dbx 0.0.2+1
dbx: ^0.0.2+1 copied to clipboard
A Data Storage Solution, created with simplicity in mind. It is a simple, lightweight, and fast database solution for Flutter apps.
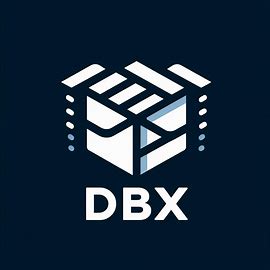
DBX #
dbx
is a Dart package designed for secure and efficient local storage using Protocol Buffers instead of JSON.
- A Key-Value Database with Easy API that supports wide range of Data Types.
Features #
- Protocol Buffers: Utilizes Protocol Buffers (protobuf) for data serialization, offering significant performance improvements over JSON.
- Secure Storage: Data is securely stored using
flutter_secure_storage
and encrypted with theencrypt
library. - Comprehensive Data Support: Supports all data types, including their list variants.
-
What we mean
- Supported data types are
int
,double
,bool
,String
,Uint8List
,List<int>
,List<double>
,List<bool>
,List<String>
, andList<Uint8List>
. Uint8List
for bytes
- Supported data types are
-
- Efficient and Compact: Protobuf is more efficient and compact compared to JSON, reducing latency and improving app performance.
- Familiar Api: Same API as of
SharedPreference
.- Won't miss
SharedPreference
. No headache, easy adaption.🚀
- Won't miss
- Created With Simplicity in mind
- No Object Creation, no overhead of state management solution
- No Object Passing
- All method are static, just call and use.
Benefits of Using Protocol Buffers Over JSON #
- Performance: Protobuf is significantly faster than JSON for serialization and deserialization.
- Compactness: Protobuf messages are smaller in size, which reduces storage space and improves transmission speed.
- Efficiency: Protobuf is more efficient in terms of CPU and memory usage.
Advantages Over Shared Preferences #
- Data Integrity: Protobuf ensures data integrity and consistency, which is crucial for complex data structures.
- Security: Data is encrypted and securely stored, unlike shared preferences which can be more vulnerable to security breaches.
- Scalability: Protobuf's efficient serialization makes it suitable for larger datasets and more complex data structures.
Installation #
Add dbx
to your pubspec.yaml
:
dependencies:
dbx: ^0.0.1
copied to clipboard
you can install packages from the command line:
with Flutter
:
$ flutter pub get
copied to clipboard
with Dart
:
$ dart pub get
copied to clipboard
Initialization #
with Flutter
:
import 'package:dbx/dbx.dart';
// Example usage
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await DBX.init();
runApp(MyApp());
}
copied to clipboard
with dart
:
import 'package:dbx/dbx.dart';
// Example usage
void main() async {
await DBX.init();
}
copied to clipboard
Usage #
- Store Data
// Store data
DBX.setString('message', 'Give it a try!');
DBX.setString('username', 'john_doe');
DBX.setInt('age', 30);
DBX.setDouble('height', 5.9);
DBX.setBool('isAdmin', true);
DBX.setBytes(
'profilePicture', File('assets/profile.jpeg').readAsBytesSync());
DBX.setStringList('tags', ['dart', 'flutter']);
DBX.setIntList('scores', [100, 95, 85]);
DBX.setDoubleList('strike_rate', [36.6, 37.0, 36.8]);
DBX.setBoolList('attendence', [true, false, true]);
DBX.setBytesList('files', [
File('assets/file1.jpeg').readAsBytesSync(),
File('assets/logo.jpeg').readAsBytesSync(),
File('assets/file2.jpeg').readAsBytesSync()
]);
copied to clipboard
- Read Data
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
appBar: AppBar(
title: Text('DBX Example'),
centerTitle: true,
),
body: Center(
child: FutureBuilder(
future: writeData(),
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.done) {
final files =DBX.getBytesList('files');
return Column(
children: [
Image.memory(Uint8List.fromList(
DBX.getBytes('profilePicture') ?? [])),
Text("Message: ${DBX.getString('message') ?? 'No data'}"),
Text(
"Username: ${DBX.getString('username') ?? 'No Data'}"), // Output: john_doe
Text("Age: ${DBX.getInt('age')}"), // Output: 30
Text(
"Height: ${DBX.getDouble('height')}"), // Output: 5.9
Text(
"IsAdmin: ${DBX.getBool('isAdmin')}"), // Output: true
Text(
"Tags: ${DBX.getStringList('tags') ?? [].join(',')}"), // Output: [dart, flutter]
Text(
"Scores: ${DBX.getIntList('scores') ?? [].join(',')}"), // Output: [100, 95, 85]
Text(
"Strike Rate: ${DBX.getDoubleList('strike_rate')}"), // Output: [36.6, 37.0, 36.8]
Text(
"Attendence: ${DBX.getBoolList('attendence')}"), // Output: [true, false, true]
if (files != null)
Row(
mainAxisSize: MainAxisSize.min,
children: [
for (final file in files)
Image.memory(Uint8List.fromList(file)),
],
),
],
);
} else {
return CircularProgressIndicator();
}
},
),
),
),
);
}
copied to clipboard
Contributing #
We welcome contributions from the community! If you have any ideas, suggestions, or bug reports, please open an issue or submit a pull request.
Sponsor #
If you find this package useful, please consider sponsoring its development to help us continue improving it. You will be mentioned below
License #
This project is licensed under the MIT License - see the LICENSE file for details.
TODO #
- Support for Dart Objects: Adding support for storing Dart objects is still pending and requires contributions from the community. If you are interested in helping out, please check out the issues section or submit a pull request.