flutter_painting_tools 1.1.0+1
flutter_painting_tools: ^1.1.0+1 copied to clipboard
A simple flutter library that allows the user to paint on the screen.
Flutter Painting Tools #
A simple flutter library that allows the user to paint on the screen.
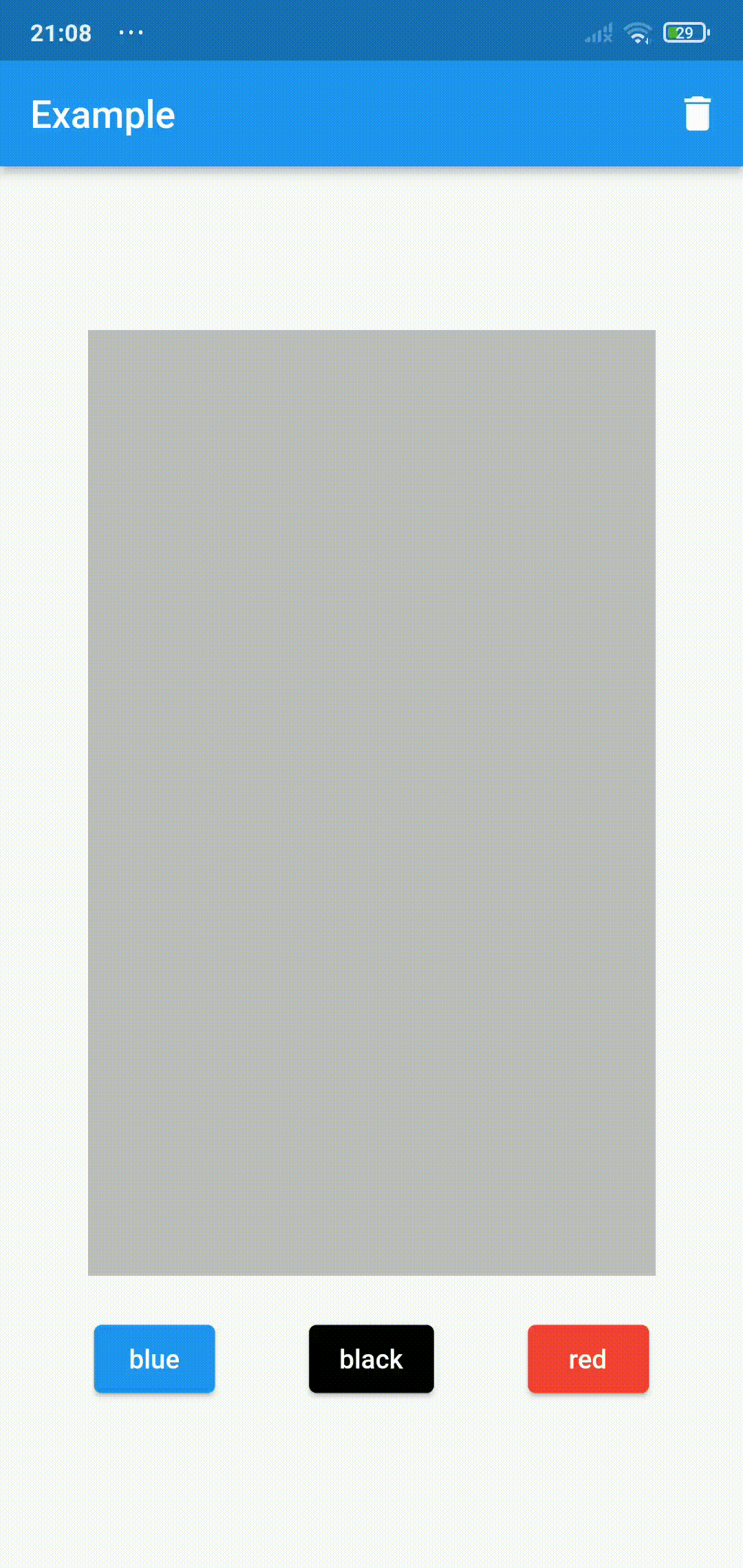
Getting started #
Basic Usage
This package is very easy to use.
For a basic usage you can just show a simple board on the screen where the user can paint, by displaying a PaintingBoard
in your code.
