simple_numpad 1.0.5
simple_numpad: ^1.0.5 copied to clipboard
A simple numpad. You can easily change it to your desired design.
Features #
A simple numeric keypad that allows for easy design modifications.
How to use #
Installation #
dependencies:
simple_numpad: ^1.0.2
copied to clipboard
Import #
import 'package:simple_numpad/simple_numpad.dart';
copied to clipboard
Example #
Basic #

SimpleNumpad(
buttonWidth: 80,
buttonHeight: 60,
onPressed: (str) {
print(str);
},
);
copied to clipboard
Circular #

SimpleNumpad(
buttonWidth: 60,
buttonHeight: 60,
gridSpacing: 10,
buttonBorderRadius: 30,
onPressed: (str) {
print(str);
},
);
copied to clipboard
Bordered #

SimpleNumpad(
buttonWidth: 60,
buttonHeight: 60,
gridSpacing: 10,
buttonBorderRadius: 30,
buttonBorderSide: const BorderSide(
color: Colors.black,
width: 1,
),
onPressed: (str) {
print(str);
},
);
copied to clipboard
Backspace & Option #
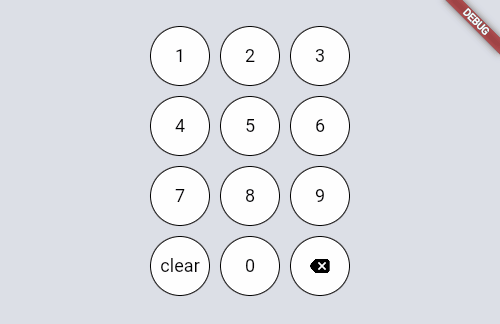
SimpleNumpad(
buttonWidth: 60,
buttonHeight: 60,
gridSpacing: 10,
buttonBorderRadius: 30,
buttonBorderSide: const BorderSide(
color: Colors.black,
width: 1,
),
useBackspace: true,
optionText: 'clear',
onPressed: (str) {
print(str);
},
);
copied to clipboard
Custom1 #

SimpleNumpad(
buttonWidth: 80,
buttonHeight: 60,
gridSpacing: 5,
buttonBorderRadius: 8,
foregroundColor: Colors.white,
backgroundColor: Colors.black.withAlpha(200),
textStyle: const TextStyle(
color: Colors.white,
fontSize: 22,
fontWeight: FontWeight.w400,
),
useBackspace: true,
optionText: 'clear',
onPressed: (str) {
print(str);
};
copied to clipboard
Custom2 #
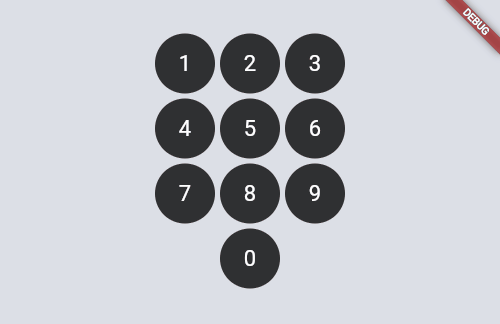
SimpleNumpad(
buttonWidth: 60,
buttonHeight: 60,
gridSpacing: 5,
buttonBorderRadius: 30,
foregroundColor: Colors.white,
backgroundColor: Colors.black.withAlpha(200),
textStyle: const TextStyle(
color: Colors.white,
fontSize: 22,
fontWeight: FontWeight.w400,
),
useBackspace: false,
removeBlankButton: true,
onPressed: (str) {
print(str);
},
);
copied to clipboard
Custom3 #

SimpleNumpad(
buttonWidth: 80,
buttonHeight: 60,
gridSpacing: 0,
buttonBorderRadius: 8,
foregroundColor: Colors.black,
backgroundColor: Colors.transparent,
textStyle: const TextStyle(
color: Colors.black,
fontSize: 22,
fontWeight: FontWeight.w400,
),
useBackspace: false,
removeBlankButton: true,
onPressed: (str) {
print(str);
},
);
copied to clipboard
onPressed function example #
The onPressed function takes input for the string displayed on the Numpad.
Default:
'1', '2', '3', '4', '5', '6', '7', '8', '9', '0'
Optional:
'BACKSPACE', '${optionText}'
void onPressed(String str) {
switch(str) {
case 'BACKSPACE':
// This case is accessible when you have set "useBackspace: true".
removeLast();
break;
case 'clear':
// This string is what you have injected into "optionText".
removeAll();
break;
default:
// '1', '2', '3', '4', '5', '6', '7', '8', '9', '0'
int value = int.parse(str);
append(value);
break;
}
}
copied to clipboard