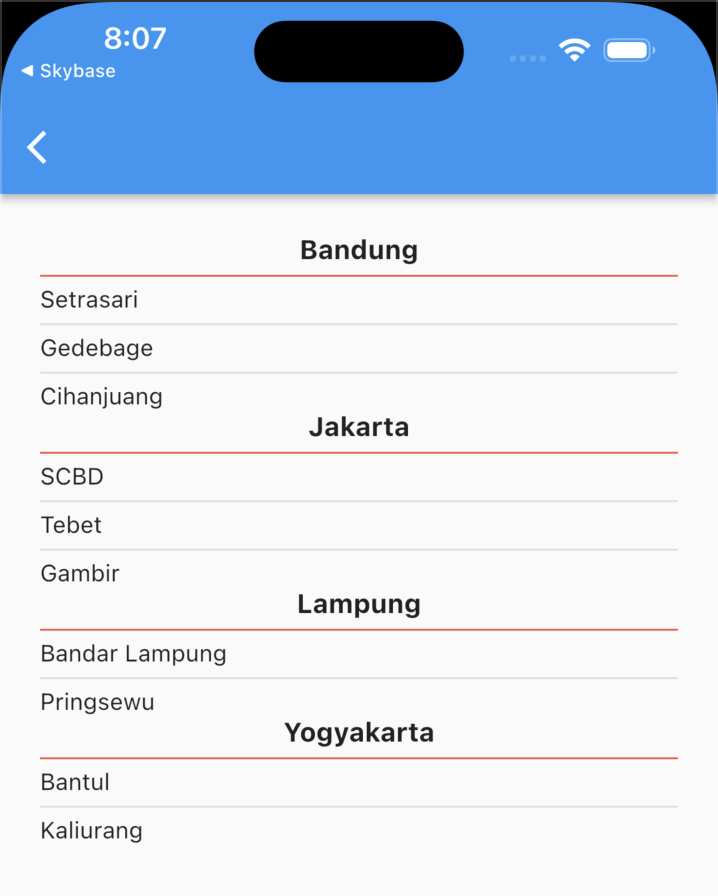
Example code:
SkyGroupedListView<CityModel, String>(
shrinkWrap: true,
physics: const NeverScrollableScrollPhysics(),
sortBy: SortBy.ASC,
data: dataCity,
separator: const Divider(thickness: 1, height: 12),
separatorGroup: const Divider(
thickness: 1,
height: 12,
color: Colors.red,
),
groupHeaderBuilder: (group) {
return Text(
group.toString(),
textAlign: TextAlign.center,
style: const TextStyle(
fontSize: 16,
fontWeight: FontWeight.w700,
),
);
},
itemBuilder: (context, index, item) {
return Text(
item.city,
textAlign: TextAlign.start,
);
},
groupBy: (element) => element.province,
)
OrderedList #
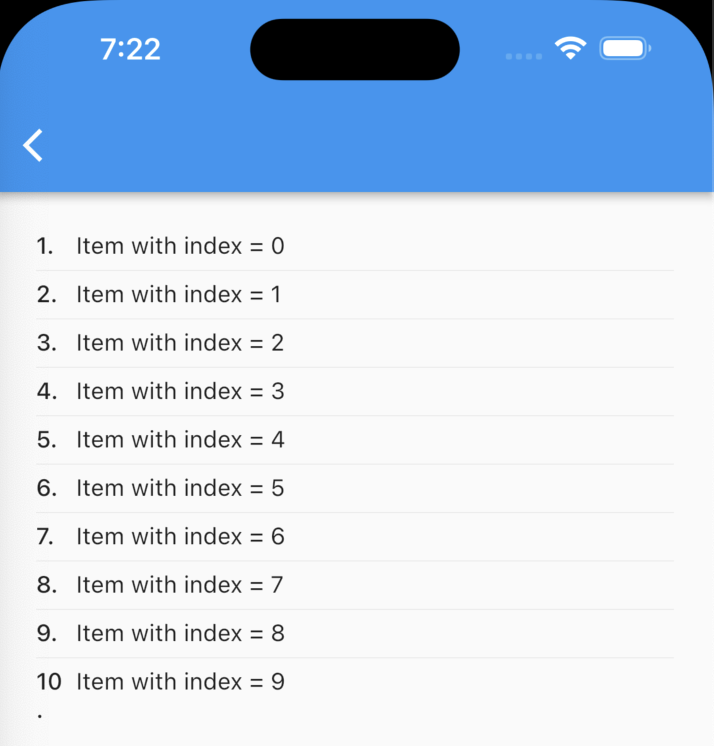
OrderedList(
itemCount: 4,
shrinkWrap: true,
separator: const Divider(height: 12),
itemBuilder: (context, index) {
return Text('Item with index = $index');
},
)
Additional information #
Thank you. Hope this package can help you. Happy coding..