json_traverse 0.0.2
json_traverse: ^0.0.2 copied to clipboard
Parse deeply nested JSON using simple query strings with dot separator.
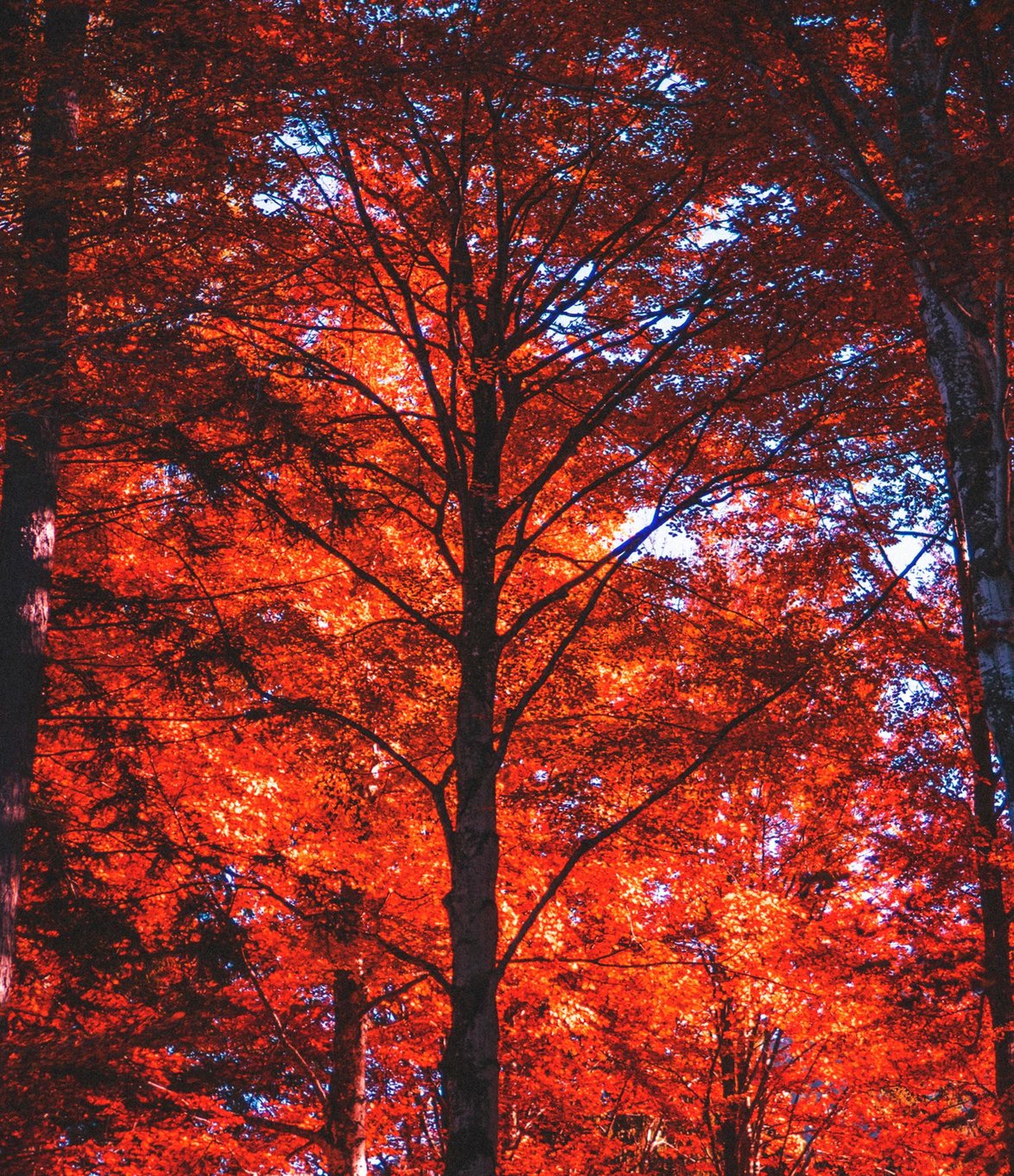
JSON Traverser
Traverse a deeply nested JSON with a query string
Photo by Simone Dalmeri on Unsplash
import 'package:json_traverse/json_traverse.dart';
void main() {
var jsonString = '''
{
"name": "John Smith",
"email": "john@example.org",
"contact": [
"123",
"456"
]
}
''';
var traverser = JSONTraverse(jsonString);
// for a single string
print(traverser.query('name')); // Prints `John Smith`
// for an array, point the index
print(traverser.query('contact.1')); // Prints `456`
}
Usage #
First instantiate a JSONTraverse
object: JSONTraverse traverser = JSONTraverse(jsonString)
.
Now, use the query
method to traverse through the JSON: traverser.query('name')
.
If you want to traverse through an array, you have to mention the index, e.g.,
traverser.query('contact.1')
for index 1
of the contact array. If the index
is not a valid number, the code will throw a FormatException
.