flutter_mask_view 1.0.2
flutter_mask_view: ^1.0.2 copied to clipboard
A Flutter plugin to show mask, you can create height-light mask view or image progress view with it.
create mask view for your application, like image progress bar, or guide view for newer
Features #
- show height-light mask for newer
- create image progress bar
Usage #
Import the packages: #
import 'package:flutter_mask_view/flutter_mask_view.dart';
copied to clipboard
show height-light mask for newer: #
Scaffold(
body: Stack(
children: [
//only display background for demo
Image.asset(ImagesRes.BG_HOME),
//config
HeightLightMaskView(
//set view size
maskViewSize: Size(720, 1080),
//set barrierColor
backgroundColor: Colors.blue.withOpacity(0.6),
//set color for height-light
color: Colors.transparent,
//set height-light shape
// if width == radius, circle or rect
rRect: RRect.fromRectAndRadius(
Rect.fromLTWH(100, 100, 50, 50),
Radius.circular(50),
),
)
],
),
)
copied to clipboard
more:
HeightLightMaskView(
maskViewSize: Size(720, 1080),
backgroundColor: Colors.blue.withOpacity(0.6),
color: Colors.transparent,
//custom height-light shape
pathBuilder: (Size size) {
return Path()
..moveTo(100, 100)
..lineTo(50, 150)
..lineTo(150, 150);
},
//draw something above height-light view
