modular_di 0.2.1
modular_di: ^0.2.1 copied to clipboard
A simple way to organize dependency injection using modules.
Modular DI (Dependency Injection)
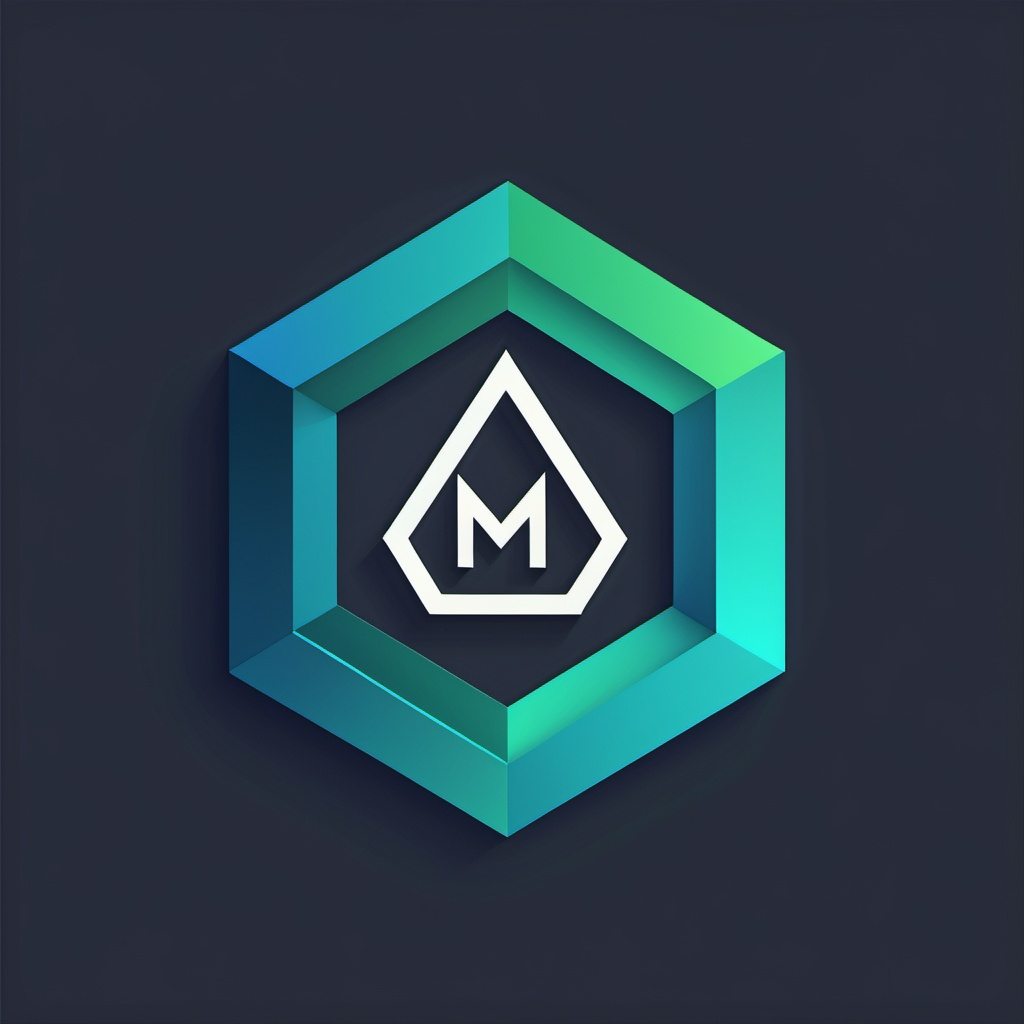
⚠️ DEPRECATED ⚠️
This package is deprecated #
This package is no longer maintained. Please use flutter_easy_di instead.
Migration #
To migrate to the new package, update your pubspec.yaml
:
dependencies:
flutter_easy_di: <last version> # Use this package instead of modular_di
Then follow the documentation for flutter_easy_di to update your code.
Legal stuff #
This project uses the MIT License - check out LICENSE if you're into that kind of thing.