For example:
Center(
child: PaintingBoard(
boardHeight: 500,
boardWidth: 300,
),
)
Which will produce an output like this:
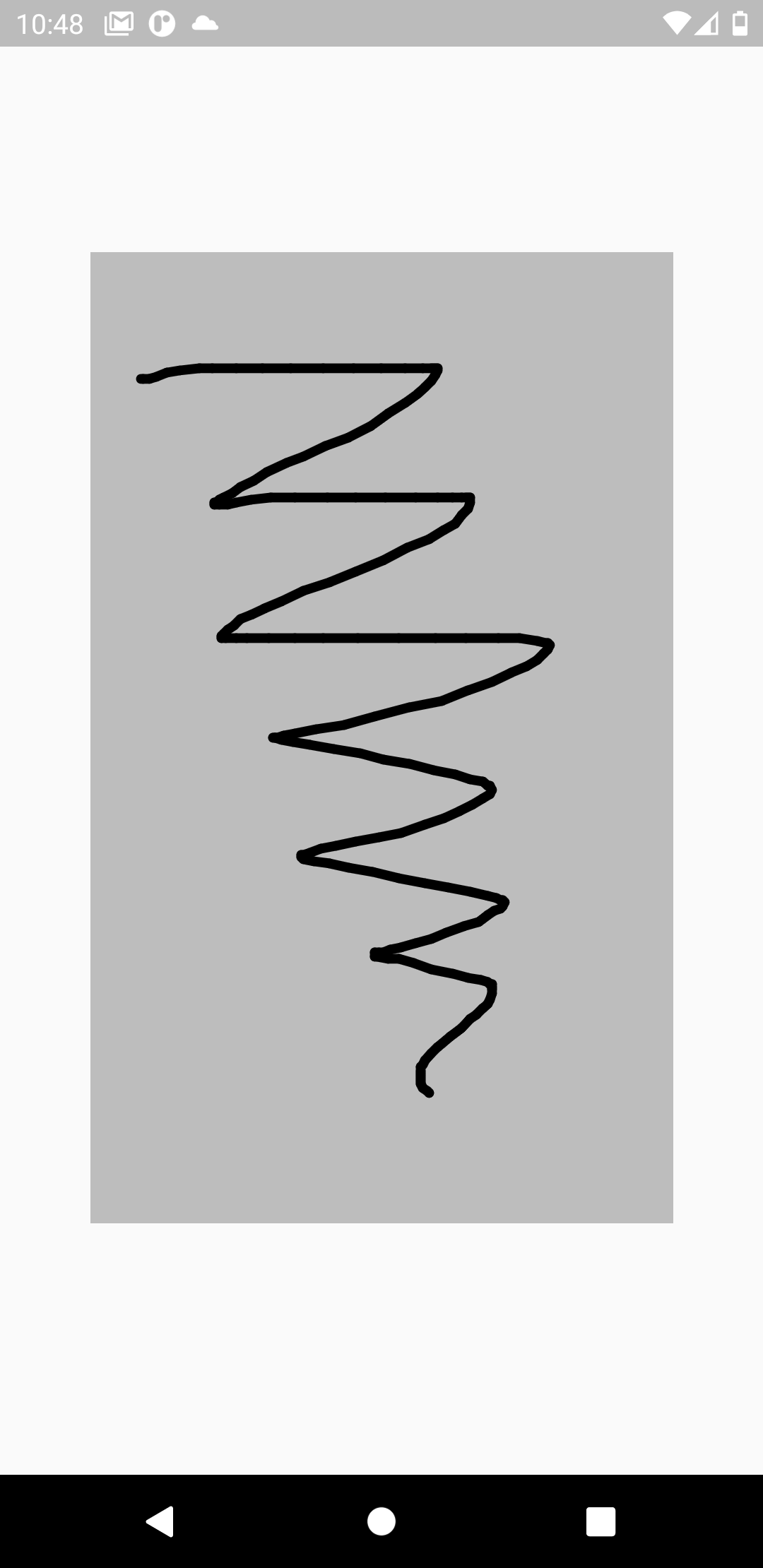
You can customize your PaintingBoard
how much do you want by changing its parameters
Parameter | Type | Meaning | Default Value |
---|---|---|---|
boardHeight |
double |
The height of the PaintingBoard |
double.infinity |
boardWidth |
double |
The width of the PaintingBoard |
double.infinity |
boardbackgroundColor |
Color? |
The background color of the PaintingBoard |
Colors.grey |
boardDecoration |
BoxDecoration? |
The decoration of the PaintingBoard |
null |
controller |
PaintingBoardController? |
The controller used to manage advanced task of the PaintingBoard (explained further) |
null |
Advanced Usage
Setting up the controller
If you want do perform advanced task, such as paint with different colors, delete the painting, ecc. you need to set up a PaintingBoardController
.
Follow these steps to do it properly:
-
Declare the
PaintingBoardController
inside the state of yourStatefulWidget
late final PaintingBoardController controller;
-
Allocate the
PaintingBoardController
inside theinitState()
method of yourStatefulWidget
@override void initState() { controller = PaintingBoardController(); super.initState(); }
-
Remember to dispose the
PaintingBoardController
inside thedispose()
method of yourStatefulWidget
@override void dispose() { controller.dispose(); super.dispose(); }
-
Pass the
PaintingBoardController
to thecontroller
property of thePaintingBoard
PaintingBoard( controller: controller, )
Paint with multiple colors
The default color of the "brush" used to paint inside the board is Colors.black
. If you want to paint with different colors you can use the changeBrushColor()
method of the PaintingBoardController
and pass to it the color you want.
Suppose for example you want to change the color of the "brush" when a button is tapped. You may do something like this:
ElevatedButton(
onPressed: () {
controller.changeBrushColor(Colors.blue);
}
)
Delete the painting
If you want to delete everything inside your PaintingBoard
you can simply call the deletePainting()
method of the PaintingBoardController
.
Example:
IconButton(
onPressed: () => controller.deletePainting(),
icon: const Icon(Icons.delete),
)
Delete last line painted
It is very simple to delete the last line painted. You just need to call the deleteLastLine()
method of the PaintingBoardController
.
Example:
IconButton(
onPressed: () => controller.deleteLastLine(),
icon: const Icon(Icons.delete),
)