fluent_sdk 0.2.2
fluent_sdk: ^0.2.2 copied to clipboard
Package that provide a way to modularize features through a service locator.
fluent_sdk #
Package that provide a way to modularize features through a service locator.
Getting Started #
Add dependencies #
fluent_sdk: ^0.2.2
Create a interface/implementation to access the feature functionalities #
// Interface
abstract class HomeApi {
Widget getHomePage();
}
// Implementation
class HomeApiImpl extends HomeApi {
@override
Widget getHomePage() {
return Scaffold(
appBar: AppBar(
title: const Text("Fluent SDK Demo"),
),
body: const Center(
child: Text("Hello from Fluent SDK"),
),
);
}
}
Create module #
class HomeModule extends Module {
@override
Future<void> build(Registry registry) async {
// Register home api to access globally to it
registry.registerSingleton<HomeApi>((it) => HomeApiImpl());
}
}
Build module #
void main() async {
await Fluent.build([
HomeModule(),
]);
runApp(const MainApp());
}
Use it #
class MainApp extends StatelessWidget {
const MainApp({super.key});
@override
Widget build(BuildContext context) {
// Access to home api and get home page
final homePage = Fluent.get<HomeApi>().getHomePage();
return MaterialApp(
home: Scaffold(
body: homePage,
),
);
}
}
Example #
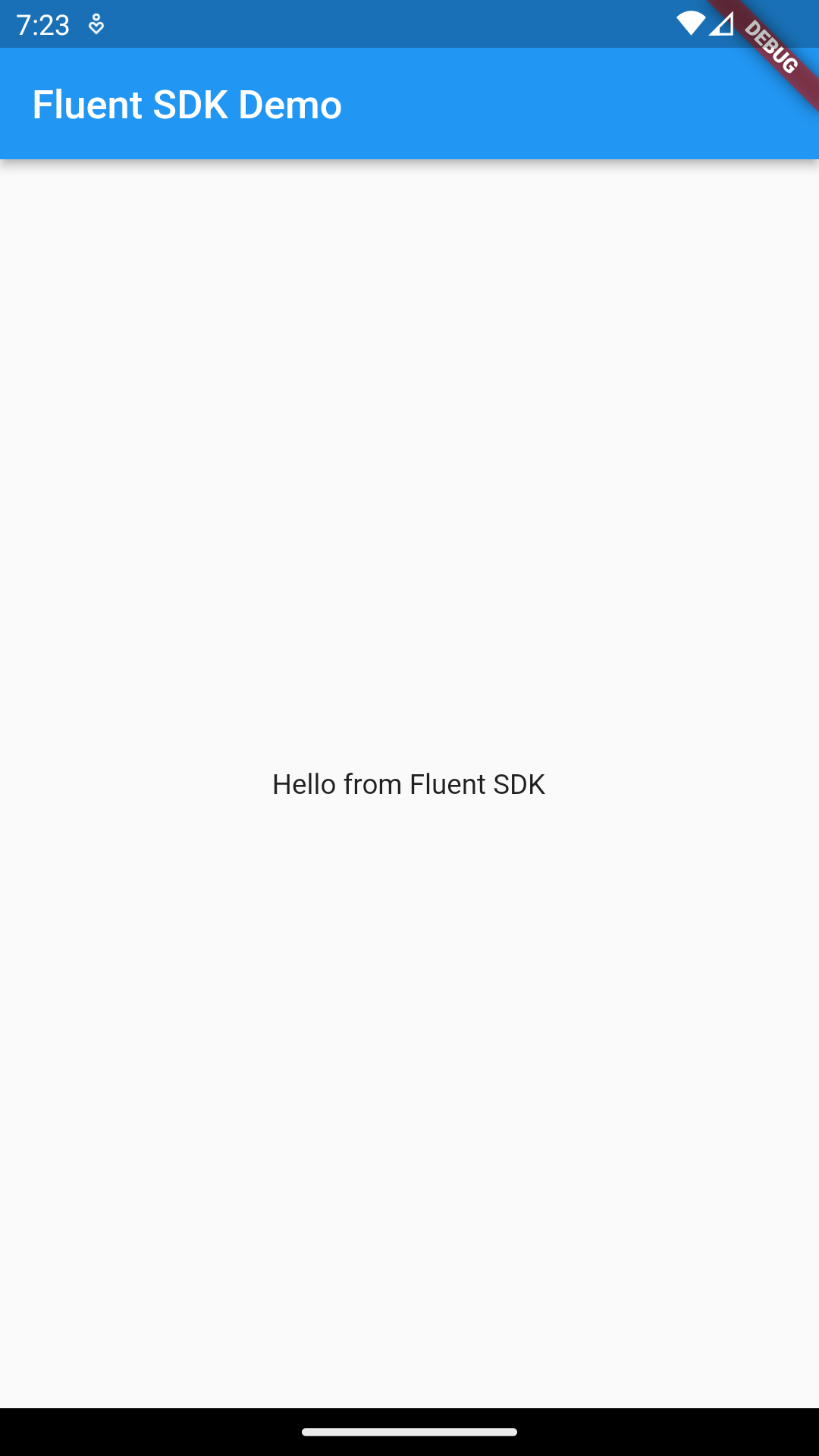