drawAfter: (Canvas canvas, Size size) {
Paint paint = Paint()
..color = Colors.red
..strokeWidth = 15
..style = PaintingStyle.stroke;
canvas.drawCircle(Offset(150, 150), 50, paint);
},
//should repaint, default 'return false'
rePaintDelegate: (CustomPainter oldDelegate){
return false;
},
)
copied to clipboard
Display
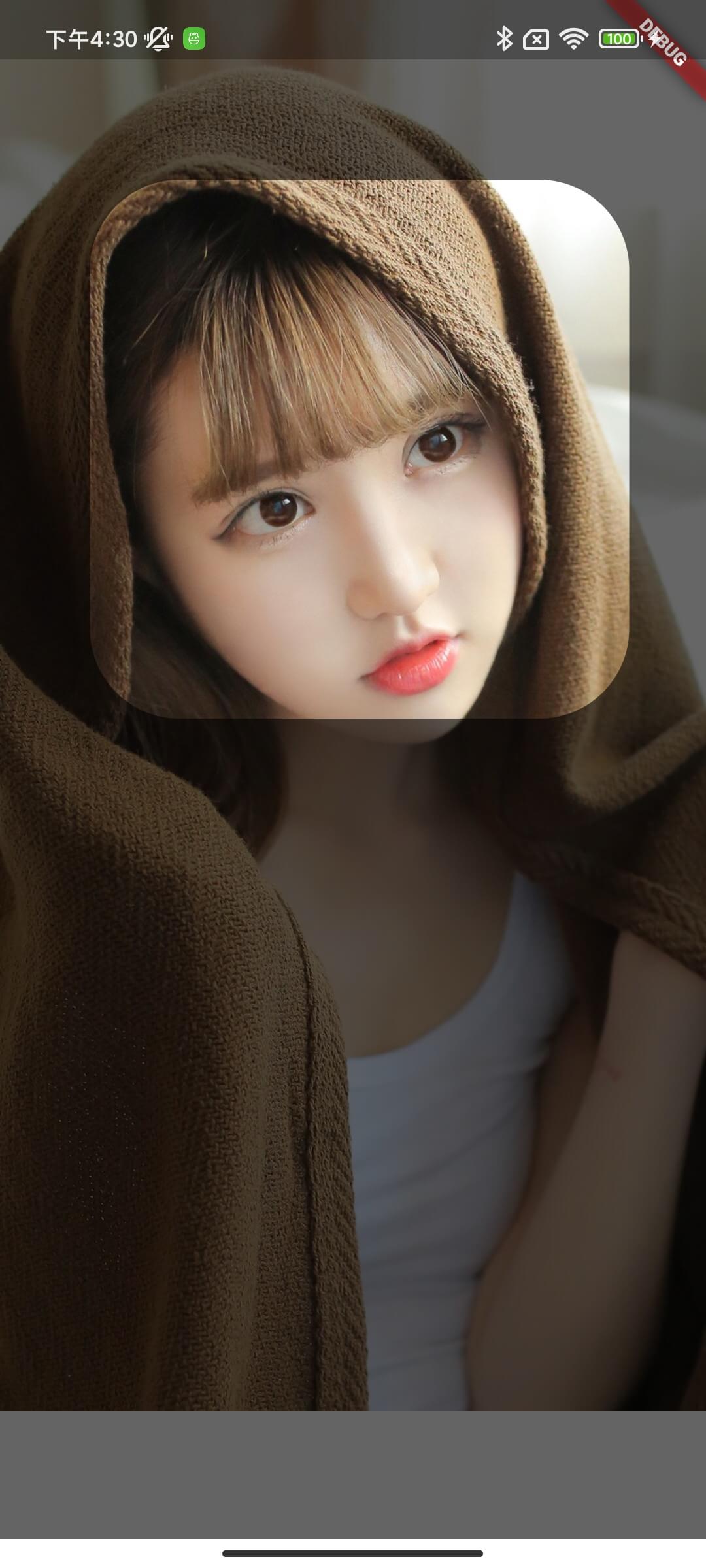
create image progress bar: #
ImageProgressMaskView(
size: Size(360, 840),
//background image
backgroundRes: 'images/bg.png',
//current progress
progress: 0.5,
//mask shape, built-in:
//PathProviders.sRecPathProvider: wave progress bar
//PathProviders.createWaveProvider: rect clip progressbar
