easy_model 1.0.1
easy_model: ^1.0.1 copied to clipboard
A simple flutter state management package, can use global and local management
Language:简体中文
💼 easy_model #
A simple flutter state management package, supporting local state management and global state management
- support
initState()
anddispose()
- Support global access to
Model
objects
🚀 Getting Started #
Before the introduction, you can have a try for Online Demo
🔑 Useage #
First define your Model
object
class YourModel extends Model {
@override
void initState() {...}
@override
void dispose() {...}
int value = 0;
}
When you want to use it in combination with a Widget or Page, you can do like this
ModelWidget<YourModel>(
childBuilder: (ctx, model) => YourWidgetOrPage(),
modelBuilder: () => YourModel(),
),
🔄 Get data and refresh #
get data
final model = ModelGroup.findModel<YourModel>();
refresh
model.refresh();
📃 Same pages and models #
If you have the following usage scenarios
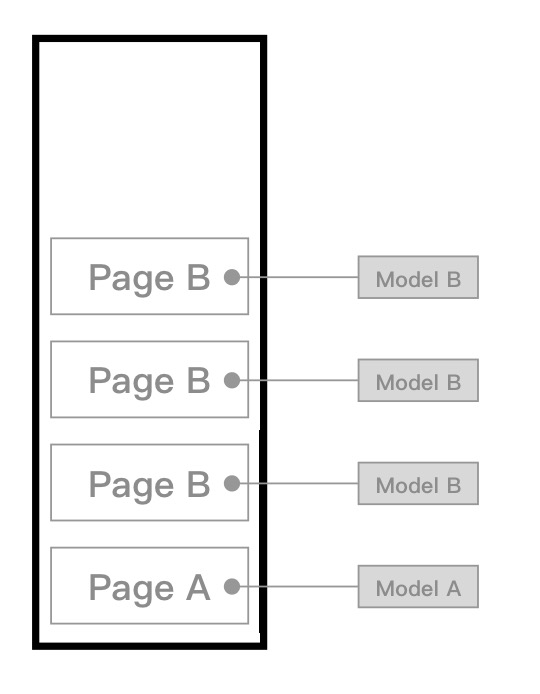
Using ModelGroup.findModel <T> ()
is unable to get the Model
object corresponding to each Page
Then you can use ModelGroup.findModelByKey <T> (modelKey)
to obtain the corresponding Model
, but it should be noted that a different modelKey
must be specified when creating ModelWidget
ModelWidget<YourModel>(
childBuilder: (ctx, model) => YourWidgetOrPage(),
modelBuilder: () => YourModel(),
modelKey: 'YourModelKey'
),
///get model
final model = ModelGroup.findModelByKey<YourModel>('YourModelKey');
🦋 Partial refresh #
With the new ModelWidget
and the new Model
, you can achieve the effect of partial refresh, but if you think that Model
is created too frequently, then you can use PartModelWidget
to achieve partial refresh
ModelWidget<YourModel>(
childBuilder: (ctx, model) => YourWidgetOrPage(),
modelBuilder: () => YourModel(),
modelKey: 'YourModelKey'
)
class YourWidgetOrPage extends StatelessWidget{
@override
Widget build(BuildContext context) {
return Column(
children: <Widget>[
ChildOne(),
ChildTwo(),
PartModelWidget<YourModel>(
childBuilder: (ctx,YourModel model) => ChildThree(),
partKey: 'YourKey',
),
],
);
}
}
In the above example, if you want to refresh ChildThree()
separately, you can achieve it by calling the following method
model.refreshPart('YourKey')
This avoids frequent creation of Model
. If multiple PartModelWidget
correspond to the same partKey
, they can be refreshed together every time they are refreshed!
🤗 Welcome for issue and pr #
Click here to create an issue
Click here to submit a pull request (please submit pr by forking the dev
branch)