plex 0.1.7
plex: ^0.1.7 copied to clipboard
PLEX is Flutter UI framework for enterprise apps with pre-built components and best practices for efficient development in addition with many built in widgets
PLEX #
`PLEX` is a UI framework for Flutter designed specifically for building enterprise applications. It provides a robust and customizable foundation for the entire application, including boilerplate code for main.dart file, routes, screens, and other essential components.
In addition to the basic application structure, PLEX
comes with pre-built components for common UI elements such as tableviews and forms. The tableviews can be easily customized to display data in various formats like lists and grids, while the forms can collect data from users using different
input
types like text fields, dropdowns, and checkboxes.
PLEX
also offers guidelines and best practices for building enterprise applications, including data management, architecture, testing, and more. These guidelines can help developers to build scalable, maintainable, and high-performance applications.
The PLEX
framework is an ideal choice for developers who want to build enterprise-level applications quickly and efficiently, without compromising on quality or customization. Its pre-built components and best practices can save developers a significant amount of time and effort, while also
ensuring
the resulting application meets the high standards of enterprise-level software.
Note:
PLEX
also provide a single click to move from Material 2
to Material 3
and Light Mode
to Dark Mode
.
Screenshots #
Material 3 #
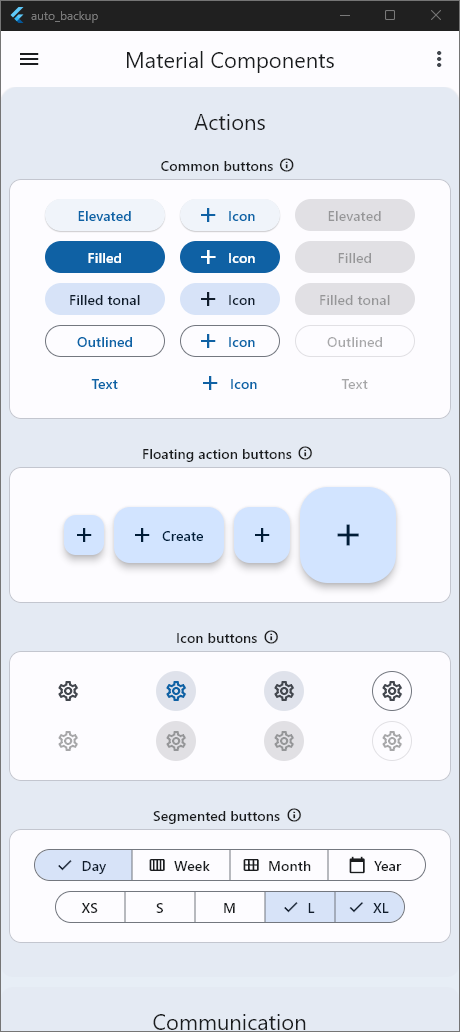
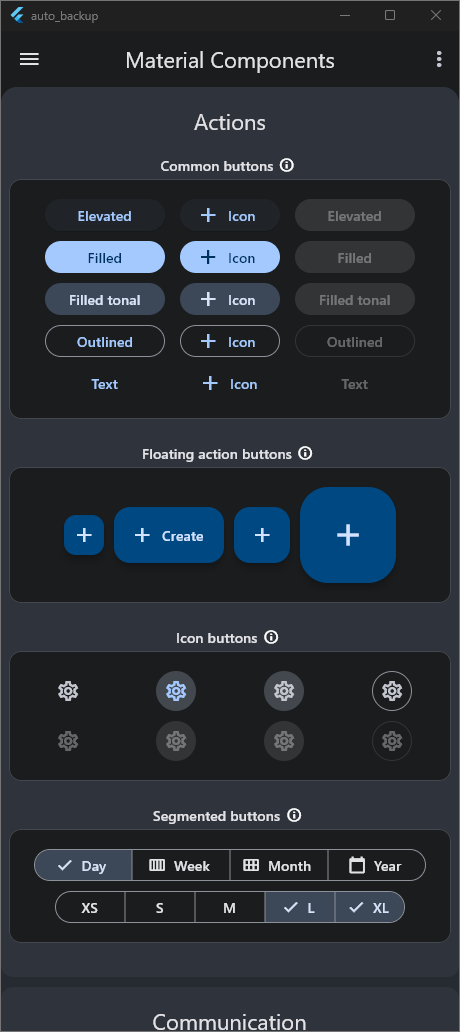
Material 2 #
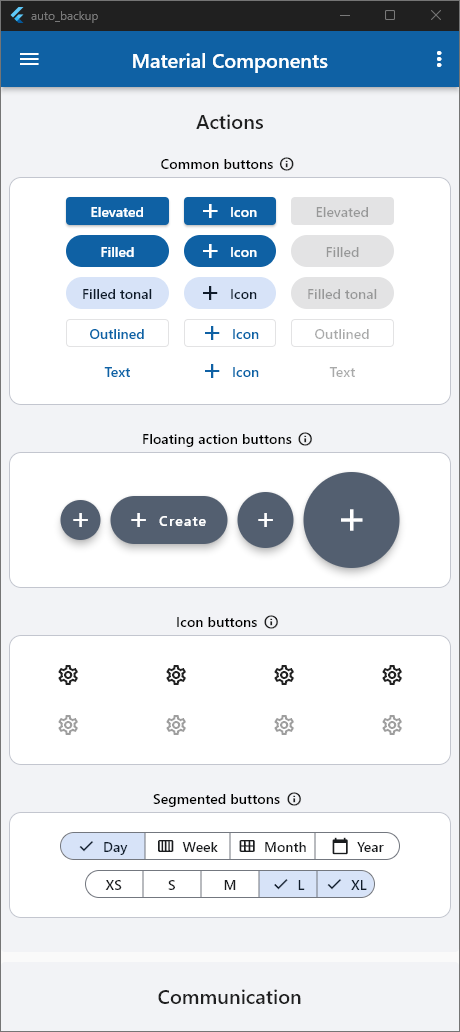
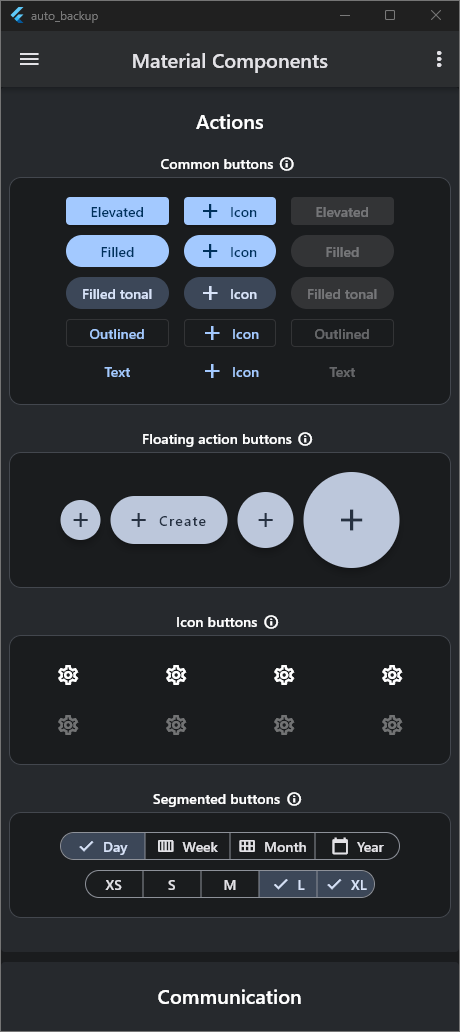
Features #
- Create boilerplate code for an Application
- Built in login screen
- Built in User session manager
- Free useful widgets
- Free useful utilities
- Built in screens and pages
- Builtin form builder from model class
Getting started #
Install the plex
in your application.
