flutter_hooks_test 0.0.7+1
flutter_hooks_test: ^0.0.7+1 copied to clipboard
Simple and complete Flutter hooks testing utilities that encourage good testing practices..
Flutter Hooks Testing Library
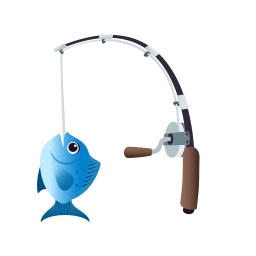
Simple and complete Flutter hooks testing utilities that encourage good testing practices.
Inspired by react-hooks-testing-library.
The first thing #
This package has great respect for react-hooks-testing-library. So the idea is based on it.
The problem #
You're writing an awesome custom hook and you want to test it, but as soon as you call it you see the following error:
Invariant Violation: Hooks can only be called inside the body of a function component.
You don't really want to write a component solely for testing this hook and have to work out how you were going to trigger all the various ways the hook can be updated, especially given the complexities of how you've wired the whole thing together.
The solution #
The Flutter Hooks Testing Library
allows you to create a simple test harness for Flutter hooks that
handles running them within the body of a function component, as well as providing various useful
utility functions for updating the inputs and retrieving the outputs of your amazing custom hook.
This library aims to provide a testing experience as close as possible to natively using your hook
from within a real component.
Using this library, you do not have to concern yourself with how to construct, render or interact with the flutter component in order to test your hook. You can just use the hook directly and assert the results.
When to use this library #
- You're writing a library with one or more custom hooks that are not directly tied to a component
- You have a complex hook that is difficult to test through component interactions
When not to use this library #
- Your hook is defined alongside a component and is only used there
- Your hook is easy to test by just testing the components using it
Installation #
dev_dependencies:
flutter_hooks_test: ^x.x.x
Example #
use_update.dart
#
VoidCallback useUpdate() {
final attempt = useState(0);
return () => attempt.value++;
}
use_update_test.dart
#
Not using
testWidgets('should re-build component each time returned function is called', (tester) async {
// Before
const key = Key('button');
var buildCount = 0;
// called count is 1
await tester.pumpWidget(HookBuilder(builder: (context) {
final update = useUpdate();
buildCount++;
return GestureDetector(
key: key,
onTap: () => update(),
);
}));
// called count is 2
await tester.tap(find.byKey(key));
await tester.pumpAndSettle(const Duration(milliseconds: 1));
expect(buildCount, 2);
});
Using
testWidgets('should re-build component each time returned function is called', (tester) async {
// After
var buildCount = 0;
final result = await buildHook(
(_) {
buildCount++;
return useUpdate();
},
wrapper: (child) => Container(child: child),
);
expect(buildCount, 1);
final update = result.current;
await act(() => update());
expect(buildCount, 2);
});
Issues #
Please file Flutter Hooks Testing Library specific issues, bugs, or feature requests in our issue tracker.
Plugin issues that are not specific to Flutter Hooks Testing Library can be filed in the Flutter issue tracker.
Contributing #
If you wish to contribute a change to any of the existing plugins in this repo, please review our contribution guide and open a pull request.