field_suggestion 0.1.4
field_suggestion: ^0.1.4 copied to clipboard
Create highly customizable, simple, and controllable autocomplete fields.
Field Suggestions
Installing #
Depend on it #
Add this to your package's pubspec.yaml
file:
dependencies:
field_suggestion: <latest_version>
Install it #
You can install packages from the command line:
$ flutter pub get
Import it #
Now in your Flutter code, you can use:
import 'package:field_suggestion/field_suggestion.dart';
Usage and overview #
Require to create a TextEditingController
and suggestions list. E.g:
final textEditingController = TextEditingController();
// And
List<String> suggestionList = [
'test@gmail.com',
'test1@gmail.com',
'test2@gmail.com',
];
// Or
List<int> numSuggestions = [
13187829696,
13102743803,
15412917703,
];
Basic usage. #
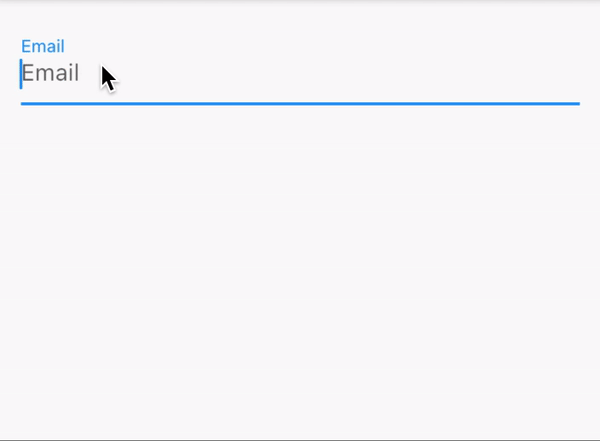
FieldSuggestion(
textController: textEditingController,
suggestionList: suggestionList,
hint: 'Email',
),
Custom usage. #
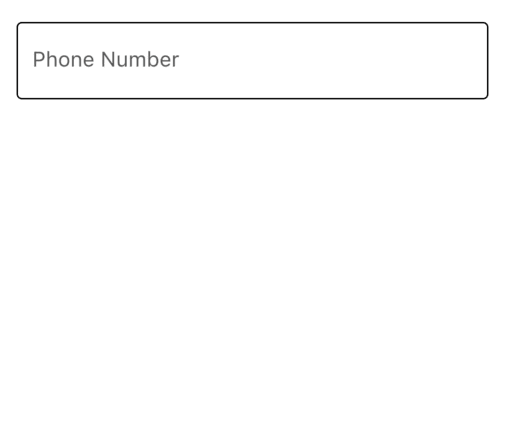
FieldSuggestion(
textController: textEditingController,
suggestionList: numSuggestions,
fieldDecoration: InputDecoration(
hintText: "Phone number",
enabledBorder: const OutlineInputBorder(),
focusedBorder: const OutlineInputBorder(),
),
wDivider: true,
divider: const SizedBox(height: 5),
wSlideAnimation: true,
slideAnimationStyle: SlideAnimationStyle.LTR,
slideCurve: Curves.linearToEaseOut,
animationDuration: const Duration(milliseconds: 300),
suggestionItemStyle: SuggestionItemStyle.WhiteNeumorphismedStyle,
suggestionBoxStyle: SuggestionBoxStyle(
backgroundColor: Colors.white,
borderRadius: BorderRadius.circular(15),
boxShadow: [
BoxShadow(
color: Colors.blue.withOpacity(.2),
spreadRadius: 5,
blurRadius: 10,
offset: const Offset(0, 5),
),
],
),
)
External control #
Here we just wrapped our Scaffold
with GestureDetector
to handle gestures on the screen.
And now we can close box when we tap on the screen. (You can do it everywhere, where you used FieldSuggestion
with BoxController
).
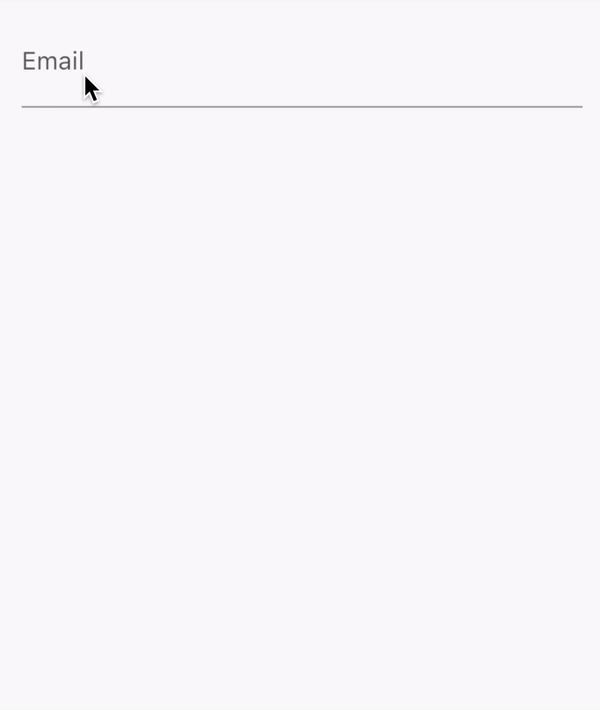
class Example extends StatelessWidget {
final _textController = TextEditingController();
final _boxController = BoxController();
@override
Widget build(BuildContext context) {
return GestureDetector(
onTap: () => _boxController.close(),
child: Scaffold(
body: Center(
child: FieldSuggestion(
hint: 'test',
suggestionList: [], // Your suggestions list here...
boxController: _boxController,
textController: _textController,
),
),
),
);
}
}
Contributions #
If you find a bug or want a feature, but don't know how to fix/implement it, please fill an issue.
If you fixed a bug or implemented a new feature, please send a pull request.