flutter_paytabs_bridge 2.1.5
flutter_paytabs_bridge: ^2.1.5 copied to clipboard
Flutter plugin for Paytabs.
Flutter PayTabs Bridge #
Flutter paytabs plugin is a wrapper for the native PayTabs Android and iOS SDKs, It helps you integrate with PayTabs payment gateway.
Plugin Support:
- ✅ iOS
- ✅ Android
Installation #
dependencies:
flutter_paytabs_bridge: ^2.1.5`
Usage #
import 'package:flutter_paytabs_bridge/BaseBillingShippingInfo.dart';
import 'package:flutter_paytabs_bridge/PaymentSdkConfigurationDetails.dart';
import 'package:flutter_paytabs_bridge/PaymentSdkLocale.dart';
import 'package:flutter_paytabs_bridge/PaymentSdkTokenFormat.dart';
import 'package:flutter_paytabs_bridge/PaymentSdkTokeniseType.dart';
import 'package:flutter_paytabs_bridge/flutter_paytabs_bridge.dart';
import 'package:flutter_paytabs_bridge/IOSThemeConfiguration.dart';
import 'package:flutter_paytabs_bridge/PaymentSdkTransactionClass.dart';
Pay with Card #
- Configure the billing & shipping info, the shipping info is optional
var billingDetails = new BillingDetails("billing name",
"billing email",
"billing phone",
"address line",
"country",
"city",
"state",
"zip code");
var shippingDetails = new ShippingDetails("shipping name",
"shipping email",
"shipping phone",
"address line",
"country",
"city",
"state",
"zip code");
- Create object of
PaymentSDKConfiguration
and fill it with your credentials and payment details.
var configuration = PaymentSdkConfigurationDetails(
profileId: "profile id",
serverKey: "your server key",
clientKey: "your client key",
cartId: "cart id",
cartDescription: "cart desc",
merchantName: "merchant name",
screentTitle: "Pay with Card",
billingDetails: billingDetails,
shippingDetails: shippingDetails,
locale: PaymentSdkLocale.EN, //PaymentSdkLocale.AR or PaymentSdkLocale.DEFAULT
amount: "amount in double",
currencyCode: "Currency code",
merchantCountryCode: "2 chars iso country code");
Options to show billing and shipping info
configuration.showBillingInfo = true;
configuration.showShippingInfo = true;
- Set merchant logo from the project assets:
- create 'assets' directory and put the image inside it.
- be sure you add in the Runner iOS Project in the infor.plist the image usage description.
- under flutter section in the pubspec.yaml declare your logo.
flutter:
assets:
- assets/logo.png
- be sure you pass the image path like this:-
var configuration = PaymentSdkConfigurationDetails();
var theme = IOSThemeConfigurations();
theme.logoImage = "assets/logo.png";
configuration.iOSThemeConfigurations = theme;
- Start payment by calling
startCardPayment
method and handle the transaction details
FlutterPaytabsBridge.startCardPayment(configuration, (event) {
setState(() {
if (event["status"] == "success") {
// Handle transaction details here.
var transactionDetails = event["data"];
print(transactionDetails);
} else if (event["status"] == "error") {
// Handle error here.
} else if (event["status"] == "event") {
// Handle events here.
}
});
});
Pay with Apple Pay #
-
Follow the guide Steps to configure Apple Pay to learn how to configure ApplePay with PayTabs.
-
Do the steps 1 and 2 from Pay with Card although you can ignore Billing & Shipping details and Apple Pay will handle it, also you must pass the merchant name and merchant identifier.
var configuration = PaymentSdkConfigurationDetails(
profileId: "profile id",
serverKey: "your server key",
clientKey: "your client key",
cartId: "cart id",
cartDescription: "cart desc",
merchantName: "merchant name",
screentTitle: "Pay with Card",
locale: PaymentSdkLocale.AR, //PaymentSdkLocale.EN or PaymentSdkLocale.DEFAULT
amount: "amount in double",
currencyCode: "Currency code",
merchantCountryCode: "2 chars iso country code",
merchantApplePayIndentifier: "merchant.com.bundleID",
);
- To simplify ApplePay validation on all user's billing info, pass simplifyApplePayValidation parameter in the configuration with true.
configuration.simplifyApplePayValidation = true;
- Call
startApplePayPayment
to start payment
FlutterPaytabsBridge.startApplePayPayment(configuration, (event) {
setState(() {
setState(() {
if (event["status"] == "success") {
// Handle transaction details here.
var transactionDetails = event["data"];
print(transactionDetails);
} else if (event["status"] == "error") {
// Handle error here.
} else if (event["status"] == "event") {
// Handle events here.
}
});
});
});
Pay with Samsung Pay #
Pass Samsung Pay token to the configuration and call startSamsungPayPayment
configuration.samsungToken = "{Json token returned from the samsung pay payment}"
Pay with Alternative Payment Methods #
It becomes easy to integrate with other payment methods in your region like STCPay, OmanNet, KNet, Valu, Fawry, UnionPay, and Meeza, to serve a large sector of customers.
- Do the steps 1 and 2 from Pay with Card
- Choose one or more of the payment methods you want to support
.alternativePaymentMethods(list Of PaymentSdkApms) // add the Payment Methods you want to the list
Theme IOS #
Use the following guide to cusomize the colors, font, and logo by configuring the theme and pass it to the payment configuration.
var theme = IOSThemeConfigurations();
theme.backgroundColor = "e0556e"; // Color hex value
configuration.iOSThemeConfigurations = theme;
Theme Android #
Use the following guide to customize the colors, font, and logo by configuring the theme and pass it to the payment configuration.
