locale_switcher 0.9.4
locale_switcher: ^0.9.4 copied to clipboard
A widget for switching the locale of your application with 2 lines of code.
locale_switcher #
A widget for switching the locale of your application.
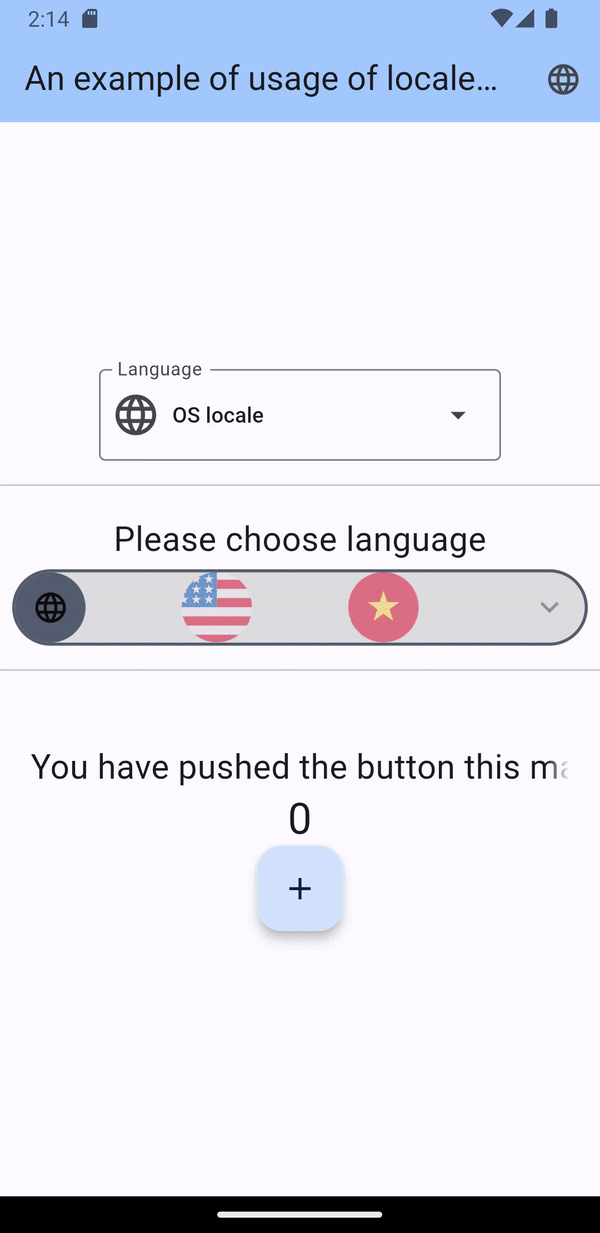
Content #
About #
This package allows you to add locale-switching functionality to your app with just 2 lines of code.
This is NOT a localization package, it is just a few useful widgets that extend functionality of localization systems, such as: intl, easy_localization, etc...
Features #
-
LocaleManager widget:
- optionally: load/stores the last selected locale in
SharedPreferences
, - update locale of app (listen to
notifier
and rebuildMaterialApp
), - observes changes in the system locale,
- optionally: load/stores the last selected locale in
-
LocaleSwitcher - a widget to switch locale:
- show a list of locales and special option to use system locale,
- constructor LocaleSwitcher.toggle,
- constructor LocaleSwitcher.segmentedButton,
- constructor LocaleSwitcher.menu,
- constructor LocaleSwitcher.custom,
- constructor LocaleSwitcher.iconButton - button/indicator which open popup window to select locale,
-
Some other helpers...
Usage #
- Wrap
MaterialApp
orCupertinoApp
with LocaleManager:
@override
Widget build(BuildContext context, WidgetRef ref) {
return LocaleManager(
child: MaterialApp(
locale: LocaleManager.locale.value,
supportedLocales: AppLocalizations.supportedLocales,
localizationsDelegates: AppLocalizations.localizationsDelegates,
//...
- Add LocaleSwitcher widget anywhere into your app.
Troubleshooting #
Note: localization should be set up before you start to use this package, if there some problems - please, check next section and/or documentation of localization system you use, before reporting bug.
Check that intl package is setup correctly: #
The following instructions are from intl package, so you probably already did them:
In pubspec.yaml
:
dependencies: # in this section
intl:
flutter_localizations:
sdk: flutter
dev_dependencies: # in this section
build_runner:
flutter: # in this section
generate: true
Optionally - in l10n.yaml:
arb-dir: lib/src/l10n
template-arb-file: intl_en.arb
output-dir: lib/src/l10n/generated
output-localization-file: app_localizations.dart
untranslated-messages-file: desiredFileName.txt
preferred-supported-locales: [ "en", "vi", "de" ]
nullable-getter: false
Example #
Another example code: LocaleSwitcher used without LocaleManager(not recommended).
Example with dynamic option switch: here.
TODO: #
- ❌ Test with other localization system (currently: tested only intl)
- ❌ Support slang
- ❌ Rectangle flags
- ❌ reduce assets: use flutter_svg: format .vec? and code_gen to add assets
FAQ #
- How to change order of languages?
Languages are shown in the same order as they listed in l10n.yaml.
- How to change flag of language?
Use LocaleManager.reassign
parameter like this:
LocaleManager(
reassign: {'en': ['GB', 'English', <Your_icon_optional>]}
// (first two options are required, third is optional)
// first option is the code of country which flag you want to use
...
)
- How to use localization outside of MaterialApp
(or CupertinoApp)?
Here is a useful example, although it is not depend on this package:
import 'package:flutter_gen/gen_l10n/app_localizations.dart';
/// Access localization through locale
extension LocaleWithDelegate on Locale {
/// Get class with translation strings for this locale.
AppLocalizations get tr => lookupAppLocalizations(this);
}
Locale("en").tr.example
// or
LocaleManager.locale.value.tr.example