Usage #
Widgets #
- PlexWidget
- PlexDataTable
- PlexInputWidget
- PlexFormWidget
- PlexInputWidget
PlexInputWidget
Usage
// Input Types
// PlexInputWidget.typeInput
// PlexInputWidget.typeDropdown
// PlexInputWidget.typeDate
// PlexInputWidget.typeButton
PlexInputWidget(
title: "Username / Email",
type: PlexInputWidget.typeInput,
inputHint: "Enter Your Email or Username",
inputController: usernameController,
inputOnChange: (value) {},
inputOnSubmit: (value) {},
inputAction: TextInputAction.go,
inputKeyboardType: TextInputType.name,
isPassword: false,
dropdownItemOnSelect: (item) {},
dropdownItemAsString: (item) => item.toString(),
dropdownItems: const ["Data"],
dropdownAsyncItems: Future(() => ["Data"]),
dropdownSelectionController: PlexWidgetController(),
dropDownLeadingIcon: (item) => const Icon(Icons.add),
dropdownItemWidget: (item) => const Text("Data"),
dropdownOnSearch: (query, item) { return true; },
dropdownCustomOnTap: () {},
buttonClick: ,
buttonIcon: ,
buttonColor: ,
useMargin: ,
margin: ,
fieldColor: ,
editable: ,
helperText: ,
)
Persistent Storage
//Only initialize if you are not using PlexApp
//and using PlexDb separately
PlexDb.initialize();
PlexDb.instance.setString("Key", "Value");
PlexDb.instance.getString("Key");
PlexDb.instance.setBool("Key", true);
PlexDb.instance.getBool("Key");
Messaging #
BuildContext context;
context.showSnackBar("Your Message...");
void main() {
WidgetsFlutterBinding.ensureInitialized();
runApp(PlexApp(
themeFromColor: const Color(0xFF26A9E1),
//themeFromImage: const AssetImage('assets/images/logo.png'),
//You can also use image to load theme by image color pallets but dont use both at the same time
appLogo: Image.asset(Assets.assetsLogo),
title: 'Application Name',
initialRoute: '/dashboard',
//Optional
useAuthorization: true,
//Optional Note: But required when useAuthorization is true
loginConfig: PlexLoginConfig(
additionalWidgets: Container(),
onLogin: (email, password) async {
//Chek user credentials
//If anything wrong return null
//If authentication is successfull return a PlexUser object
//or subclass of PlexUser
return PlexUser({
"Key": "Value",
});
}),
routes: [
//Use plex Route to add another screen in the application
PlexRoute(
route: '/dashboard',
category: "Tables",
title: "Data Table",
logo: const Icon(Icons.account_balance_outlined),
//Return a widget that will be displayed when this page or route is called
screen: (key, context) =>
Container(
//This PlexDataTable usage will show how you can show a data table using plex
child: PlexDataTable(
headerTextStyle: const TextStyle(fontWeight: FontWeight.bold),
headerBackground: Colors.red,
alternateColor: Colors.lightGreen,
columns: [
PlexDataCell.text("Id"),
PlexDataCell.text("First Name"),
PlexDataCell.text("Last Name"),
PlexDataCell.text("Emp Code"),
PlexDataCell.text("Designation"),
PlexDataCell.text("Grade"),
PlexDataCell.text("Company"),
],
rows: [
[
PlexDataCell.text("1"),
PlexDataCell.text("Abdur"),
PlexDataCell.text("Rahman"),
PlexDataCell.text("EMP953346RT"),
PlexDataCell.text("Software Engineer"),
PlexDataCell.text("Grade / Scale"),
PlexDataCell.custom(
"Company Pvt. Ltd",
const DataCell(
Text("Company Pvt. Ltd", style: TextStyle(color: Colors.lime)),
),
),
],
[
PlexDataCell.text("1"),
PlexDataCell.text("Abdur"),
PlexDataCell.text("Rahman"),
PlexDataCell.text("EMP953346RT"),
PlexDataCell.text("Software Engineer"),
PlexDataCell.text("Grade / Scale"),
PlexDataCell.custom(
"Company Pvt. Ltd",
const DataCell(
Text("Company Pvt. Ltd", style: TextStyle(color: Colors.lime)),
),
)
],
],
),
),
),
//Other Screens
PlexRoute(
route: '/settings',
category: "Settings",
title: "Settings",
logo: const Icon(Icons.settings),
screen: (key, context) => Container(),
),
],
));
}