//you can create more shape
pathProvider: PathProviders.createWaveProvider(60, 100),
),
)
copied to clipboard
PathProviders.sRecPathProvider
:
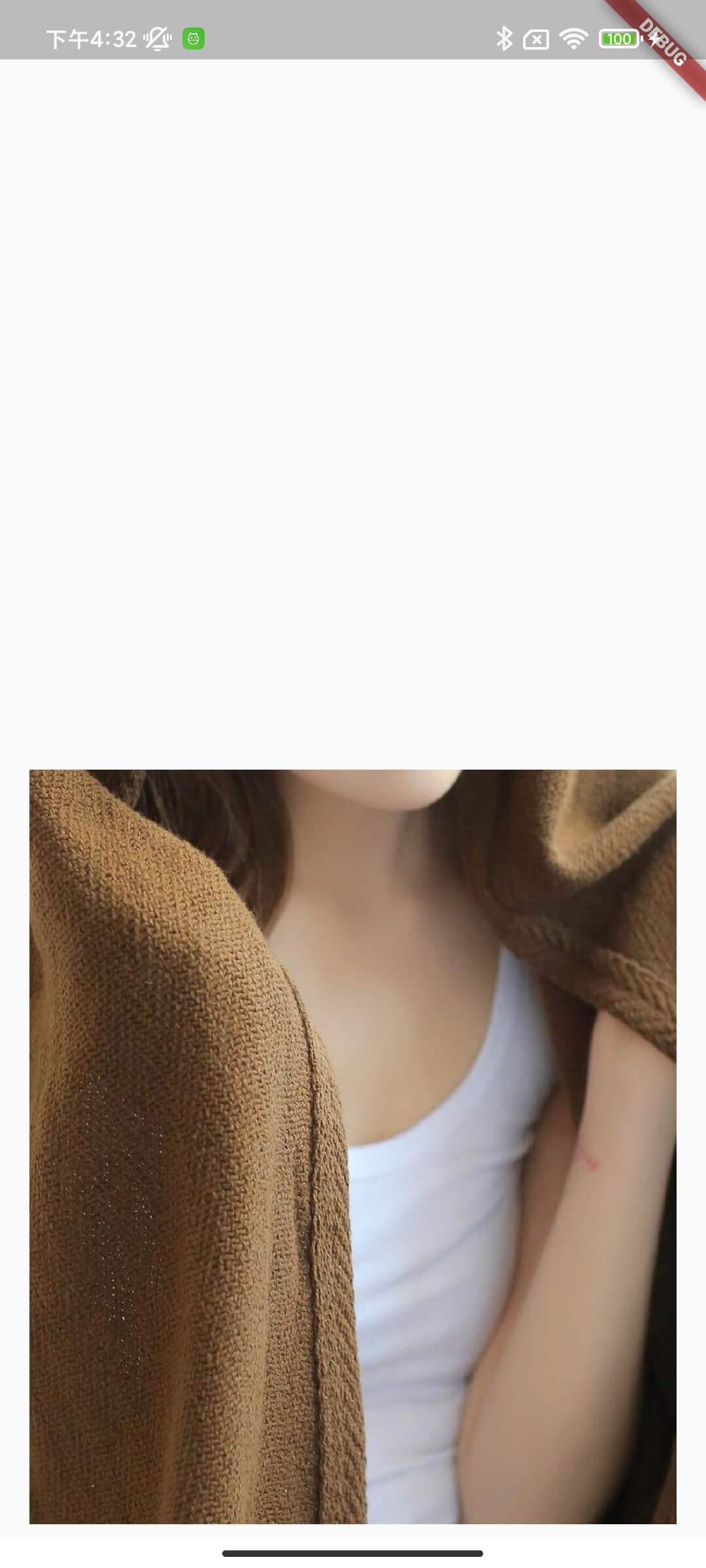
PathProviders.createWaveProvider
:
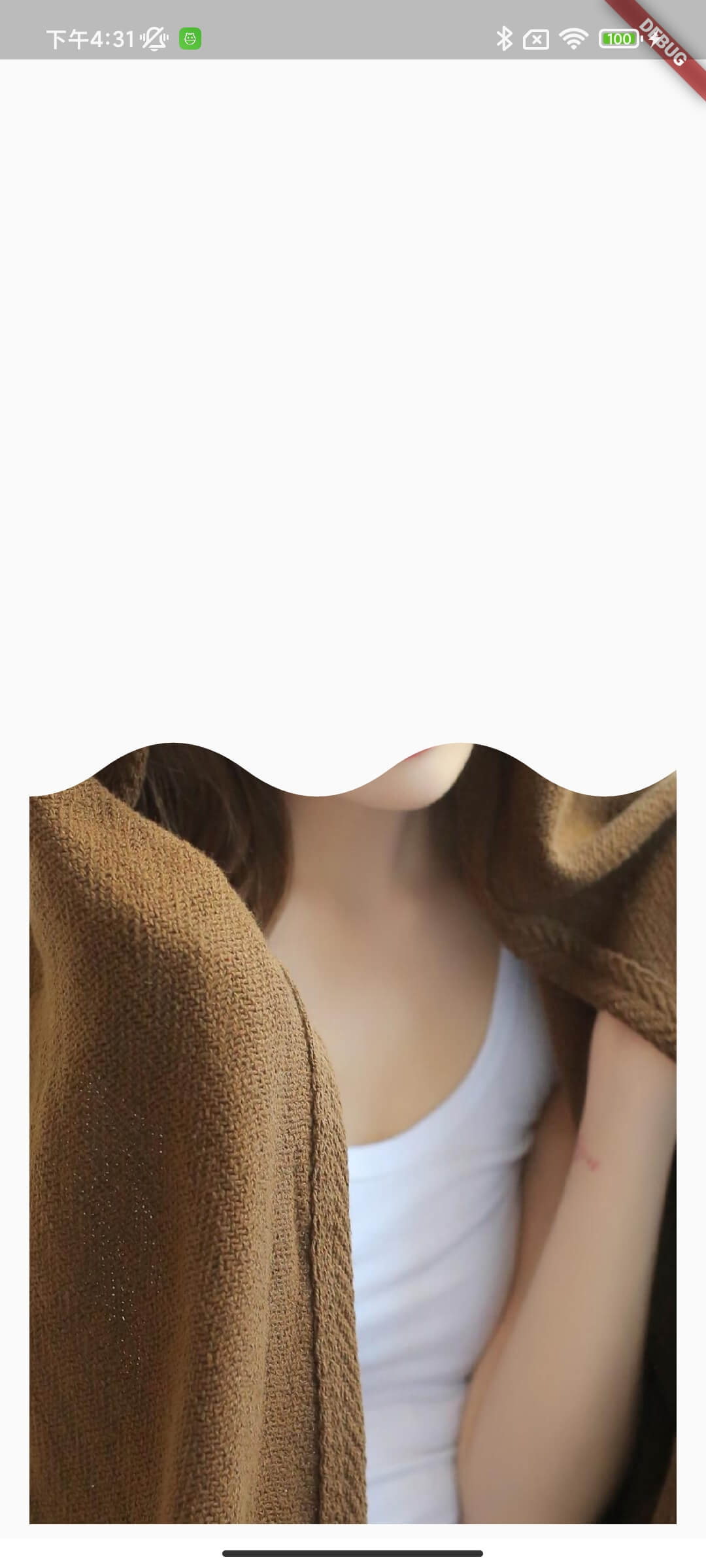
for animation:
class _MaskTestAppState extends State<MaskTestApp>
with SingleTickerProviderStateMixin {
late AnimationController _controller;
@override
void initState() {
_controller =
AnimationController(duration: Duration(seconds: 5), vsync: this);
_controller.forward();
super.initState();
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.black,
