selection_build 1.0.1
selection_build: ^1.0.1 copied to clipboard
A library that provides custom basic functionality for radio buttons or checkboxes
A library that provides custom basic functionality for radio buttons or checkboxes
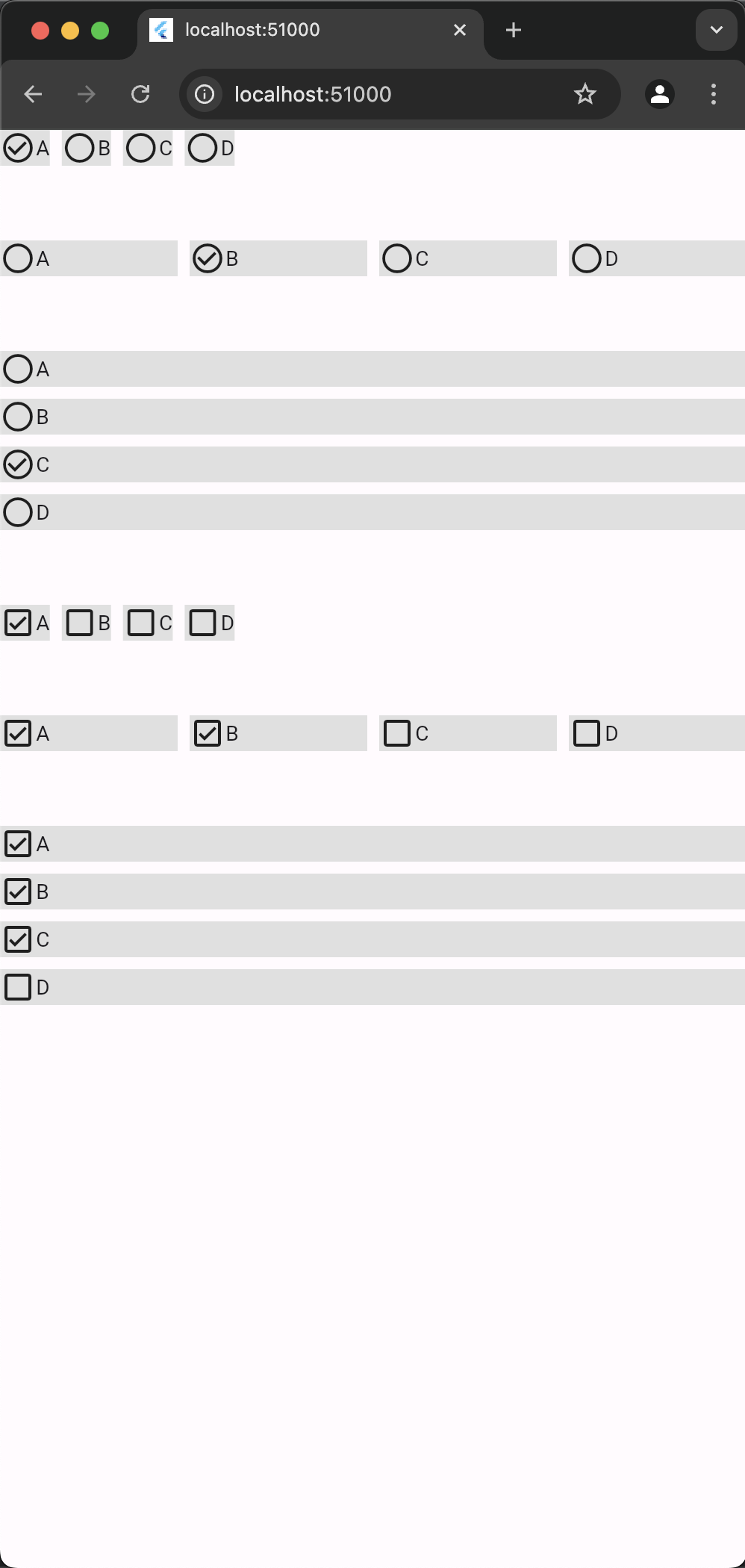
Getting started #
To install, add the following to your pubspec.yaml
file:
dependencies:
selection_build:
copied to clipboard
To import it:
import 'package:selection_build/selection_build.dart';
copied to clipboard
Usage #
This package allows you to create radio buttons or checkboxes to collect values as a list, enum, etc
Example using an enum:
enum Data {
a,
b,
c,
d;
@override
toString() {
switch (this) {
case a:
return 'A';
case b:
return 'B';
case c:
return 'C';
case d:
return 'D';
}
}
}
copied to clipboard
You can customize the item widget by overriding the build method of SingleSelection or MultipleSelection:
class SingleSelectionData extends SingleSelection<Data> {
const SingleSelectionData({
super.key,
required super.data,
required super.onChanged,
super.layout,
});
@override
Widget build(Data item, bool isSelected) =>
Container(
color: Colors.grey.shade300,
child: Row(
mainAxisSize: MainAxisSize.min,
children: [
Icon(
isSelected ? Icons.check_circle_outline : Icons.circle_outlined,
),
Text(item.toString()),
],
),
);
}
class MultipleSelectionData extends MultipleSelection<Data> {
const MultipleSelectionData({
super.key,
required super.data,
required super.onChanged,
super.layout,
});
@override
Widget build(Data item, bool isSelected) =>
Container(
color: Colors.grey.shade300,
child: Row(
mainAxisSize: MainAxisSize.min,
children: [
Icon(
isSelected
? Icons.check_box_outlined
: Icons.check_box_outline_blank,
),
Text(item.toString()),
],
),
);
}
copied to clipboard
Implementation in main():
void main() =>
runApp(MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
body: SafeArea(
child: SingleChildScrollView(
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
SingleSelectionData(
data: Data.values,
onChanged: (item) {},
),
const SizedBox(height: 50),
SingleSelectionData(
data: Data.values,
onChanged: (item) {},
layout: SelectionLayout.row,
),
const SizedBox(height: 50),
SingleSelectionData(
data: Data.values,
onChanged: (item) {},
layout: SelectionLayout.column,
),
const SizedBox(height: 50),
MultipleSelectionData(
data: Data.values,
onChanged: (item) {},
),
const SizedBox(height: 50),
MultipleSelectionData(
data: Data.values,
onChanged: (item) {},
layout: SelectionLayout.row,
),
const SizedBox(height: 50),
MultipleSelectionData(
data: Data.values,
onChanged: (item) {},
layout: SelectionLayout.column,
),
],
),
),
),
),
));
copied to clipboard