giphy_get 3.0.0-pre.1
giphy_get: ^3.0.0-pre.1 copied to clipboard
Pick EMOJI,STICKER or GIF from Giphy in pure dart code. Support Android,iOS,Web and Desktop.
giphy_get #
Overview #
This package allow to get gifs, sticker or emojis from GIPHY in pure dart code using Giphy SDK design guidelines.
Inspiration #
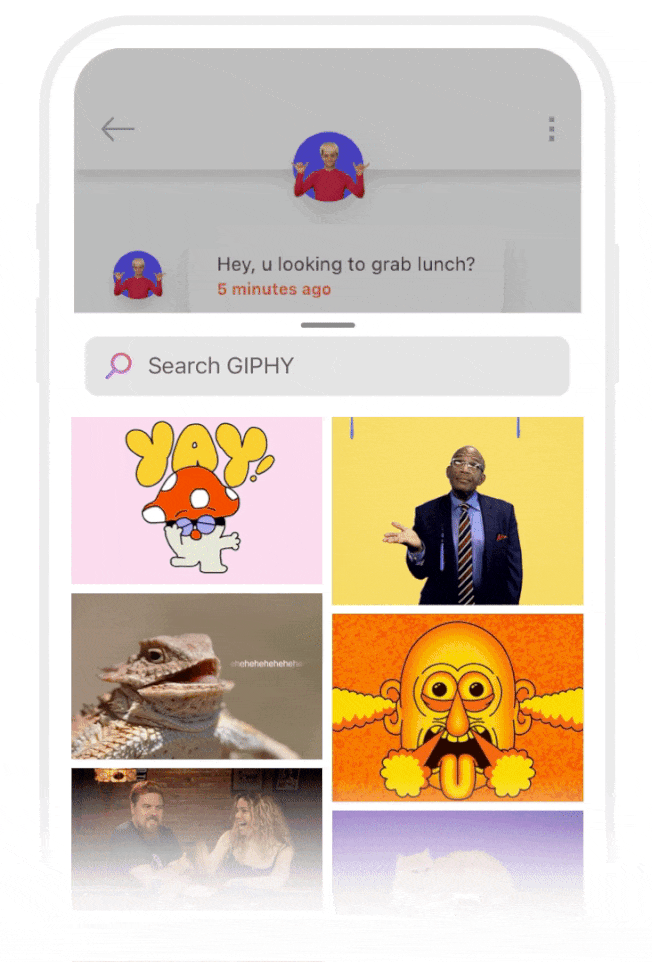
Result #

Getting Started #
Important! you must register your app at Giphy Develepers and get your APIKEY
import 'package:giphy_get/giphy_get.dart';
GiphyGif gif = await GiphyGet.getGif(
context: context, //Required
apiKey: "your api key HERE", //Required.
lang: GiphyLanguage.english, //Optional - Language for query.
randomID: "abcd", // Optional - An ID/proxy for a specific user.
tabColor:Colors.teal, // Optional- default accent color.
);
Options #
Value | Type | Description | Default |
---|---|---|---|
lang |
String | Use ISO 639-1 language code or use GiphyLanguage constants | GiphyLanguage.english |
randomID |
String | An ID/proxy for a specific user. | null |
searchText |
String | Input search hint, we recomend use flutter_18n package for translation | "Search GIPHY" |
tabColor |
Color | Color for tabs and loading progress, | Theme.of(context).accentColor |
Get Random ID #
GiphyClient giphyClient = GiphyClient(apiKey: "YOUR API KEY");
String randomId = await giphyClient.getRandomId();
Localizations #
Currently english and spanish is supported.
return MaterialApp(
title: 'Giphy Get Demo',
localizationsDelegates: [
// Default Delegates
GlobalMaterialLocalizations.delegate,
GlobalWidgetsLocalizations.delegate,
// Add this line
GiphyGetUILocalizations.delegate
],
supportedLocales: [
//Your supported languages
Locale('en', ''),
Locale('es', ''),
],
home: MyHomePage(title: 'Giphy Get Demo'),
themeMode: Provider.of<ThemeProvider>(context).currentTheme,
);
Widgets #
Optional but this widget is required to get more gif's of user or view on Giphy following Giphy Design guidelines and return Gif's with stream
GiphyGifWidget #
Params
Value | Type | Description | Default |
---|---|---|---|
gif required |
GiphyGif | GiphyGif object from stream or JSON | null |
giphyGetWrapper required |
GiphyGetWrapper instance required for tap to more | ||
showGiphyLabel | boolean | show or hide Powered by GIPHY label at bottom |
true |
borderRadius | BorderRadius ex: BorderRadius.circular(10) | add border radius to image | null |
imageAlignment | Alignment | this widget is a STACK with Image and tap buttons this property adjust alignment | Alignment.center |
GiphyGetWrapper #
Params
Value | Type | Description | Default |
---|---|---|---|
giphy_api_key required |
String | Your Giphy API KEY | null |
builder |
function | return Stream<GiphyGif> and Instance of GiphyGetWrapper |
Methods #
void getGif(String queryText,BuildContext context)
return GiphyGetWrapper(
giphy_api_key: REPLACE_WITH YOUR_API_KEY,
// Builder with Stream<GiphyGif> and Instance of GiphyGetWrapper
builder: (stream, giphyGetWrapper) => StreamBuilder<GiphyGif>(
stream: stream,
builder: (context, snapshot) {
return Scaffold(
body: snapshot.hasData
? SizedBox(
// GiphyGifWidget with tap to more
child: GiphyGifWidget(
imageAlignment: Alignment.center,
gif: snapshot.data,
giphyGetWrapper: giphyGetWrapper,
borderRadius: BorderRadius.circular(30),
showGiphyLabel: true,
),
)
: Text("No GIF"),
floatingActionButton: FloatingActionButton(
onPressed: () async {
//Open Giphy Sheet
giphyGetWrapper.getGif('', context);
},
tooltip: 'Open Sticker',
child: Icon(Icons
.insert_emoticon)),
);
})
});
Contrib #
Feel free to make any PR's