body: Center(
child: AnimatedBuilder(
animation: _controller,
builder: (context, child) {
return Stack(
alignment: Alignment.center,
children: [
ImageProgressMaskView(
size: Size(300, 300),
backgroundRes: ImagesRes.IMG,
progress: _controller.value,
pathProvider: PathProviders.createWaveProvider(60, 40),
rePaintDelegate: (_) => true,
),
Text(
'${(_controller.value * 100).toInt()} %',
style: TextStyle(
color: Colors.red,
fontWeight: FontWeight.bold,
fontSize: 30,
),
)
],
);
},
),
),
);
}
}
copied to clipboard
Result:
case 1:
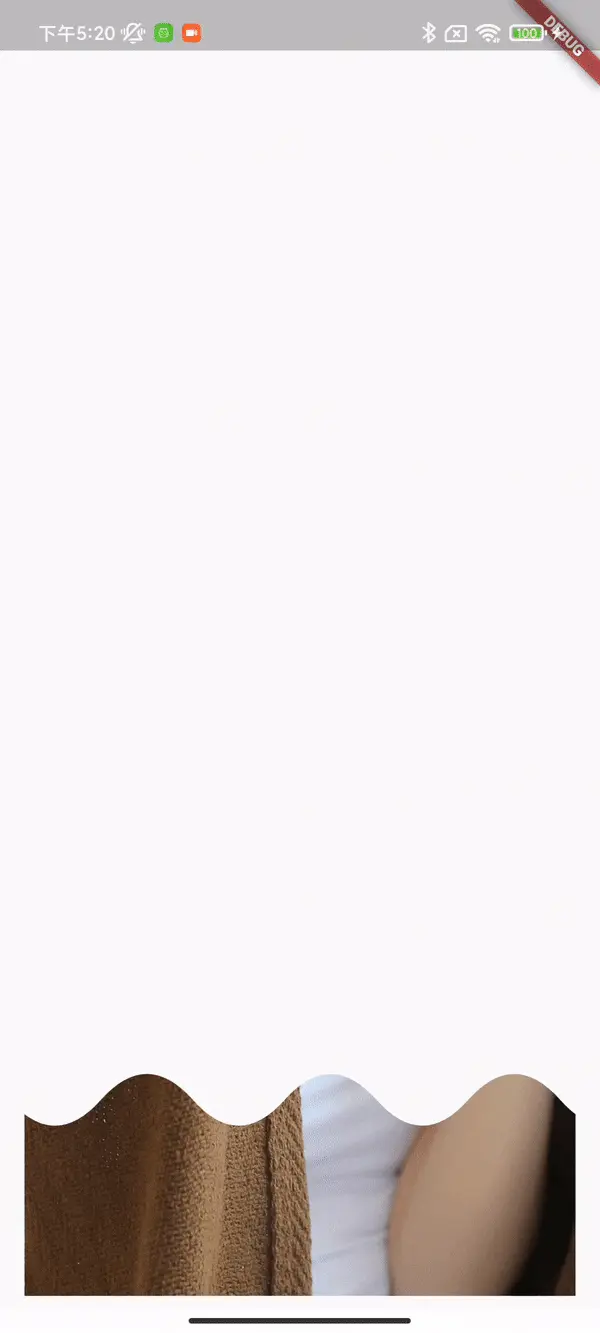
case 2: (png)
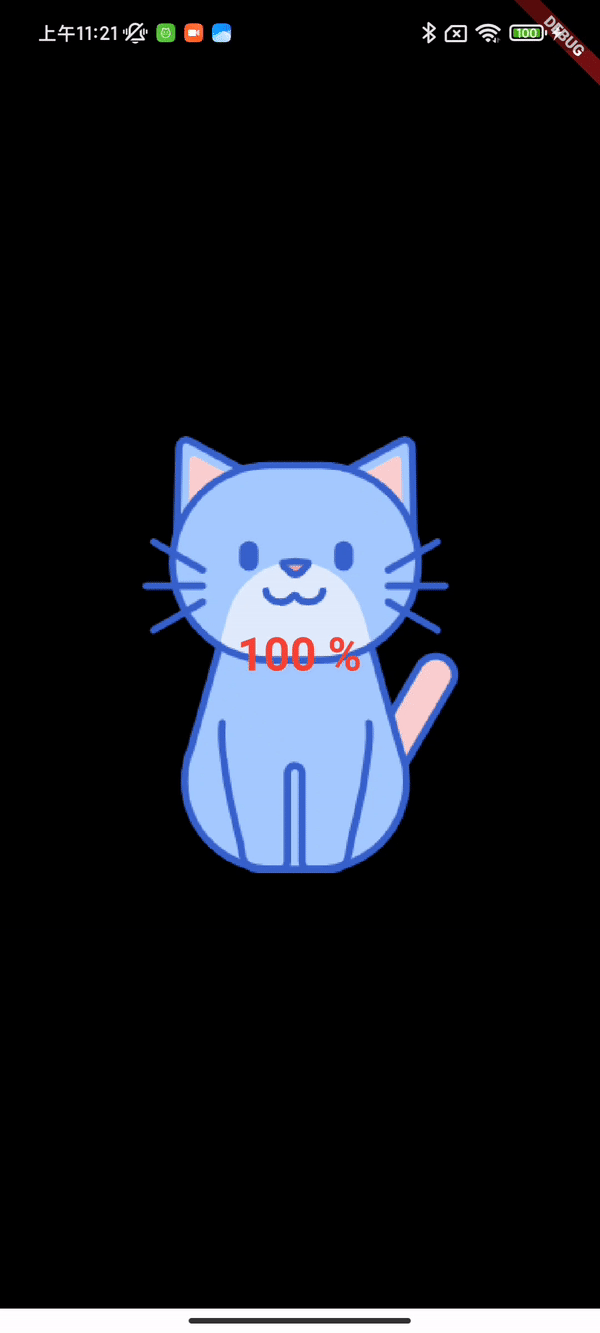