-- Override strings
To override string you can find the keys with the default values here
<resourse>
// to override colors
<color name="payment_sdk_primary_color">#5C13DF</color>
<color name="payment_sdk_secondary_color">#FFC107</color>
<color name="payment_sdk_primary_font_color">#111112</color>
<color name="payment_sdk_secondary_font_color">#6D6C70</color>
<color name="payment_sdk_separators_color">#FFC107</color>
<color name="payment_sdk_stroke_color">#673AB7</color>
<color name="payment_sdk_button_text_color">#FFF</color>
<color name="payment_sdk_title_text_color">#FFF</color>
<color name="payment_sdk_button_background_color">#3F51B5</color>
<color name="payment_sdk_background_color">#F9FAFD</color>
<color name="payment_sdk_card_background_color">#F9FAFD</color>
// to override dimens
<dimen name="payment_sdk_primary_font_size">17sp</dimen>
<dimen name="payment_sdk_secondary_font_size">15sp</dimen>
<dimen name="payment_sdk_separator_thickness">1dp</dimen>
<dimen name="payment_sdk_stroke_thickness">.5dp</dimen>
<dimen name="payment_sdk_input_corner_radius">8dp</dimen>
<dimen name="payment_sdk_button_corner_radius">8dp</dimen>
</resourse>
Enums #
Those enums will help you in customizing your configuration.
- Tokenise types
The default type is none
enum PaymentSdkTokeniseType {
NONE,
USER_OPTIONAL,
USER_MANDATORY,
MERCHANT_MANDATORY
}
configuration.tokeniseType = PaymentSdkTokeniseType.USER_OPTIONAL;
- Token formats
The default format is hex32
enum PaymentSdkTokenFormat {
Hex32Format,
NoneFormat,
AlphaNum20Format,
Digit22Format,
Digit16Format,
AlphaNum32Format
}
- Transaction Type
The default type is PaymentSdkTransactionType.SALE
enum PaymentSdkTransactionType {
SALE,
AUTH
}
configuration.tokenFormat = PaymentSdkTokenFormat.Hex32Format
Demo application #
Check our complete example here https://github.com/paytabscom/flutter-sdk-bridge/tree/master/example.
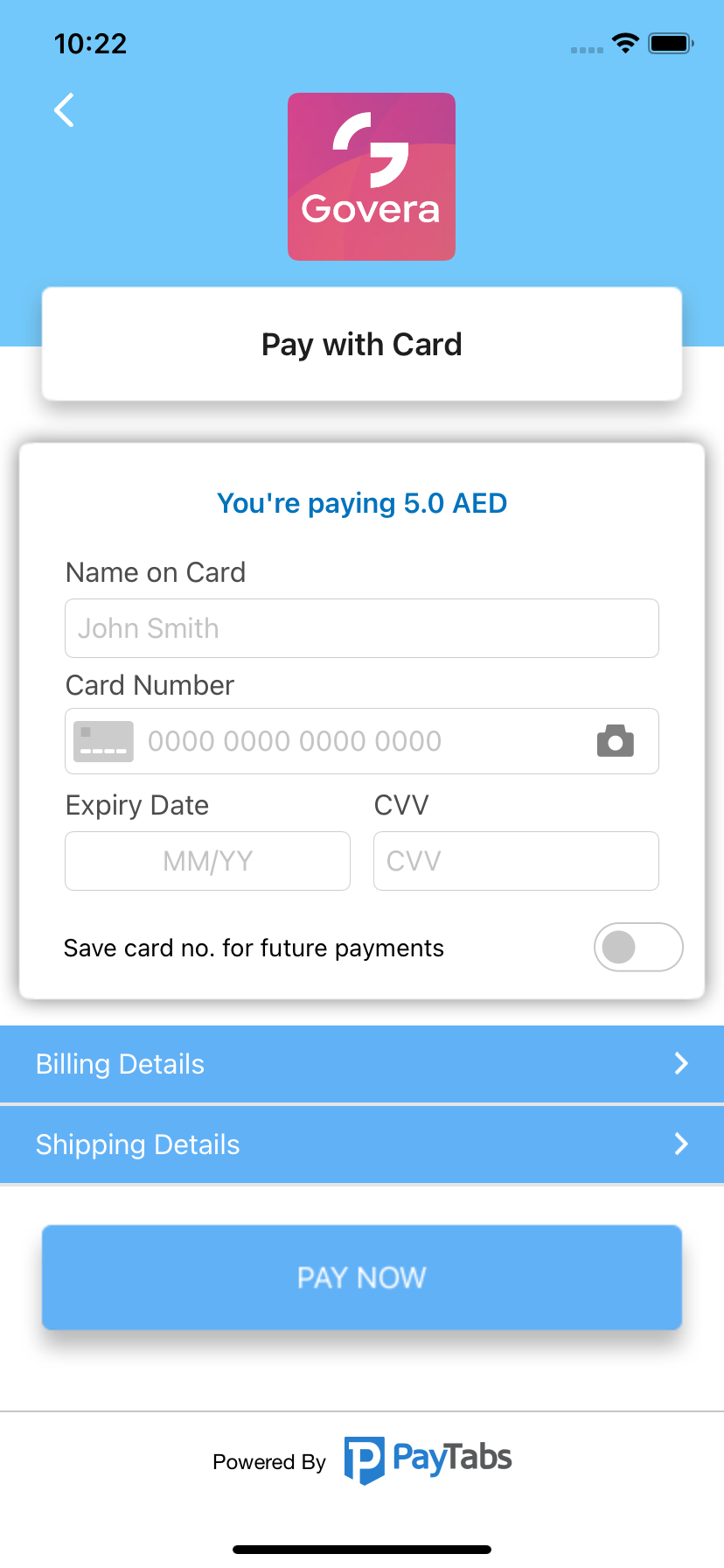
License #
See LICENSE.