keyboard_detection 0.7.3
keyboard_detection: ^0.7.3 copied to clipboard
This plugin gives you an easy way to detect the keyboard visibility.
Keyboard Detection #
This plugin gives you an easy way to detect if the keyboard is visible or not. It uses the resizing of the bottom view inset to check the the keyboard visibility, so it's native to flutter.
Introduction #
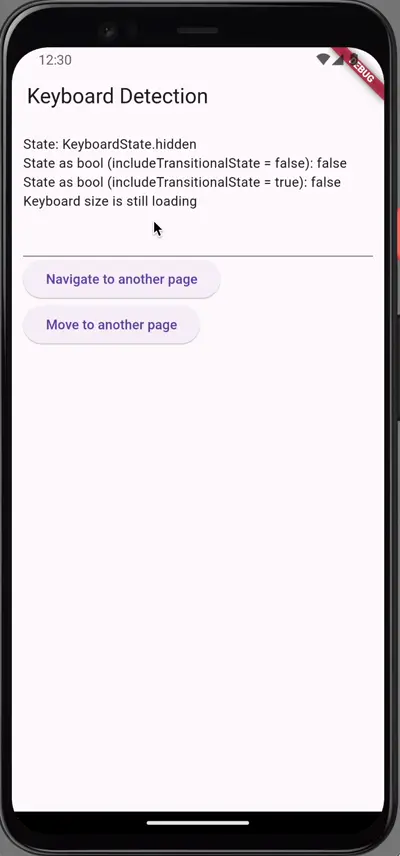
Simple Usage #
You just need to wrap the Scaffold
with KeyboardDetection
like below and listen to onChanged
value.
@override
Widget build(BuildContext context) {
return MaterialApp(
home: KeyboardDetection(
controller: KeyboardDetectionController(
onChanged: (value) {
print('Keyboard visibility onChanged: $value');
setState(() {
keyboardState = value;
stateAsBool = keyboardDetectionController.stateAsBool();
stateAsBoolWithParamTrue =
keyboardDetectionController.stateAsBool(true);
});
},
),
child: Scaffold(
appBar: AppBar(
title: const Text('Keyboard Detection'),
),
body: Center(
child: Column(
children: [
Text('State: $keyboardState'),
Text(
'State as bool (includeTransitionalState = false): $stateAsBool'),
Text(
'State as bool (includeTransitionalState = true): $stateAsBoolWithParamTrue'),
const TextField(),
],
),
),
),
),
);
}
onChanged
will be returned in enum KeyboardState
(unknown
: unknown, visibling
: visibling, visible
: visible, hiding
: hiding, hidden
: hidden).
Usage With Controller #
You can declare the controller
outside the build
method like below:
late KeyboardDetectionController keyboardDetectionController;
@override
void initState() {
keyboardDetectionController = KeyboardDetectionController(
onChanged: (value) {
print('Keyboard visibility onChanged: $value');
setState(() {
keyboardState = value;
stateAsBool = keyboardDetectionController.stateAsBool();
stateAsBoolWithParamTrue =
keyboardDetectionController.stateAsBool(true);
});
},
);
// Listen to the stream
_sub = keyboardDetectionController.stream.listen((state) {
print('Listen to onChanged with Stream: $state');
});
// One time callback
keyboardDetectionController.addCallback((state) {
print('Listen to onChanged with one time Callback: $state');
// End this callback by returning false
return false;
});
// Looped callback
keyboardDetectionController.addCallback((state) {
print('Listen to onChanged with looped Callback: $state');
// This callback will be looped
return true;
});
// Looped with future callback
keyboardDetectionController.addCallback((state) async {
await Future.delayed(const Duration(milliseconds: 100));
print('Listen to onChanged with looped future Callback: $state');
// This callback will be looped
return true;
});
super.initState();
}
and add it to controller
inside build
method:
@override
Widget build(BuildContext context) {
return KeyboardDetection(
controller: keyboardDetectionController,
child: Scaffold(
appBar: AppBar(
title: const Text('Keyboard Detection'),
),
body: Center(
child: Padding(
padding: const EdgeInsets.all(12.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text('State: $keyboardState'),
Text('State as bool (includeTransitionalState = false): $stateAsBool'),
Text('State as bool (includeTransitionalState = true): $stateAsBoolWithParamTrue'),
FutureBuilder(
future: keyboardDetectionController.ensureKeyboardSizeLoaded,
builder: (context, snapshot) {
if (snapshot.hasData) {
return Text(
'Keyboard size is loaded with size: ${keyboardDetectionController.keyboardSize}');
}
return const Text('Keyboard size is still loading');
},
),
const TextField(),
ElevatedButton(
onPressed: () {
Navigator.push(
context,
MaterialPageRoute(
builder: (_) => const MyApp(),
),
);
},
child: const Text('Navigate to another page'),
),
ElevatedButton(
onPressed: () {
Navigator.pushAndRemoveUntil(
context,
MaterialPageRoute(
builder: (_) => const MyApp(),
),
(_) => false);
},
child: const Text('Move to another page'),
)
],
),
),
),
),
);
}
You can get the current state of the keyboard visibility by using:
-
keyboardDetectionController.state
: the current state of the keyboard visibility return in enumKeyboardState
(unknown
: unknown,visibling
: visibling,visible
: visible,hiding
: hiding,hidden
: hidden). -
keyboardDetectionController.stateAsBool([bool includeTransitionalState = false])
: the current state of the keyboard visibility return inbool?
(null
: unknown,true
: visible,false
: hidden). If theincludeTransitionalState
istrue
than it will returntrue
even when thestate
isvisibling
andfalse
when it'shiding
. -
keyboardDetectionController.addCallback(callback)
to add a callback to be called when the keyboard state is changed. If the callback returnstrue
then it will be called eachtime the keyboard is changed, iffalse
then it will be ignored. Thiscallback
also supports theFuture
method. -
keyboardDetectionController.stream
to listen for keyboard visibility changing events inKeyboardState
. -
keyboardDetectionController.size
to get the keyboard size. Please notice that this value may returns 0 even when the keyboard state is visible because the keyboard needs time to show up completely. So that, you should callkeyboardDetectionController.isSizeLoaded
to checks that the keyboard size is loaded or not. From version0.5.0
, you can wait for this value by usingawait keyboardDetectionController.ensureSizeLoaded
.
Limitations #
- This package uses the bottom inset to detect the keyboard visibility so it doesn't work with the floating keyboard (Issue: #1)